Taking a Selenium ScreenShot with JUnit in Java
In this example we will show you how you can take a selenium screenshot whenever you desire. Selenium has a built in ScreenShot function. This allows the developer to take screenshots of the selenium tests. This is especially cool when there are test failures, It provides more information when debugging why the tests failed. With a little tweak we can also take screenshots of specific WebDriver Element.
Maven Dependencies
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
</dependency>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>2.44.0</version>
</dependency>
Taking Selenium ScreenShots
package com.memorynotfound.test;
import org.apache.commons.io.FileUtils;
import org.junit.AfterClass;
import org.junit.BeforeClass;
import org.junit.Test;
import org.openqa.selenium.*;
import org.openqa.selenium.Point;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.remote.Augmenter;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.UUID;
public class ScreenShotTest {
private static final String FOLDER = "/tmp//";
private static WebDriver driver;
@BeforeClass
public static void setUp(){
driver = new FirefoxDriver();
}
@Test
public void testTakeScreenShot() throws IOException {
driver.get("https://memorynotfound.com/");
takeScreenShot();
}
@Test
public void testTakeScreenShotElement() throws IOException {
driver.get("https://memorynotfound.com/");
takeScreenShot(driver.findElement(By.id("tag_cloud-2")));
}
public void takeScreenShot() throws IOException {
File scrFile = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
String fileName = UUID.randomUUID().toString();
File targetFile = new File(FOLDER + fileName + ".png");
FileUtils.copyFile(scrFile, targetFile);
}
public void takeScreenShot(WebElement element) throws IOException {
File screen = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
Point p = element.getLocation();
Rectangle rect = new Rectangle(element.getSize().getWidth(), element.getSize().getHeight());
BufferedImage img = ImageIO.read(screen);
BufferedImage dest = img.getSubimage(p.getX(), p.getY(), rect.width, rect.height);
ImageIO.write(dest, "png", screen);
FileUtils.copyFile(screen, new File(FOLDER + System.nanoTime() + ".png"));
}
@AfterClass
public static void cleanUp(){
if (driver != null){
driver.close();
driver.quit();
}
}
}
Selenium ScreenShots
Selenium Screenshot Full Web Page
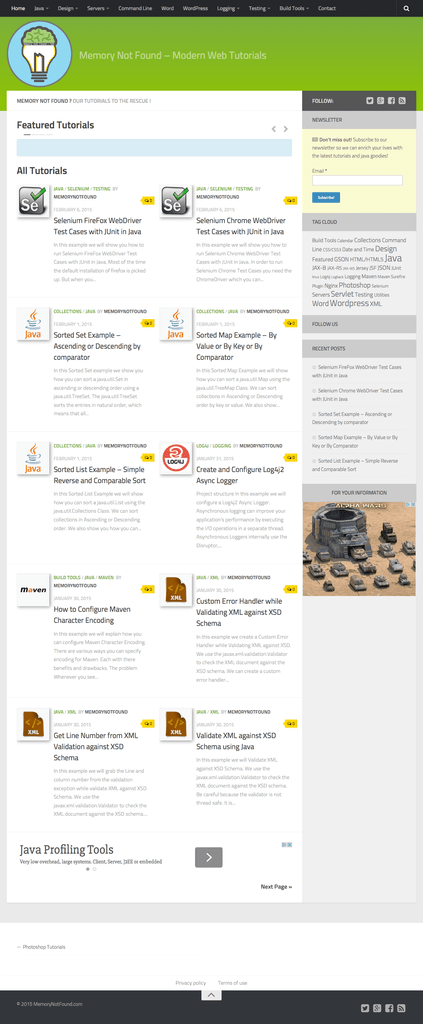
Selenium Screenshot Element
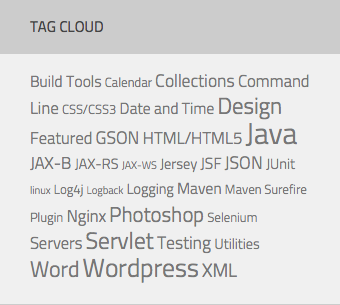