JSF Validate Length Input Field Example
This tutorial shows how to use f:validateLenght
JSF Validate Length Input Field validate. This validator validates if an input field has the specified length. This element takes two attributes minimum and maximum to configure the required length.
JSF Managed Bean
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class UserBean {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Validate Emtpy Input
If you are experiencing that JSF does not validate empty fields, then you should add the following snippet to your web.xml. Using this property tells JSF to validate empty fields.
<context-param>
<param-name>javax.faces.VALIDATE_EMPTY_FIELDS</param-name>
<param-value>true</param-value>
</context-param>
JSF Validate Length Input Field Page
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core">
<h:body>
<h2>JSF validate length input field example</h2>
<h:form>
<h:panelGrid columns="2">
<h:outputLabel value="Name:">
<h:inputText label="Name" id="name" value="#{userBean.name}">
<f:validateLength minimum="2" maximum="6"/>
</h:inputText>
</h:outputLabel>
<h:message for="name" style="color:red" />
</h:panelGrid>
<h:commandButton value="submit" action="result"/>
</h:form>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-validate-length/
JSF Validate Length – Minimum

JSF Validate Length – Maximum
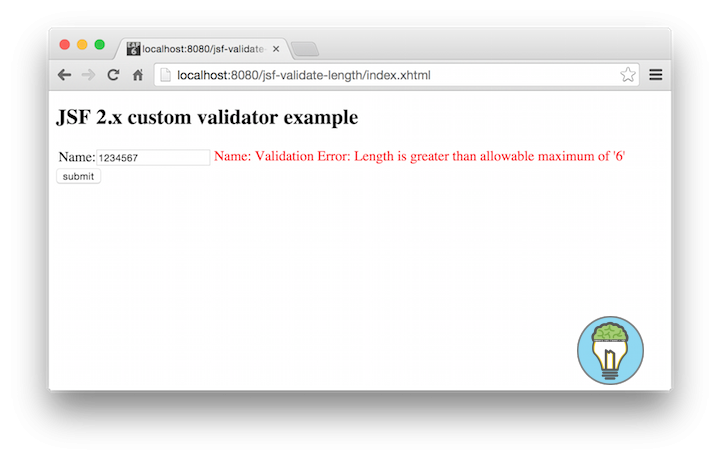