Apache PDFBox Bookmark PDF Example
In this tutorial we demonstrate how to create Bookmarks in a PDF document using Apache PDFBox. In the context of a PDF document, you can attach a bookmark to a section of a specific page. You can add an action to this bookmark like navigation. In Adobe Acrobat Reader you can view the PDF Bookmarks in the left column.
This examples will show you three examples.
- Creating Bookmarks in PDF Document
- Navigate to Bookmark Action on Open Event
- Get and Print all Bookmarks in PDF Document
Maven Dependencies
We use Apache Maven to manage our project dependencies. Make sure the following dependencies reside on the class-path.
<dependency>
<groupId>org.apache.pdfbox</groupId>
<artifactId>pdfbox</artifactId>
<version>2.0.8</version>
</dependency>
Creating Bookmarks in PDF Document
In the following example we create three pages. We add each page to the PDF document. We want to create bookmarks for each page, so we start by adding a top level category All pages using the PDDocumentOutline
class. Next, we loop over each page add a bookmarks accordingly and set the destination for these pages using a class derived from PDPageDestination
.
package com.memorynotfound.pdf.pdfbox;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.PDPage;
import org.apache.pdfbox.pdmodel.PDPageContentStream;
import org.apache.pdfbox.pdmodel.font.PDType1Font;
import org.apache.pdfbox.pdmodel.interactive.documentnavigation.destination.PDPageDestination;
import org.apache.pdfbox.pdmodel.interactive.documentnavigation.destination.PDPageFitWidthDestination;
import org.apache.pdfbox.pdmodel.interactive.documentnavigation.outline.PDDocumentOutline;
import org.apache.pdfbox.pdmodel.interactive.documentnavigation.outline.PDOutlineItem;
import java.io.File;
import java.io.IOException;
public class CreateBookmarks {
public static void main(String[] args) throws Exception{
try (final PDDocument doc = new PDDocument()){
PDPage page1 = new PDPage();
PDPage page2 = new PDPage();
PDPage page3 = new PDPage();
doc.addPage(page1);
doc.addPage(page2);
doc.addPage(page3);
PDDocumentOutline outline = new PDDocumentOutline();
doc.getDocumentCatalog().setDocumentOutline(outline);
PDOutlineItem pagesOutline = new PDOutlineItem();
pagesOutline.setTitle("All Pages");
outline.addLast(pagesOutline);
int pageNum = 1;
for (PDPage page : doc.getPages()) {
// write some content
PDPageContentStream contents = new PDPageContentStream(doc, page);
contents.beginText();
contents.newLineAtOffset(100, 700);
contents.setFont(PDType1Font.HELVETICA, 12);
contents.showText("Content page " + pageNum);
contents.endText();
contents.close();
PDPageDestination dest = new PDPageFitWidthDestination();
dest.setPage(page);
PDOutlineItem bookmark = new PDOutlineItem();
bookmark.setDestination(dest);
bookmark.setTitle("Page " + pageNum);
pagesOutline.addLast(bookmark);
pageNum++;
}
pagesOutline.openNode();
outline.openNode();
doc.save(new File("/tmp/bookmark.pdf"));
} catch (IOException e){
System.err.println("Exception while trying to create pdf document - " + e);
}
}
}
Note: if you want to have several bookmarks pointing to different areas on the same page, have a look at the other classes derived from
PDPageDestination
.
PDPageFitDestination
– represents a destination to a page and the page contents will be magnified to just fit on the screen.PDPageFitHeightDestination
– represents a destination to a page at a x location and the height is magnified to just fit on the screen.PDPageFitRectangleDestination
– represents a destination to a page at a y location and the width is magnified to just fit on the screen.PDPageFitWidthDestination
– represents a destination to a page at a y location and the width is magnified to just fit on the screen.PDPageXYZDestination
– represents a destination to a page at an x,y coordinate with a zoom setting. The default x,y,z will be whatever is the current value in the viewer application and are not required.
Demo
When we run the application, three pages are created with their bookmark accordingly.
