Servlet Redirect HTTP Request Example
In this example we will redirect an HTTP Request from one servlet to another servlet. The sendRedirect()
method of the HttpServletResponse
sends a temporary redirect response to the client. This redirect sets the status code to 302 – Temporary redirect. If the response already has been committed, this method throws an IllegalStateException
indicating that the response has already been written for that request.
Servlet Redirect HTTP Request
The sendRedirect()
method of the HttpServletResponse
sends a temporary redirect response to the client. This redirect sets the status code to 302 – Temporary redirect.
package com.memorynotfound;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet("/redirect")
public class RedirectServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
resp.sendRedirect(req.getContextPath() + "/landing");
}
}
Servlet Redirected HTTP Request
package com.memorynotfound;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet("/landing")
public class LandingServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
PrintWriter out = resp.getWriter();
out.write("Servlet redirect HTTP Request Example");
}
}
Demo
We enter the following URL: localhost:8080/servlet-redirect/redirect. This will redirect the request to the following URL.
URL: localhost:8080/servlet-redirect/landing
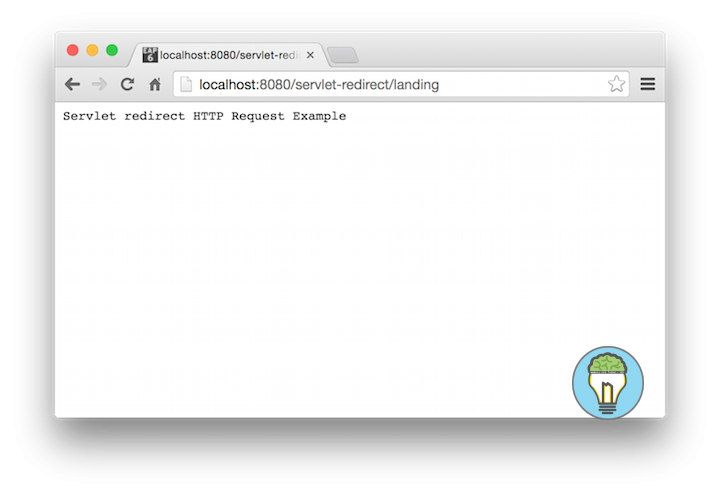