JSF Select One And Select Many Checkboxes Example
In this tutorial we will show you how to use checkboxes in JSF. We can have two types of checkboxes:
- SelectBooleanCheckbox: assign a single value to the managed property.
- selectManyCheckbox: assigns an array of values to the managed property.
Checkboxes Generated Output
the <h:selectBooleanCheckbox>
is used to render a single input element of type “checkbox.
// JSF
<h:selectBooleanCheckbox id="existing" value="#{user.existing}" />
// Generated HTML
<input id="cf:existing" type="checkbox" name="cf:existing" />
the <h:selectManyCheckbox>
is used to render a multiple input elements of type “checkbox. The checkbox group is also formatted inside an HTML table with complementary labels.
// JSF
<h:selectManyCheckbox id="course1" value="#{user.course1}">
<f:selectItem itemValue="1" itemLabel="Java" />
<f:selectItem itemValue="2" itemLabel="Scala" />
<f:selectItem itemValue="3" itemLabel="Groovy" />
<f:selectItem itemValue="4" itemLabel="Ruby" />
</h:selectManyCheckbox>
// Generated HTML
<table id="cf:course1">
<tr>
<td>
<input name="cf:course1" id="cf:course1:0" value="1" type="checkbox" />
<label for="cf:course1:0" class=""> Java</label>
</td>
<td>
<input name="cf:course1" id="cf:course1:1" value="2" type="checkbox" />
<label for="cf:course1:1" class=""> Scala</label>
</td>
<td>
<input name="cf:course1" id="cf:course1:2" value="3" type="checkbox" />
<label for="cf:course1:2" class=""> Groovy</label>
</td>
<td>
<input name="cf:course1" id="cf:course1:3" value="4" type="checkbox" />
<label for="cf:course1:3" class=""> Ruby</label>
</td>
</tr>
</table>
JSF Checkboxes Example
Managed Bean for Submitted Values
This managed bean will hold the values submitted from the index.xhtml page.
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import java.util.Arrays;
@ManagedBean(name = "user")
@RequestScoped
public class UserBean {
private boolean existing;
private String[] course1;
private String[] course2;
private String[] course3;
private String[] course4;
public boolean isExisting() {
return existing;
}
public void setExisting(boolean existing) {
this.existing = existing;
}
public String[] getCourse1() {
return course1;
}
public void setCourse1(String[] course1) {
this.course1 = course1;
}
public String[] getCourse2() {
return course2;
}
public void setCourse2(String[] course2) {
this.course2 = course2;
}
public String[] getCourse3() {
return course3;
}
public void setCourse3(String[] course3) {
this.course3 = course3;
}
public String[] getCourse4() {
return course4;
}
public void setCourse4(String[] course4) {
this.course4 = course4;
}
public String getCourse1toString(){
return Arrays.toString(course1);
}
public String getCourse2toString(){
return Arrays.toString(course2);
}
public String getCourse3toString(){
return Arrays.toString(course3);
}
public String getCourse4toString(){
return Arrays.toString(course4);
}
@Override
public String toString() {
return "UserBean{" +
"existing=" + existing +
", course1=" + Arrays.toString(course1) +
", course2=" + Arrays.toString(course2) +
", course3=" + Arrays.toString(course3) +
", course4=" + Arrays.toString(course4) +
'}';
}
}
Managed Bean Providing the data
This managed bean provides the data used by the <h:selectManyCheckbox>
.
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import java.util.LinkedHashMap;
import java.util.Map;
@ManagedBean(name = "courses")
@RequestScoped
public class CourseBean {
public String[] getCourseArray() {
return new String[] {"Java", "Scala", "Groovy", "Ruby"};
}
public Map<String, Object> getCourseMap(){
Map<String, Object> courses = new LinkedHashMap<String, Object>();
courses.put("Java", "1");
courses.put("Scala", "2");
courses.put("Groovy", "3");
courses.put("Ruby", "4");
return courses;
}
public Course[] getCourseObjectArray(){
return new Course[]{
new Course("Java", "1"),
new Course("Scala", "2"),
new Course("Groovy", "3"),
new Course("Ruby", "4")};
}
}
Object representation of checkbox
This object is used to pass an Object Array into the <h:selectManyCheckbox>
.
