Trigger custom AJAX function onevent with JSF
In this tutorial we will trigger a custom AJAX function onevent with JSF. You can bind a javascript function when an AJAX event occurs in different ways. You can either bind a javascript function using the onevent attribute of the <f:ajax>
element. Or you can globally bind an event listener using the jsf.ajax.addOnEvent(methodName)
function.
Processing Managed Bean
In the example we’ll display a loading gif to indicate the process is executing. We use the wait()
method to execute this method for 3 seconds.
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class ProcessingBean {
public void processing(){
synchronized(this){
try {
wait(3000);
} catch (InterruptedException e1) {
// waiting interrupted
}
}
}
}
Execute Custom JavaScript on JSF AJAX onevent
We can bind a custom JavaScript function using either the onevent attribute of the <f:ajax>
element. Or by globally defining an event listener using the jsf.ajax.addOnEvent(methodName)
function. Both methods require the jsf.js script.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:f="http://xmlns.jcp.org/jsf/core">
<h:head>
<h:outputScript library="js" name="custom-script.js" target="body"/>
<h:outputScript library="javax.faces" name="jsf.js" target="body"/>
<script type="text/javascript">
//jsf.ajax.addOnEvent(onStatusChange);
</script>
</h:head>
<h:body>
<h2>JSF 2: onevent ajax</h2>
<h:form id="user-form">
<h:panelGrid columns="1">
<img src="resources/img/ajax-loader.gif" id="spinner" style="display: none"/>
<h:commandButton value="Submit" actionListener="#{processingBean.processing}">
<f:ajax render="@form" onevent="onStatusChange" />
</h:commandButton>
</h:panelGrid>
</h:form>
</h:body>
</html>
Info: In order for the javascript selectors to work we have to change our default char separator.
<!-- change default separator for javascript and css selectors -->
<context-param>
<param-name>javax.faces.SEPARATOR_CHAR</param-name>
<param-value>-</param-value>
</context-param>
Custom JavaScript Loader
function onStatusChange(data) {
var spinner = document.getElementById("spinner");
var status = data.status;
if (status === "begin") {
spinner.style.display = "inline";
} else {
spinner.style.display = "none";
}
}
Demo
URL: localhost:8081/jsf-ajax-onevent
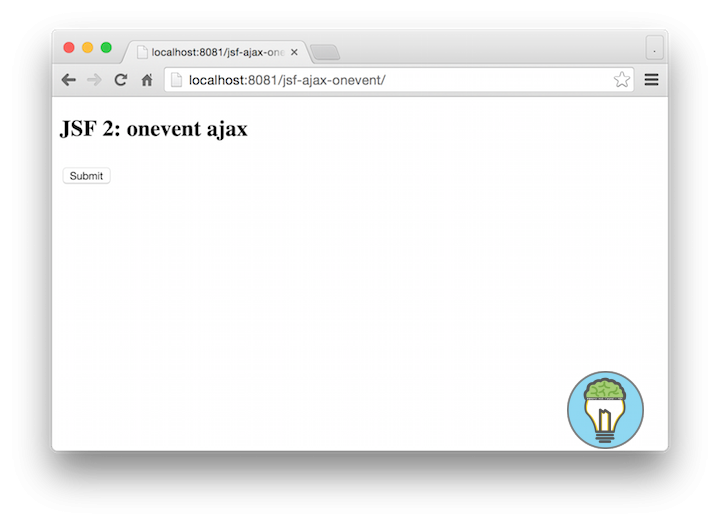
When the button is pressed.
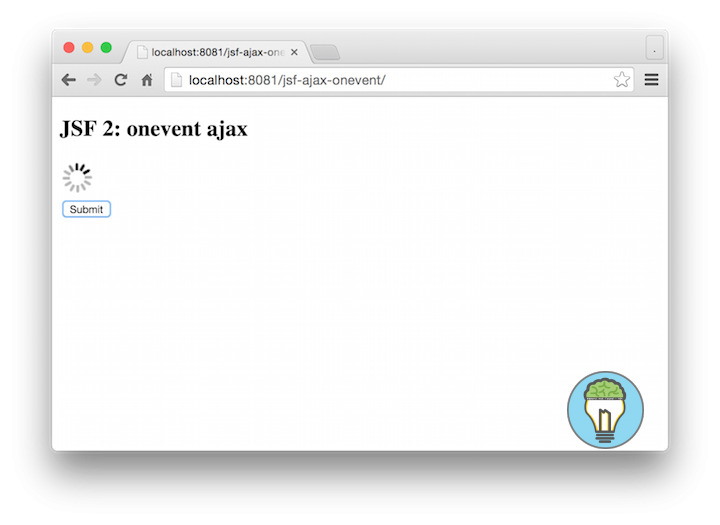