Creating Lists and Maps as Managed Beans in JSF
This example explains how to use Lists and Maps as Managed Beans in JSF. It is recommend to put the managed beans in a separate managed-beans.xml file to keep a clear overview of managed beans that have been registered with xml in our application.
Configuring Lists and Maps as managed beans in JSF
managed-beans.xml
We can configure Lists and Maps as Managed Beans using JSF. We assign a name that we can use in the view to reference the bean. We must provide an implementation class of the Map or List because JSF cannot choose an implementation at runtime.
Note: that the sample configuration uses ‘none’ as the scope for the bean. This is because the managed bean is created per request and will not be stored anywhere in any scope.
<faces-config xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-facesconfig_2_2.xsd" version="2.2">
<managed-bean>
<managed-bean-name>colors</managed-bean-name>
<managed-bean-class>java.util.ArrayList</managed-bean-class>
<managed-bean-scope>none</managed-bean-scope>
<list-entries>
<value>Red</value>
<value>Green</value>
<value>Blue</value>
</list-entries>
</managed-bean>
<managed-bean>
<managed-bean-name>beers</managed-bean-name>
<managed-bean-class>java.util.HashMap</managed-bean-class>
<managed-bean-scope>none</managed-bean-scope>
<map-entries>
<key-class>java.lang.String</key-class>
<value-class>java.lang.Integer</value-class>
<map-entry>
<key>La Chouffe</key>
<value>1</value>
</map-entry>
<map-entry>
<key>Westmalle</key>
<value>3</value>
</map-entry>
<map-entry>
<key>Chimay</key>
<value>2</value>
</map-entry>
</map-entries>
</managed-bean>
</faces-config>
Registering the managed-beans.xml in the Servlet Descriptor
web.xml
The javax.faces.CONFIG_FILES
initialization parameter tells JSF where to look for configuration files. So if you created a separate managed-beans.xml file you must register that file in this parameter, as described below.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<display-name>JavaServerFaces</display-name>
<!-- Change to "Production" when you are ready to deploy -->
<context-param>
<param-name>javax.faces.PROJECT_STAGE</param-name>
<param-value>Development</param-value>
</context-param>
<context-param>
<param-name>javax.faces.CONFIG_FILES</param-name>
<param-value>/WEB-INF/managed-beans.xml</param-value>
</context-param>
<!-- Welcome page -->
<welcome-file-list>
<welcome-file>index.xhtml</welcome-file>
</welcome-file-list>
<!-- JSF mapping -->
<servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<!-- Map these files with JSF -->
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
</web-app>
Displaying Managed Lists and Maps
We can reference the map and list we created using the managed-bean-name from the managed beans configuration file.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF List Map Managed Beans</h1>
<h3>JSF List Managed Bean</h3>
<h:panelGrid columns="2">
<h:outputLabel value="Colors: "/>
<h:outputText value="#{colors}"/>
</h:panelGrid>
<h3>JSF Map Managed Bean</h3>
<h:panelGrid columns="2">
<h:outputLabel value="Beers: "/>
<h:outputText value="#{beers}"/>
</h:panelGrid>
</h:body>
</html>
Demo
URL: http://localhost:8080/list-map-managed-beeans-jsf/
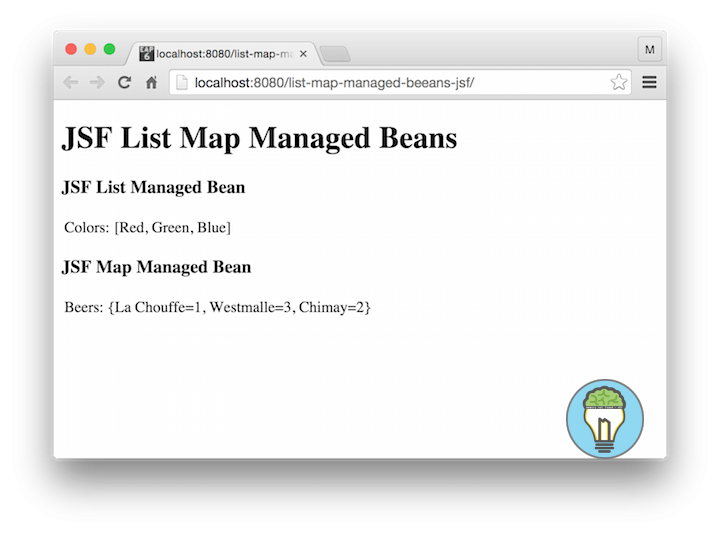