JSF Internationalisation i18n – Message bundles
In this tutorial we will show you how to support multiple languages using JSF Internationalisation.
JSF internationalisation
Project Structure
src
|--main
| +--java
| +--com
| +--memorynotfound
| |--LanguageSwitcher.java
| +--resources
| +--com
| +--memorynotfound
| +--i18n
| |--messages.properties
| |--messages_en.properties
| |--messages_nl.properties
| +--webapp
| +--resources
| +--img
| +--icon
| |--be.png
| |--us.png
| +--WEB-INF
| |--faces-config.xml
| |--web.xml
| +--index.xhtml
pom.xml
Properties files
messages_en.properties
funny.slogan = Behind every great women, there is a man... looking at her ass
messages_nl.properties
funny.slogan = Achter elke sucesvolle vrouw, staat en man... kijkend naar haar kont
Faces-Config.xml
<faces-config xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-facesconfig_2_2.xsd" version="2.2">
<application>
<locale-config>
<default-locale>en</default-locale>
<supported-locale>en</supported-locale>
<supported-locale>nl</supported-locale>
</locale-config>
<resource-bundle>
<base-name>com.memorynotfound.i18n.messages</base-name>
<var>msg</var>
</resource-bundle>
</application>
</faces-config>
Managed Bean
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import javax.faces.context.FacesContext;
import java.util.Locale;
@ManagedBean
@RequestScoped
public class LanguageSwitcher {
private Locale locale = FacesContext.getCurrentInstance().getViewRoot().getLocale();
public Locale getLocale() {
return locale;
}
public String getLanguage() {
return locale.getLanguage();
}
public void changeLanguage(String language) {
locale = new Locale(language);
FacesContext.getCurrentInstance().getViewRoot().setLocale(new Locale(language));
}
}
JSF View
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core">
<h:head>
<style type="text/css">
.flag {
display: block;
text-indent: 25px;
}
.be {
background: url('resources/img/icon/be.png') no-repeat 0;
}
.us {
background: url('resources/img/icon/us.png') no-repeat 0;
}
</style>
</h:head>
<h:body>
<h1>JSF 2.2 Internationalization Example</h1>
<h:form>
<h:commandLink styleClass="flag us" action="#{languageSwitcher.changeLanguage('us')}" value="English"/>
<h:commandLink styleClass="flag be" action="#{languageSwitcher.changeLanguage('nl')}" value="Dutch"/>
</h:form>
<p><h:outputText value="#{msg['funny.slogan']}"/></p>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-internationalisation/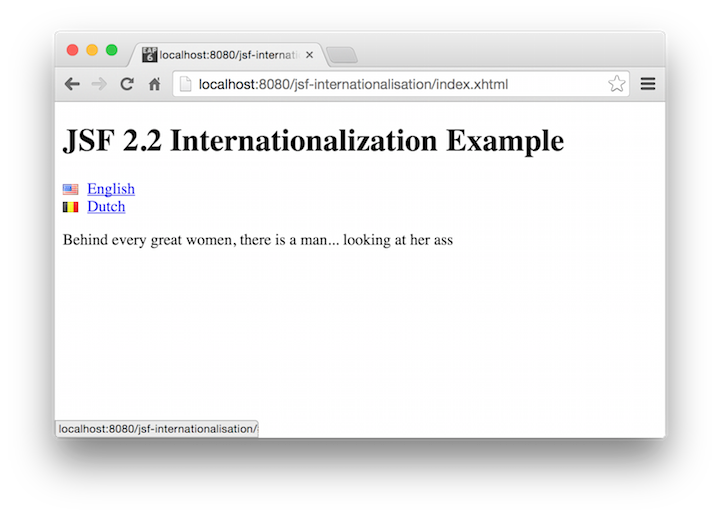
And when we click on the dutch link we get the dutch representation of the funny.slogan text
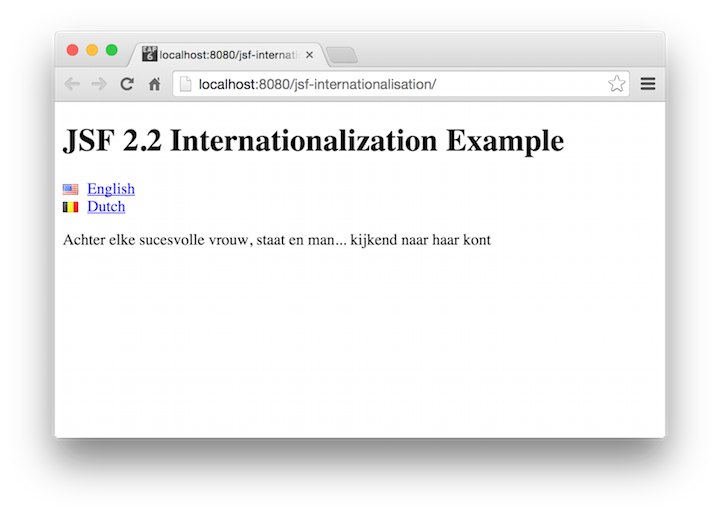
Download Source Code
Download it – JSF-2-2-Internationalization example