Calling an action on GET Request using JSF viewAction
This example shows how to call an action on a GET request using JSF viewAction. Since JSF 2.2 we have a new element called <f:viewAction> which is a child element of <f:metadata> . This allows us to call an action on GET requests.
JSF Managed Bean
Just a normal managed bean as you can see later the index.xhtml page is specifying a JSF viewAction and maps the action attribut to the loadUserById()
method.
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class UserBean {
private Integer id;
private User currentUser;
/**
* pre loads the user from a repository
* @return current page
*/
public String loadUserById(){
currentUser = UserRepository.findUser(id);
return null;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public User getCurrentUser() {
return currentUser;
}
}
User POJO
Class representing a user
package com.memorynotfound;
public class User {
private Integer id;
private String name;
public User(Integer id, String name) {
this.id = id;
this.name = name;
}
public Integer getId() {
return id;
}
public String getName() {
return name;
}
}
User repository
Simple repository to lookup a user
package com.memorynotfound;
public class UserRepository {
final static User[] users = {
new User(0, "user a"),
new User(1, "user b"),
new User(2, "user c")
};
public static User findUser(Integer id){
for (User user : users){
if (user.getId().equals(id)){
return user;
}
}
return null;
}
}
Mapping a JSF viewAction to a managed bean
The JSF viewAction allows us to execute a method on GET request of a page. This action has the ability to implicitly navigate to another page.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:f="http://xmlns.jcp.org/jsf/core"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:head>
<f:metadata>
<f:viewParam name="id" value="#{userBean.id}" />
<f:viewAction action="#{userBean.loadUserById}"/>
</f:metadata>
</h:head>
<h:body>
<h1>JSF ViewAction: execute method on GET Requests</h1>
<h:panelGrid columns="2">
<h:outputLabel value="User id to load: "/>
<h:outputText value="#{userBean.id}"/>
<h:outputLabel value="Loaded user id: "/>
<h:outputText value="#{userBean.currentUser.id}"/>
<h:outputLabel value="Loaded user name: "/>
<h:outputText value="#{userBean.currentUser.name}"/>
</h:panelGrid>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-viewaction/result.xhtml?id=1
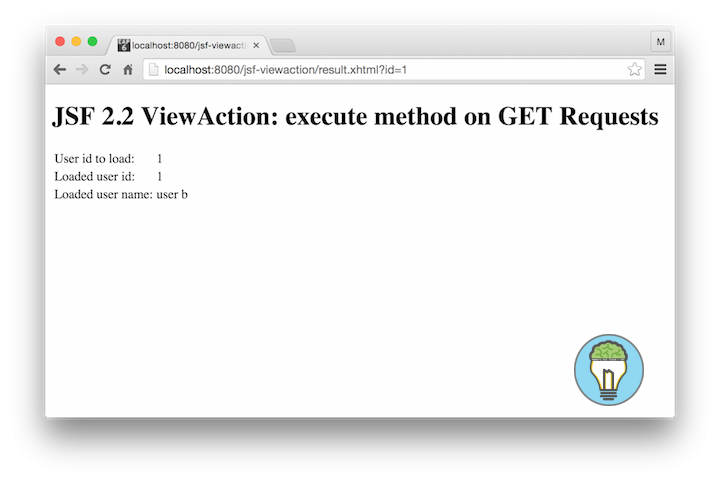
Thank you