Servlet Context Parameter Example Configuration
In this tutorial we will show how you can use the servlet context parameter to inject in a servlet. We can configure our servlet entirely with annotations, but as the context parameters are meant for all servlets we need to define them globally inside the web.xml servlet descriptor.
Project structure
+--src
| +--main
| +--java
| +--com
| +--memorynotfound
| |--ExampleServlet.java
| |--resources
| +--webapp
| +--WEB-INF
| |--web.xml
pom.xml
Maven Dependency
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
Servlet Context parameter definition
A servlet context parameter is intend for global servlet parameters there is no way to include them with an annotation. That is why we need to add the servlet context parameter in the web.xml file also known as the servlet descriptor.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<context-param>
<param-name>email</param-name>
<param-value>[email protected]</param-value>
</context-param>
<context-param>
<param-name>phone</param-name>
<param-value>xxxx/xx.xx.xx</param-value>
</context-param>
</web-app>
Servlet 3 Getting the Servlet Context Pameters
package com.memorynotfound;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet("/example")
public class ExampleServlet extends HttpServlet {
private String email, phone;
@Override
public void init(ServletConfig config) throws ServletException {
ServletContext ctx = config.getServletContext();
email = ctx.getInitParameter("email");
phone = ctx.getInitParameter("phone");
}
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
resp.setContentType("text/html");
PrintWriter out = resp.getWriter();
out.write("<html><body>");
out.write("<h2>Servlet Context Parameter example configuration</h2>");
out.write("<p><strong>E-mail: </strong>" + email + "</p>");
out.write("<p><strong>Phone: </strong>" + phone + "</p>");
out.write("</body></html>");
}
}
Demo
URL: localhost:8080/context-param/example
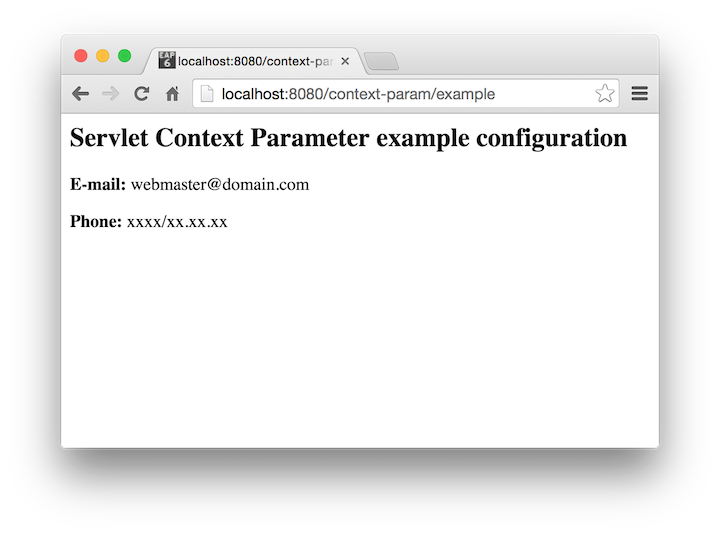