JAX-RS JSON Rest Service with Jersey Example
In this tutorial we will show you how you can create a JSON rest service with jersey that will respond with JSON output. This tutorial covers both jersey version 1 and 2.
Maven Dependencies
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.memorynotfound.webservice.rs.jersey1</groupId>
<artifactId>jersey-json</artifactId>
<version>1.0.0-SNAPSHOT</version>
<packaging>war</packaging>
<properties>
<jersey.version>1.18.3</jersey.version>
</properties>
<dependencies>
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-servlet</artifactId>
<version>${jersey.version}</version>
</dependency>
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-json</artifactId>
<version>${jersey.version}</version>
</dependency>
</dependencies>
</project>
Note: If you want to use jersey version 2 you need to replace the jersey-servlet dependency with the following jersey-container-servlet dependency. And the jersey-json dependency with jersey-media-json-jackson.
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet</artifactId>
<version>2.14</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-json-jackson</artifactId>
<version>2.14</version>
</dependency>
Model
This model will be serialized to JSON representation. Note that no annotations or default constructor is needed.
package com.memorynotfound.rs;
public class Notification {
private Integer id;
private String message;
public Notification(Integer id, String message) {
this.id = id;
this.message = message;
}
public Integer getId() {
return id;
}
public String getMessage() {
return message;
}
}
Rest Service
We create our Jersey JSON Rest service using Jackson. We will give a short explanation of what the annotations are for.
@Path
: maps the java class as a rest service to the specified path. The@Path
annotation can be used on your class and/or methods. When you use this on your class this works like a base and all the methods derive from it. In our example we map our class to notification. On our method fetchBy we use a path parameter.@GET
: maps the method to a HTTP GET request you can also use@POST
,@PUT
,@DELETE
.@Produces
: tells jersey which content type to serve. In our case we serve application/json@Consumes
: tells jersey which content type to accept. In our case we consume application/json
package com.memorynotfound.rs;
import javax.ws.rs.*;
import javax.ws.rs.core.MediaType;
import java.util.ArrayList;
import java.util.List;
@Path("/notifications")
public class NotificationRestService {
@GET
@Produces(MediaType.APPLICATION_JSON)
public List<Notification> fetchAll() {
// fetch all notifications
List<Notification> notifications = new ArrayList<Notification>();
notifications.add(new Notification(1, "New user created"));
notifications.add(new Notification(2, "New order created"));
return notifications;
}
@GET
@Path("{id: \\d+}")
@Produces(MediaType.APPLICATION_JSON)
public Notification fetchBy(@PathParam("id") int id) {
// fetch notification by id
return new Notification(id, "Rise and shine.");
}
@POST
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.APPLICATION_JSON)
public Notification create(Notification notification) {
// create notification
return notification;
}
@PUT
@Consumes(MediaType.APPLICATION_JSON)
public void update(Notification notification) {
// update notification
}
@DELETE
@Path("{id}")
public void delete(@PathParam("id") int id) {
// deleting notification
}
}
Web.xml
Now we need to map our rest service to the jersey servlet. In our example we map the servlet to /api/*.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<servlet>
<servlet-name>Jersey-Servlet</servlet-name>
<servlet-class>com.sun.jersey.spi.container.servlet.ServletContainer</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>Jersey-Servlet</servlet-name>
<url-pattern>/api/*</url-pattern>
</servlet-mapping>
</web-app>
Note: If you want to use jersey version 2 you need to replace the servlet definition with the following:
<servlet>
<servlet-name>Jersey-Servlet</servlet-name>
<servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>jersey.config.server.provider.packages</param-name>
<param-value>com.memorynotfound.rs</param-value>
</init-param>
</servlet>
Demo
URL: http://localhost:8080/jersey-json/api/notifications
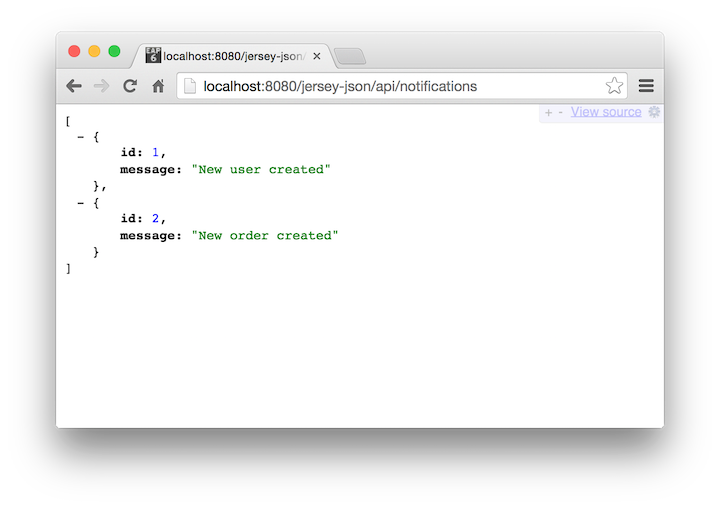
You have forgotten to mention servlet init-param for com.sun.jersey.spi.container.servlet.ServletContainer. it should be there as follows
in servlet configuration in web.xml
You should indeed use the servlet init parameters, but only if you are using Jersey 2. We noted this at the bottom.
Thanks for your response