JSF Handle Bookmarkable POST redirect GET Problem
This example show how to make a form submission in JSF bookmarkable. It is called the POST redirect GET problem. Prior to JSF 2.0 it was impossible to add the form parameters to the URL. With JSF 2.0 we have new view parameters which add the parameters to the URL.
Bookmarkable POST redirect GET Managed Bean
This managed bean has a method toUpper()
which simply gets the input parameters from a page and converts that string to upper case. Afterwards it tell’s JSF to make a redirect and include all the view parameters in the URL.
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class UserBean {
private String firstName;
private String lastName;
public String toUpper(){
firstName = firstName.toUpperCase();
lastName = lastName.toUpperCase();
return "result?faces-redirect=true&includeViewParams=true";
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
JSF Bookmarkable POST redirect GET
We can pass the attributes in two ways:
- Passing to another page: when we map the action attribute of the commandbutton we can tell JSF to do a redirect by specifying the faces-redirect parameter to true and include the view parameters by setting the includeViewParameters to true.
- Passing to a managed bean: we can also pass the action to a managed bean and let the managed bean handle the navigation.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF 2.2 Bookmarkable POST redirect GET view parameters</h1>
<h:form>
<h:panelGrid columns="2">
<h:outputLabel value="Enter first name: " for="firstName"/>
<h:inputText id="firstName" value="#{userBean.firstName}"/>
<h:outputLabel value="Enter last name: " for="firstName"/>
<h:inputText id="lastName" value="#{userBean.lastName}"/>
<h:commandButton value="Save" action="result?faces-redirect=true&includeViewParams=true"/>
<h:commandButton value="Save With BackingBean" action="#{userBean.toUpper}"/>
</h:panelGrid>
</h:form>
</h:body>
</html>
JSF Bookmarkable POST redirect Result
Here we read the view parameters from the URL and map them to the appropriate setter methods of the managed bean.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:f="http://xmlns.jcp.org/jsf/core"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:head>
<f:metadata>
<f:viewParam name="fistName" value="#{userBean.firstName}" required="true" />
<f:viewParam name="lastName" value="#{userBean.lastName}" required="true" />
</f:metadata>
</h:head>
<h:body>
<h:messages style="color: red"/>
<h1>JSF 2.2 Bookmarkable POST redirect GET view parameters</h1>
Requested first name: #{userBean.firstName}
Requested last name: #{userBean.lastName}
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-view-parameters-post-redirect/
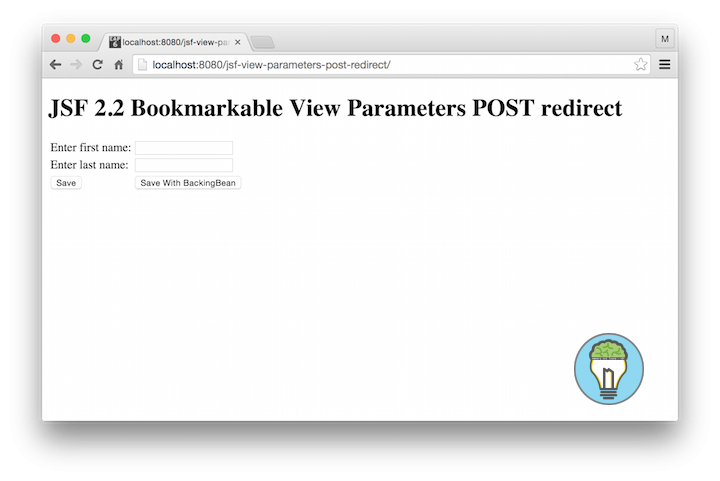
URL: http://localhost:8080/jsf-view-parameters-post-redirect/result.xhtml?fistName=John&lastName=Doe
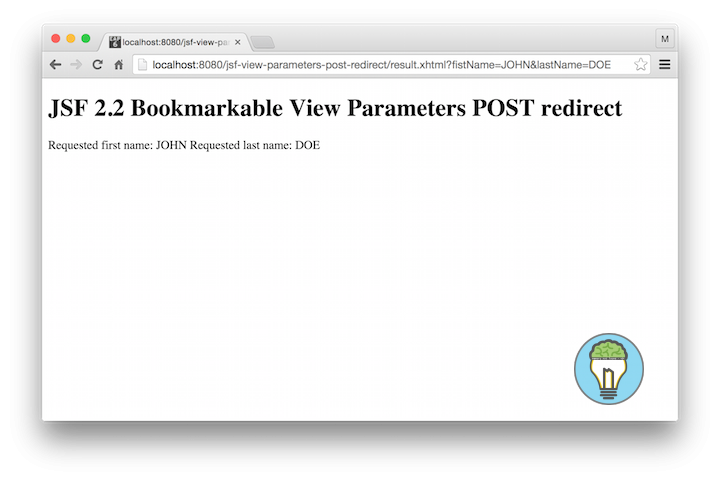
URL: http://localhost:8080/jsf-view-parameters-post-redirect/result.xhtml?fistName=JOHN&lastName=DOE
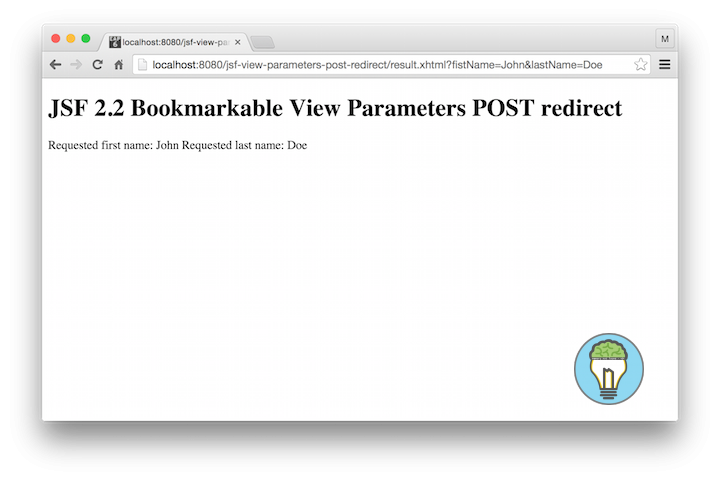