package com.memorynotfound.jsf;
public class Course {
public String label;
public String value;
public Course(String label, String value) {
this.label = label;
this.value = value;
}
public String getLabel() {
return label;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return "Course{" +
"label='" + label + '\'' +
", value='" + value + '\'' +
'}';
}
}
Demonstration of selectBooleanCheckbox and selectManyCheckbox
This page demonstrates how to use <h:selectBooleanCheckbox>
and <h:selectManyCheckbox>
.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core">
<h:body>
<h1>JSF 2 checkboxes example</h1>
<h:form id="cf">
<h2>Single checkbox</h2>
<h4>1. assigned boolean value</h4>
<h:outputLabel value="Are you a new member?">
<h:selectBooleanCheckbox id="existing" value="#{user.existing}" />
</h:outputLabel>
<h2>Multiple checkboxes</h2>
<h4>2. with hardcoded values</h4>
<h:selectManyCheckbox id="course1" value="#{user.course1}">
<f:selectItem itemValue="1" itemLabel="Java" />
<f:selectItem itemValue="2" itemLabel="Scala" />
<f:selectItem itemValue="3" itemLabel="Groovy" />
<f:selectItem itemValue="4" itemLabel="Ruby" />
</h:selectManyCheckbox>
<h4>3. with array</h4>
<h:selectManyCheckbox id="course2" value="#{user.course2}">
<f:selectItems value="#{courses.courseArray}" />
</h:selectManyCheckbox>
<h4>4. with map</h4>
<h:selectManyCheckbox id="course3" value="#{user.course3}">
<f:selectItems value="#{courses.courseMap}" />
</h:selectManyCheckbox>
<h4>5. with object array</h4>
<h:selectManyCheckbox id="course4" value="#{user.course4}">
<f:selectItems value="#{courses.courseObjectArray}" var="course"
itemLabel="#{course.label}" itemValue="#{course.value}" />
</h:selectManyCheckbox>
<h:commandButton id="submit" value="Submit" action="result" />
<h:commandButton id="reset" value="Reset" type="reset" />
</h:form>
</h:body>
</html>
This page is used for displaying the submitted values.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html">
<h:body>
<h1>JSF 2 checkboxes example</h1>
<ol>
<li><h:outputText value="existing: #{user.existing}"/></li>
<li><h:outputText value="hardcoded: #{user.course1toString}"/></li>
<li><h:outputText value="array: #{user.course2toString}"/></li>
<li><h:outputText value="map: #{user.course3toString}"/></li>
<li><h:outputText value="object array: #{user.course4toString}"/></li>
</ol>
</h:body>
</html>
Demo
URL: http://localhost:8081/jsf-checkbox/
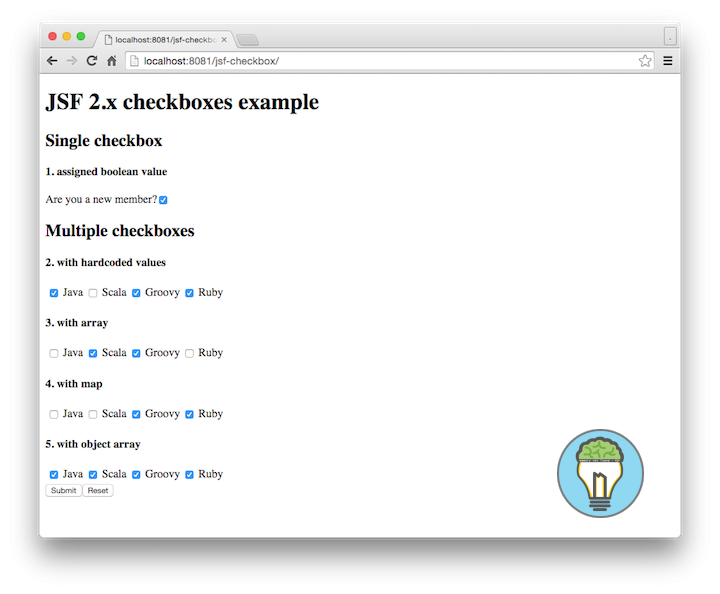
Result page showing the submitted values.
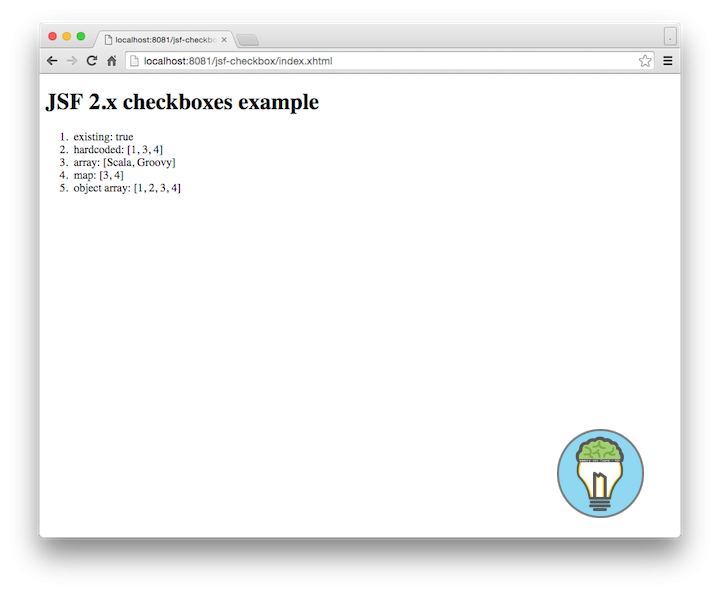