JSF Passing Parameters via Method Expression
In this example we’ll talk about passing parameters via method expression. When you need to pass a parameter to a managed bean method, you can just pass the parameter between brackets. You can pass numeric values, String values and Objects.
Passing parameters via method expression
Just a simple POJO.
package com.memorynotfound;
public class Course {
private Integer id;
private String name;
public Course(Integer id, String name) {
this.id = id;
this.name = name;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
The delete()
method has the Course
class as a parameter. We can simply reference a Course
object from our view to the managed bean.
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.SessionScoped;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
@ManagedBean
@SessionScoped
public class CourseBean implements Serializable {
static List<Course> courseList = new ArrayList<Course>();
public CourseBean(){
courseList.add(new Course(1, "Programming JSF"));
courseList.add(new Course(2, "Programming CDI"));
courseList.add(new Course(3, "Programming Facelets"));
}
public List<Course> getCourses(){
return courseList;
}
public String delete(Course course){
courseList.remove(course);
return null;
}
}
Passing the parameter via method expressions
We have a data table which passes the Course
object in every column in order to have a reference of which object to delet.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF Method Expression Example</h1>
<h:form>
<h:dataTable id="courses" var="course" value="#{courseBean.courses}">
<h:column>
<h:outputText value="#{course.name}"/>
</h:column>
<h:column>
<h:commandLink value="delete" action="#{courseBean.delete(course)}"/>
</h:column>
</h:dataTable>
</h:form>
</h:body>
</html>
Demo
URL: localhost:8080/jsf-method-expression/
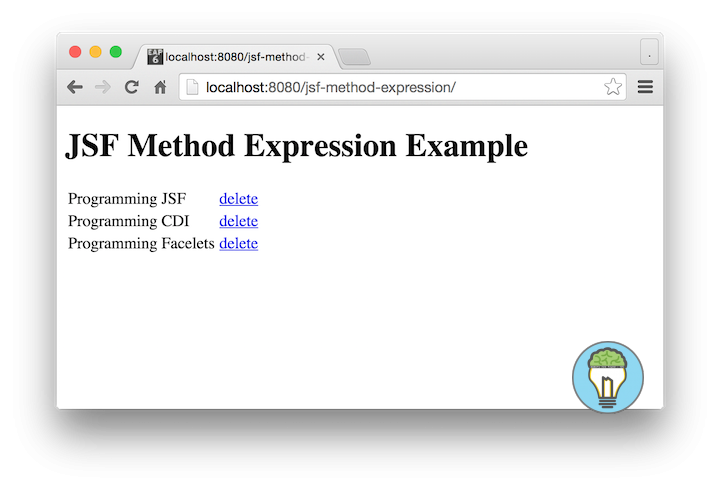