CDI Managed Bean example with @Named
This tutorial show how to create CDI managed beans. CDI is preferred over plain JSF backing beans because CDI allows for Java EE wide dependency injection. In a future release of JSF the @Managedbean
will be removed from the JSF package. CDI is more powerful than the JSF managed bean because you are not limited to JSF only. You can inject every bean managed by CDI. In this tutorial we show how to implement a CDI managed bean.
Dependencies
To compile our application we need the javaee-api and the JSF libraries.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.memorynotfound.jsf.managedbeans</groupId>
<artifactId>cdi-managed-bean</artifactId>
<version>1.0.0-SNAPSHOT</version>
<name>JSF - ${project.artifactId}</name>
<url>https://memorynotfound.com</url>
<packaging>war</packaging>
<dependencies>
<!-- java ee api -->
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>7.0</version>
<scope>provided</scope>
</dependency>
<!-- JSF api and impl -->
<dependency>
<groupId>com.sun.faces</groupId>
<artifactId>jsf-api</artifactId>
<version>2.2.12</version>
</dependency>
<dependency>
<groupId>com.sun.faces</groupId>
<artifactId>jsf-impl</artifactId>
<version>2.2.12</version>
</dependency>
<!-- servlet provided by tomcat -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
CDI Managed Bean Configuration
The equivalent to JSF’s @ManagedBean
is @Named
.
You cannot mix JSF and CDI annotations in the same class. If you want to use either CDI or JSF managed beans make sure you implement the right package, otherwise it will not work. You can have JSF and CDI managed beans in the same application, but not in the same class.
package com.memorynotfound;
import javax.enterprise.context.RequestScoped;
import javax.inject.Named;
@Named
@RequestScoped
public class UserBean {
private String firstName;
private String lastName;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
Enable CDI
To enable CDI you must have at least an empty beans.xml in the WEB-INF directory.
<?xml version="1.0" encoding="UTF-8"?>
<beans bean-discovery-mode="all" version="1.1"
xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/beans_1_1.xsd">
</beans>
Configure JSF
The servlet descriptor is the same as a normal JSF managed application.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<display-name>JavaServerFaces</display-name>
<!-- Change to "Production" when you are ready to deploy -->
<context-param>
<param-name>javax.faces.PROJECT_STAGE</param-name>
<param-value>Development</param-value>
</context-param>
<!-- Welcome page -->
<welcome-file-list>
<welcome-file>index.xhtml</welcome-file>
</welcome-file-list>
<!-- JSF mapping -->
<servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<!-- Map these files with JSF -->
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
</web-app>
Calling CDI Managed Bean
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF 2.2 CDI Managed Bean</h1>
<h:form>
<h:panelGrid columns="2">
<h:outputLabel value="First name" for="firstName"/>
<h:inputText id="firstName" value="#{userBean.firstName}"/>
<h:outputLabel value="Last name" for="lastName"/>
<h:inputText id="lastName" value="#{userBean.lastName}"/>
<h:commandButton action="result" value="send"/>
</h:panelGrid>
</h:form>
</h:body>
</html>Calling CDI Managed Bean
Displaying result
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF 2.2 CDI Managed Bean</h1>
First name: #{userBean.firstName}
Last name: #{userBean.lastName}
</h:body>
</html>
Demo
URL: http://localhost:8080/cdi-managed-bean/
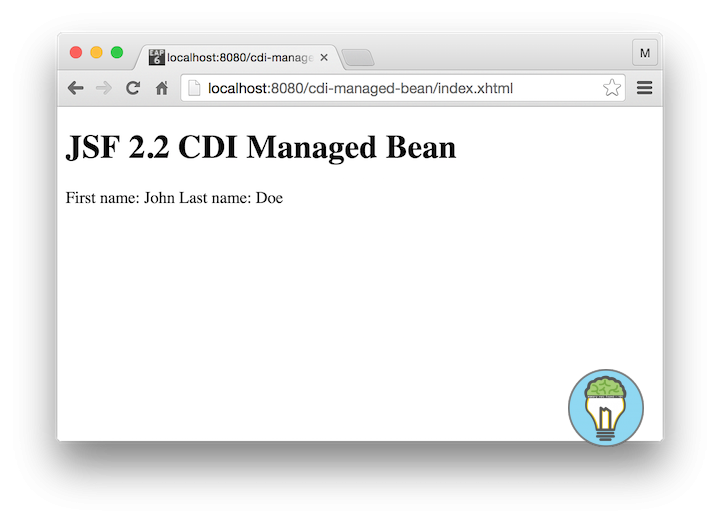
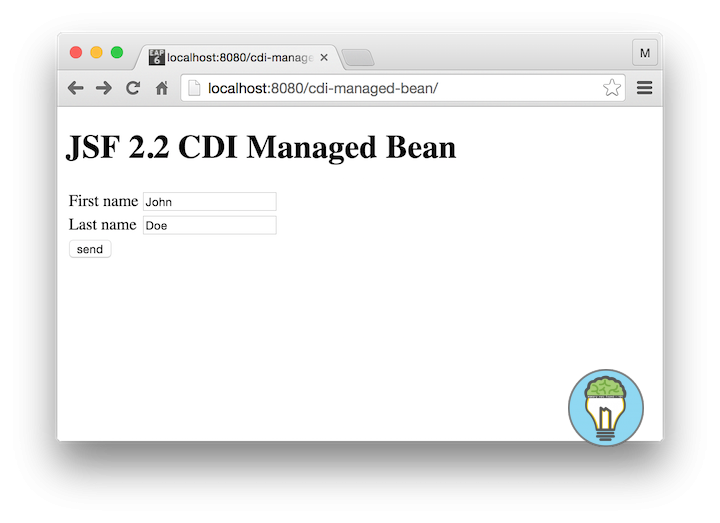