Defining and Passing JSF Context Parameters
This tutorial shows you how Context Parameters are defined in web.xml file and how you can access them in you JSF Managed bean or using the EL Expression language to retrieve them.
Create a JSF Context Parameters in web.xml
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<display-name>JavaServerFaces</display-name>
<!-- Change to "Production" when you are ready to deploy -->
<context-param>
<param-name>javax.faces.PROJECT_STAGE</param-name>
<param-value>Development</param-value>
</context-param>
<context-param>
<param-name>com.memorynotfound.title</param-name>
<param-value>Passing JSF Context Parameters</param-value>
</context-param>
<!-- Welcome page -->
<welcome-file-list>
<welcome-file>index.xhtml</welcome-file>
</welcome-file-list>
<!-- JSF mapping -->
<servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<!-- Map these files with JSF -->
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
</web-app>
Access JSF Context Parameters from Managed Bean
package com.memorynotfound;
import javax.annotation.PostConstruct;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import javax.faces.context.FacesContext;
@ManagedBean
@RequestScoped
public class AppController {
private String title;
@PostConstruct
public void init(){
title = FacesContext.getCurrentInstance().getExternalContext().getInitParameter("com.memorynotfound.title");
}
public String getTitle() {
return title;
}
}
JSF Context Param from implicit object and managed bean
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html">
<h:body>
<h1>JSF 2.2 Passing JSF Context Parameters</h1>
<h4>Using initParam</h4>
<p>#{initParam['com.memorynotfound.title']}</p>
<h4>Using facesContext</h4>
<p>#{facesContext.externalContext.initParameterMap['com.memorynotfound.title']}</p>
<h4>Using managed bean</h4>
<p>#{appController.title}</p>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-context-param/
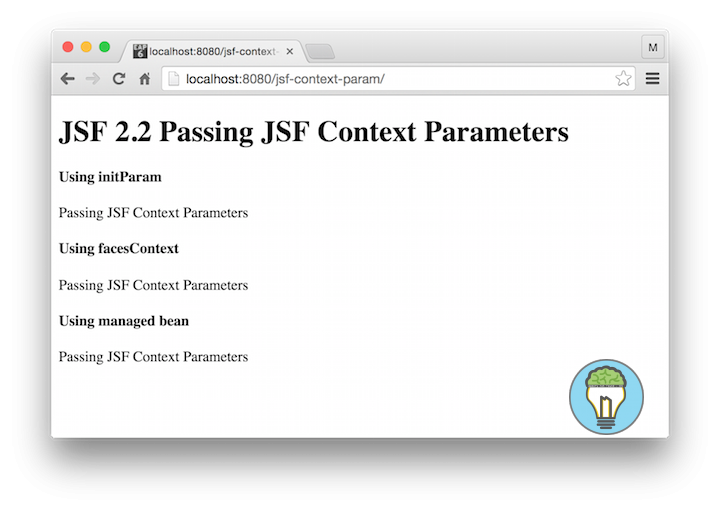