Configure Jersey with Annotations only
In this tutorial we show you how to Configure Jersey with Annotations. By using only Annotations to configure jersey, the web.xml file becomes obsolete. This results in cleaner code which is always a good thing. We need a class that extends the javax.ws.rs.core.Application
which will register the jersey application with the help of the @ApplicationPath
annotation.
Dependencies
Add jersey dependencies to your classpath.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.memorynotfound.webservice.rs.jersey</groupId>
<artifactId>no-webxml</artifactId>
<version>1.0.0-SNAPSHOT</version>
<packaging>war</packaging>
<name>JERSEY - ${project.artifactId}</name>
<url>https://memorynotfound.com</url>
<properties>
<jersey.version>2.20</jersey.version>
</properties>
<dependencies>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet</artifactId>
<version>${jersey.version}</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-json-jackson</artifactId>
<version>${jersey.version}</version>
</dependency>
</dependencies>
</project>
Simple Rest Service Example
This resource is mapped to the path courses and produces a json list of courses.
package com.memorynotfound.rs;
import javax.ws.rs.*;
import javax.ws.rs.core.MediaType;
import java.util.ArrayList;
import java.util.List;
@Path("/courses")
public class CourseRestService {
@GET
@Produces(MediaType.APPLICATION_JSON)
public List<Course> fetchAll() {
List<Course> courses = new ArrayList<Course>();
courses.add(new Course(1, "Configure Jersey with annotations"));
courses.add(new Course(2, "Configure Jersey without web.xml"));
return courses;
}
}
We use a simple POJO.
package com.memorynotfound.rs;
public class Course {
private Integer id;
private String name;
public Course(Integer id, String name) {
this.id = id;
this.name = name;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Configure Jersey with Annotations
We need to extend the javax.ws.rs.core.Application
class to configure jersey. We can manually register the rest services by overriding the getClasses()
method. But in this example we use a package scanner to automatically pickup rest services in our application. By overriding the getProperties()
metod and registering the property jersey.config.server.provider.packages
with the packages to search for rest services in our project, jersey is able to automatically register the rest services. Finally we need to map a context path that jersey must listen to. We can achieve this by annotating our class with the @ApplicationPath
annotation.
package com.memorynotfound.rs;
import java.util.HashMap;
import java.util.Map;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/api")
public class ApplicationConfig extends Application {
@Override
public Map<String, Object> getProperties() {
Map<String, Object> properties = new HashMap<String, Object>();
properties.put("jersey.config.server.provider.packages", "com.memorynotfound.rs");
return properties;
}
}
Demo
URL: http://localhost:8080/jersey-no-webxml/api/courses
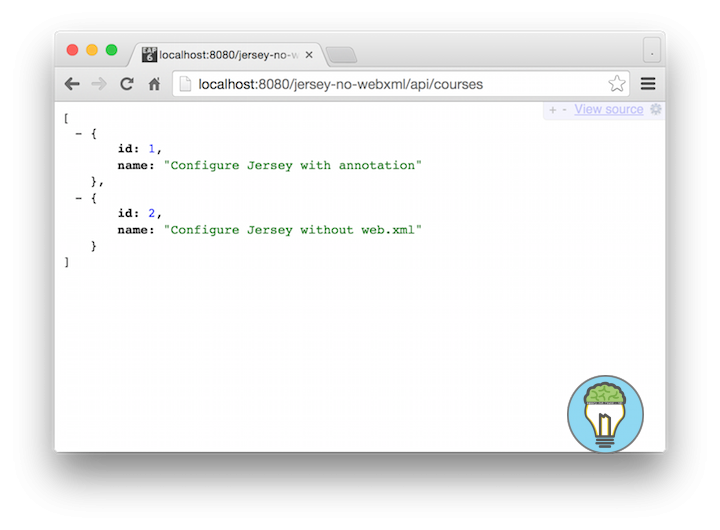