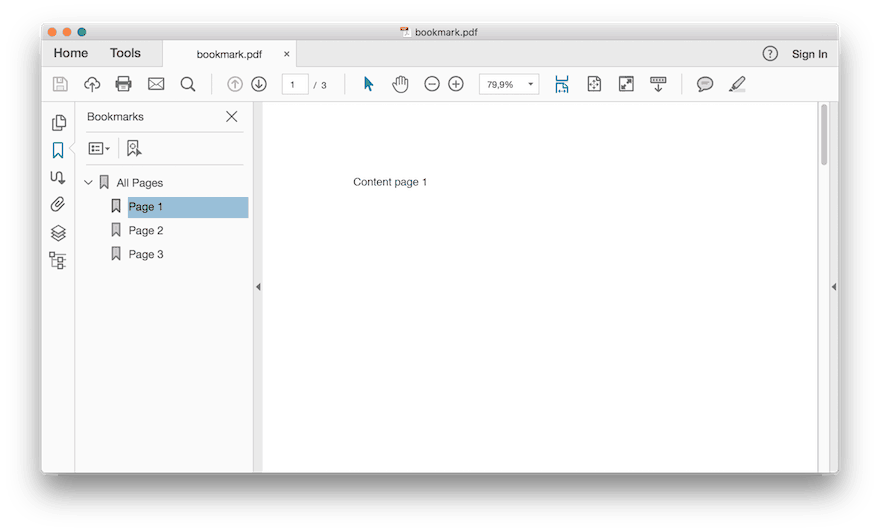
Attach an action on Open PDF Document
We can attach an action derived from PDDestinationOrAction
class using the PDDocumentCatalog#setOpenAction();
method. When the PDF document is opened this action will be called. In our case we attached a PDActionGoTo
which navigates the PDF document to a specific bookmark on the page.
package com.memorynotfound.pdf.pdfbox;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.interactive.action.PDActionGoTo;
import org.apache.pdfbox.pdmodel.interactive.documentnavigation.destination.PDDestination;
import org.apache.pdfbox.pdmodel.interactive.documentnavigation.outline.PDDocumentOutline;
import org.apache.pdfbox.pdmodel.interactive.documentnavigation.outline.PDOutlineItem;
import java.io.File;
import java.io.IOException;
public class GoToBookmarkOnOpen {
public static void main(String[] args) throws Exception{
try (final PDDocument doc = PDDocument.load(new File("/tmp/bookmark.pdf"))){
PDDocumentOutline bookmarks = doc.getDocumentCatalog().getDocumentOutline();
PDOutlineItem item = bookmarks.getFirstChild().getLastChild();
PDDestination dest = item.getDestination();
PDActionGoTo action = new PDActionGoTo();
action.setDestination(dest);
doc.getDocumentCatalog().setOpenAction(action);
doc.save(new File("/tmp/open-bookmark.pdf"));
} catch (IOException e){
System.err.println("Exception while trying to create pdf document - " + e);
}
}
}
Get and Print all Bookmarks in PDF Document
In this example we demonstrate how to get all the Bookmarks from a PDF document. Afterwards we simply print all the bookmarks to the console.
package com.memorynotfound.pdf.pdfbox;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.interactive.action.PDActionGoTo;
import org.apache.pdfbox.pdmodel.interactive.documentnavigation.destination.PDPageDestination;
import org.apache.pdfbox.pdmodel.interactive.documentnavigation.outline.PDDocumentOutline;
import org.apache.pdfbox.pdmodel.interactive.documentnavigation.outline.PDOutlineItem;
import org.apache.pdfbox.pdmodel.interactive.documentnavigation.outline.PDOutlineNode;
import java.io.File;
import java.io.IOException;
public class PrintBookmarks {
public static void main(String[] args) throws Exception{
try (final PDDocument doc = PDDocument.load(new File("/tmp/bookmark.pdf"))){
PDDocumentOutline outline = doc.getDocumentCatalog().getDocumentOutline();
if (outline != null) {
printBookmark(outline, "");
} else {
System.out.println("This document does not contain any bookmarks");
}
} catch (IOException e){
System.err.println("Exception while trying to load pdf document - " + e);
}
}
private static void printBookmark(PDOutlineNode bookmark, String indentation) throws IOException {
PDOutlineItem current = bookmark.getFirstChild();
while (current != null) {
if (current.getDestination() instanceof PDPageDestination) {
PDPageDestination pd = (PDPageDestination) current.getDestination();
System.out.println(indentation + "Destination page: " + (pd.retrievePageNumber() + 1));
}
if (current.getAction() instanceof PDActionGoTo) {
PDActionGoTo gta = (PDActionGoTo) current.getAction();
if (gta.getDestination() instanceof PDPageDestination) {
PDPageDestination pd = (PDPageDestination) gta.getDestination();
System.out.println(indentation + "Destination page: " + (pd.retrievePageNumber() + 1));
}
}
System.out.println(indentation + current.getTitle());
printBookmark(current, indentation + " - - ");
current = current.getNextSibling();
}
}
}
Demo
When we run the application, all the bookmarks are printed to the console.
All Pages
- - Destination page: 1
- - Page 1
- - Destination page: 2
- - Page 2
- - Destination page: 3
- - Page 3
References
- Apache PdfBox Official Website
- Apache PdfBox API Javadoc
- Apache PdfBox add multiline paragraph
- Apache PdfBox create PDF document
- PDDocumentOutline JavaDoc
- PDPageDestination JavaDoc