Generate Java Classes From XSD
This example shows you how to generate Java Classes from XSD using jaxb2-maven-plugin
. This plugin uses the XJC which is a JAXB Binding compiler tool that can generate Java Classes from XSD.
Maven Project Dependencies
We use the jaxb2-maven-plugin
maven plugin to transform our XSD schema into Java Classes. These Java Classes will be generated in the target/generated-resources/jaxb folder by default. You could override the output directory by adding a child element outputDirectory to the configuration element. If you use this custom output directory your files will be cleared/replaced on every build. If you add extra classes to these directories you must also add the clearOutputDir with a value of false.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.memorynotfound.xml.jaxb</groupId>
<artifactId>generate-java-classes-from-xsd</artifactId>
<version>1.0.0-SNAPSHOT</version>
<name>XSD - ${project.artifactId}</name>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
</properties>
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>jaxb2-maven-plugin</artifactId>
<version>2.2</version>
<executions>
<execution>
<id>xjc</id>
<goals>
<goal>xjc</goal>
</goals>
</execution>
</executions>
<configuration>
<xjbSources>
<xjbSource>src/main/resources/global.xjb</xjbSource>
</xjbSources>
<sources>
<source>src/main/resources/schema.xsd</source>
</sources>
</configuration>
</plugin>
</plugins>
</build>
</project>
Global JAX-B bindings
We can provide a global bindings file. These binding declarations override the default binding rules. Like in this example we override the dateTime type to the java.util.Calendar
type.
<?xml version="1.0" encoding="UTF-8"?>
<jaxb:bindings version="2.1"
xmlns:jaxb="http://java.sun.com/xml/ns/jaxb"
xmlns:xs="http://www.w3.org/2001/XMLSchema">
<jaxb:globalBindings>
<jaxb:serializable uid="1"/>
<!-- date time adapter -->
<jaxb:javaType name="java.util.Calendar" xmlType="xs:dateTime"
parseMethod="javax.xml.bind.DatatypeConverter.parseDateTime"
printMethod="javax.xml.bind.DatatypeConverter.printDateTime" />
</jaxb:globalBindings>
</jaxb:bindings>
Generate Java Classes from XSD
This XSD schema will be used to generate the Java Classes. It is important to always change your XSD file and let the classes be generated. Don’t modify the generated Java Classes because these are re-generated on every build.
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema"
targetNamespace="http://services.memorynotfound.com/"
elementFormDefault="qualified">
<xs:complexType name="CourseRequest">
<xs:sequence>
<xs:element name="id" type="xs:int" nillable="false"/>
</xs:sequence>
</xs:complexType>
<xs:complexType name="CourseResponse">
<xs:sequence>
<xs:element name="id" type="xs:int"/>
<xs:element name="name" type="xs:string"/>
<xs:element name="description" type="xs:string"/>
<xs:element name="created" type="xs:dateTime"/>
</xs:sequence>
</xs:complexType>
</xs:schema>
Running the example
When we run this example with maven, the java classes are created from the xsd schema using the jaxb2-maven-plugin.
mvn clean package
Screenshot
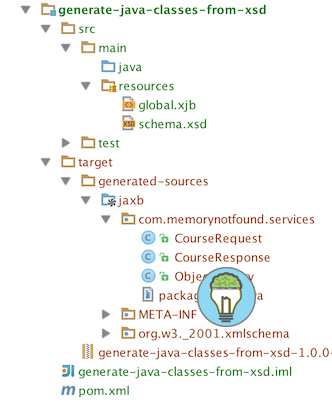
Thank you for your code. It helped A LOT!
Example demonstrates only one xsd file. In case there are more than 10 files, the source is going to include all the files or is there a way to provide folder location and get the necessary files generated?