Add, Edit Metadata of PDF Document using iText in Java
Metadata has been described as the business card of a particular digital document. The term metadata literally means “data about data”. It can contains additional information about a file. This includes, but not limits to: title, author, creation date, etc. In this tutorial we show how to add or edit metadata of PDF documents using iText and Java.
Extensible Metdata Platform (XMP)
There are two types of metadata. The first is plain old key-value pairs. These are key-value pairs like: title, author, creation data, etc. The other is Extensible Metadata Platform (XMP). XMP is an ISO Standard and it’s an XML-based document. It’s standardizes a data model, a serialization format and core properties for the definition and processing of extensible metadata. It’s designed to be incorporated into digital documents without breaking their readability by applications that do not support XMP.
Add Meta data to PDF
We can directly add meta data to the document. It is important to notice that we add the metadata before we open the document. This is critical, because we cannot add metadata after the document is opened.
package com.memorynotfound.pdf.itext;
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.util.Locale;
public class AddMetaData {
public static void main(String... args) throws FileNotFoundException, DocumentException {
// create document and writer
Document document = new Document(PageSize.A4);
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream("meta-data.pdf"));
// add meta-data to pdf
document.addAuthor("Memorynotfound");
document.addCreationDate();
document.addCreator("Memorynotfound.com");
document.addTitle("Add meta data to PDF");
document.addSubject("how to add meta data to pdf using itext");
document.addKeywords("java, itext, pdf, meta-data");
document.addLanguage(Locale.ENGLISH.getLanguage());
document.addHeader("type", "tutorial, example");
// add xmp meta data
writer.createXmpMetadata();
document.open();
document.add(new Paragraph("Add meta-data to PDF using iText"));
document.close();
}
}
Edit Met data Existing PDF
The following example adds or edits the metadata of an existing PDF document. We start by reading the PDF document using the PdfReader
. The PdfStamper
will create a new PDF document. We can retrieve the metadata using the PdfReader#getInfo()
. To update this data we can simply add key value pairs.
package com.memorynotfound.pdf.itext;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.pdf.PdfReader;
import com.itextpdf.text.pdf.PdfStamper;
import com.itextpdf.text.xml.xmp.XmpWriter;
import java.io.ByteArrayOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.HashMap;
public class UpdateMetaData {
public static void main(String... args) throws IOException, DocumentException {
// read existing pdf document
PdfReader reader = new PdfReader("meta-data.pdf");
PdfStamper stamper = new PdfStamper(reader, new FileOutputStream("updated-meta-data.pdf"));
// get and edit meta-data
HashMap<String, String> info = reader.getInfo();
info.put("Subject", "Hello World");
info.put("Author", "your name");
info.put("Keywords", "iText pdf");
info.put("Title", "Hello World stamped");
info.put("Creator", "your name");
info.put("Producer", "sdfmlkqsdjflqsjf");
// add updated meta-data to pdf
stamper.setMoreInfo(info);
// update xmp meta-data
ByteArrayOutputStream baos = new ByteArrayOutputStream();
XmpWriter xmp = new XmpWriter(baos, info);
xmp.close();
stamper.setXmpMetadata(baos.toByteArray());
stamper.close();
}
}
Metadata Example
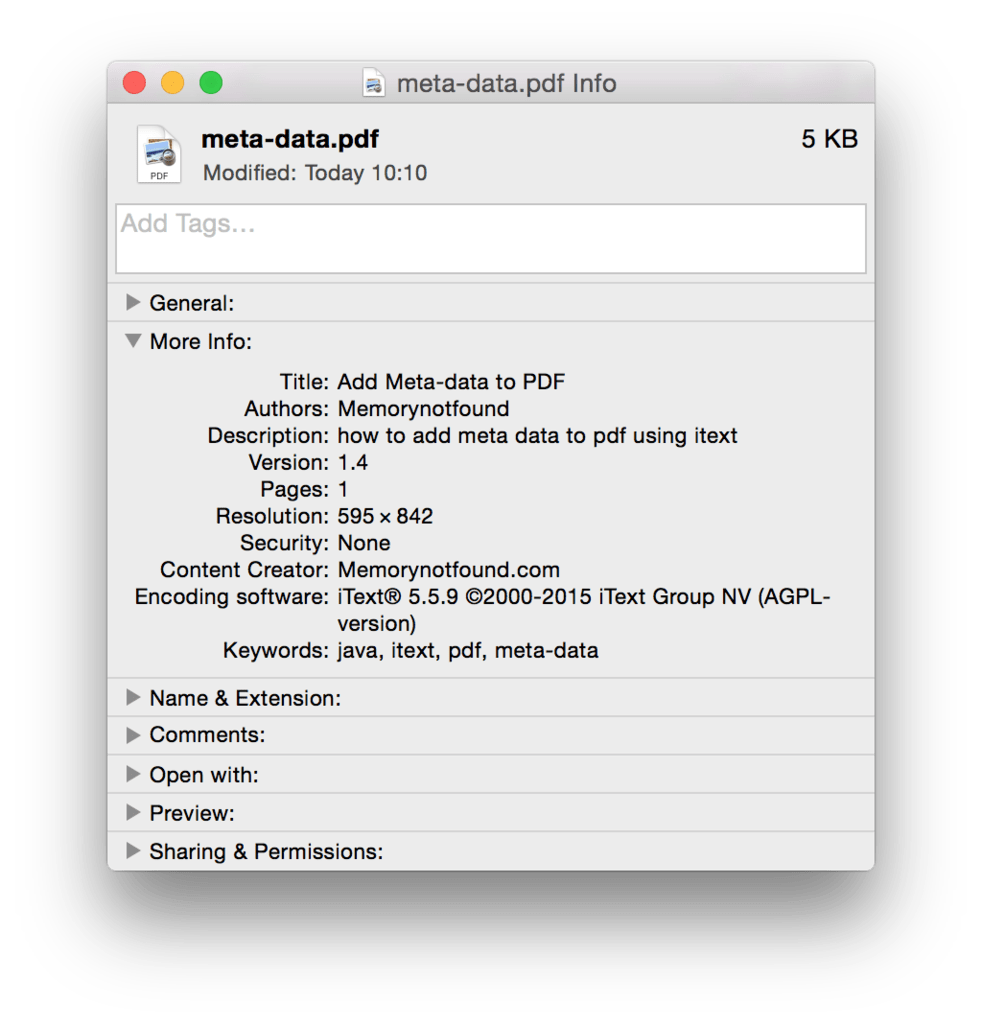
metadata properties pdf document