JSF Validate Bean using javax.validation Annotations
This tutorial shows how to validate a JSF Form using javax.validation
annotations on your managed bean. According to the JSR 303: Bean Validation Specification we can Annotate Classes, Methods or properties using the javax.validation
annotations. In order to use these annotations we need to add the following maven dependencies: the java validation API and a Reference Implementation (RI).
<!-- java validation API and RI-->
<dependency>
<groupId>javax.validation</groupId>
<artifactId>validation-api</artifactId>
<version>1.1.0.Final</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-validator</artifactId>
<version>5.1.3.Final</version>
</dependency>
JSF Managed Bean
- @Size: The annotated element size must be between the specified boundaries (included).
- @Pattern: The annotated String must match the following regular expression.
If you need other validations you can lookup all the annotations here.
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import javax.validation.constraints.*;
@ManagedBean
@RequestScoped
public class UserBean {
@Size(min = 3, message = "Name should at least be 3 characters long")
private String name;
@Pattern(regexp = "^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\\.[a-zA-Z0-9-.]+$", message = "This is not a valid email")
private String email;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
JSF Validate Length Input Field Page
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html">
<h:body>
<h2>JSF validate bean example</h2>
<h:form>
<h:panelGrid columns="3">
<h:outputLabel value="Name:" for="name"/>
<h:inputText id="name" value="#{userBean.name}"/>
<h:message for="name" style="color:red" />
<h:outputLabel value="Email:" for="email"/>
<h:inputText id="email" value="#{userBean.email}"/>
<h:message for="email" style="color:red" />
</h:panelGrid>
<h:commandButton value="submit" action="result"/>
</h:form>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-validate-bean/
JSF Validate Bean
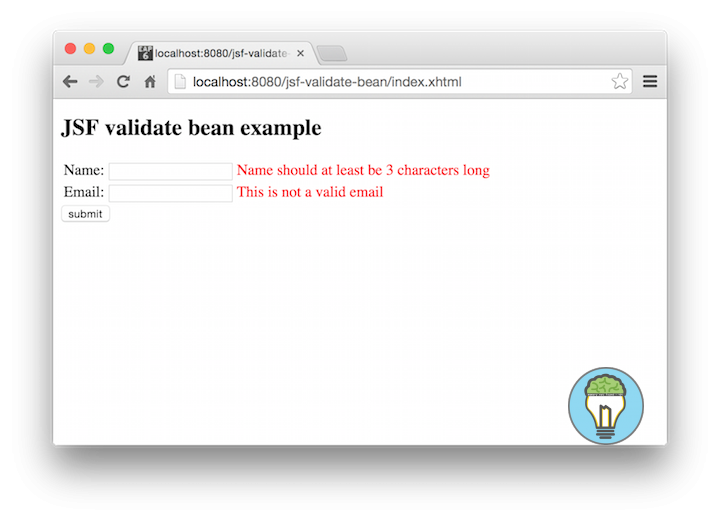