JSF Access Managed Bean Programatically
This tutorials shows how to access a JSF or CDI managed bean programatically. Sometimes, you may need to access a JSF or CDI managed bean from an event listener or another managed bean. You can accomplish this task in the following ways:
By using the evaluateExpressionGet method
FacesContext context = FacesContext.getCurrentInstance(); Application application = context.getApplication(); ProfileBean profileBean = application.evaluateExpressionGet(context, "#{profileBean}", ProfileBean.class);
By using the evaluateValueExpression method
FacesContext context = FacesContext.getCurrentInstance(); Application application = context.getApplication(); ELContext elContext = context.getELContext(); ProfileBean profileBean = (ProfileBean) application.getExpressionFactory() .createValueExpression(elContext, "#{profileBean}", ProfileBean.class).getValue(elContext);
By using the ELResolver
FacesContext context = FacesContext.getCurrentInstance(); ELContext elContext = context.getELContext(); ProfileBean profileBean = (ProfileBean) elContext.getELResolver().getValue(elContext, null, "profileBean");
Access Managed Bean Programatically
Lets put the theory in practice. We define a session scoped managed bean which we’ll access programatically from another managed bean.
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.SessionScoped;
import java.io.Serializable;
@ManagedBean
@SessionScoped
public class ProfileBean implements Serializable {
private String name = "I'm a profile";
public String getName() {
return name;
}
}
We access the managed bean programatically using different methods.
package com.memorynotfound;
import javax.el.ELContext;
import javax.faces.application.Application;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import javax.faces.context.FacesContext;
@ManagedBean
@RequestScoped
public class UserBean {
public ProfileBean getManagedBeanByEvaluateExpressionGet(){
FacesContext context = FacesContext.getCurrentInstance();
Application application = context.getApplication();
return application.evaluateExpressionGet(context, "#{profileBean}", ProfileBean.class);
}
public ProfileBean getManagedBeanByValueExpression(){
FacesContext context = FacesContext.getCurrentInstance();
Application application = context.getApplication();
ELContext elContext = context.getELContext();
return (ProfileBean) application.getExpressionFactory()
.createValueExpression(elContext, "#{profileBean}", ProfileBean.class).getValue(elContext);
}
public ProfileBean getManagedBeanByElResolver(){
FacesContext context = FacesContext.getCurrentInstance();
ELContext elContext = context.getELContext();
return (ProfileBean) elContext.getELResolver().getValue(elContext, null, "profileBean");
}
}
Displaying the result
For testing purposes we use the programatically accessed managed bean.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF Access managed bean programatically</h1>
<h:panelGrid columns="3">
<h:outputLabel value="Get Managed Bean By evalutateExpressionGet: "/>
<h:outputText value="#{userBean.managedBeanByEvaluateExpressionGet.name}"/>
<h:outputLabel value="(programatically from profile bean)"/>
<h:outputLabel value="Get Managed Bean By valueExpression: "/>
<h:outputText value="#{userBean.managedBeanByValueExpression.name}"/>
<h:outputLabel value="(programatically from profile bean)"/>
<h:outputLabel value="Get Managed Bean By elResolver: "/>
<h:outputText value="#{userBean.managedBeanByElResolver.name}"/>
<h:outputLabel value="(programatically from profile bean)"/>
</h:panelGrid>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-managed-bean-programatically/
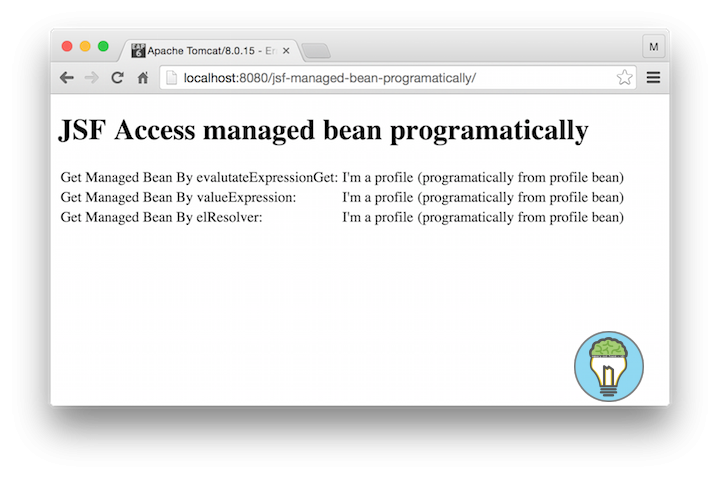