Display Custom JSF AJAX onerror event example
In this tutorial we will show you how to create a custom JSF AJAX onerror event. When the javax.faces.PROJECT_STAGE is set to Development you receive an popup every time an error occurs during an ajax processing. When the javax.faces.PROJECT_STAGE is set to Production you don’t receive anything. This is not what we want. If something goes wrong we want to inform the user about it. Let’s see how to create a custom onerror event for AJAX processing.
Processing Managed Bean
First let’s start with a managed bean that produces an error.
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class ProcessingBean {
public void processing() {
throw new RuntimeException("Error while processing data");
}
}
Display custom JSF AJAX onerror message
We can bind an onerror listener in 2 ways:
- By adding a function to the onerror attribute on the
<f:ajax>
element. This will only bind the function to that specific action. - Or you can add a global onerror listener using the
jsf.ajax.addOnError(displayErrorMessage)
method.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:f="http://xmlns.jcp.org/jsf/core">
<h:head>
<h:outputScript library="js" name="custom-script.js"/>
<h:outputScript library="javax.faces" name="jsf.js"/>
<script type="text/javascript">
//jsf.ajax.addOnError(displayErrorMessage);
</script>
</h:head>
<h:body>
<h2>JSF 2: onerror ajax</h2>
<h:form>
<pre id="error-messages"></pre>
<h:commandButton value="Submit" actionListener="#{processingBean.processing}">
<f:ajax render="@form" onerror="displayErrorMessage" />
</h:commandButton>
</h:form>
</h:body>
</html>
Display Error Message
We have a variable named data which contains the information about the response. In this example we simple display all the data on the page.
function displayErrorMessage(data) {
document.getElementById("error-messages").innerText =
"Error Description: " + data.description
+ "\nError Name: " + data.errorName
+ "\nError errorMessage: " + data.errorMessage
+ "\nResponse Code: " + data.responseCode
+ "\nResponse Text: " + data.responseText
+ "\nStatus: " + data.status
+ "\nType: " + data.type;
}
Demo
URL: http://localhost:8081/jsf-ajax-onerror/
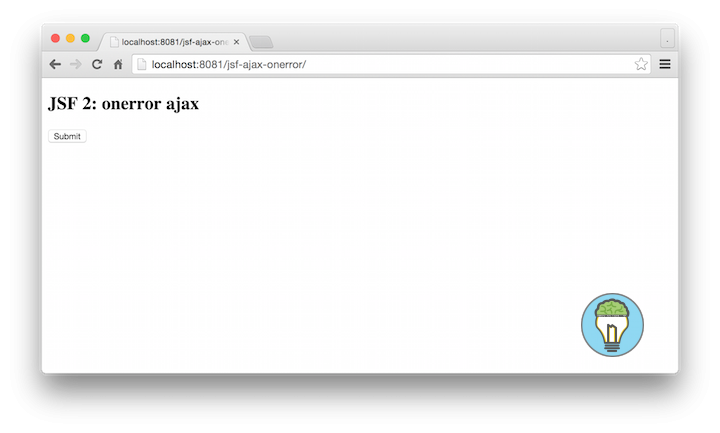
Here javax.faces.PROJECT_STAGE is set to Development.
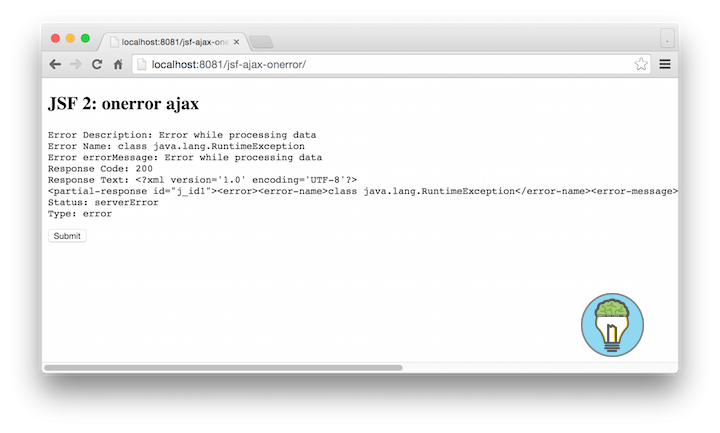
Here javax.faces.PROJECT_STAGE is set to Production.
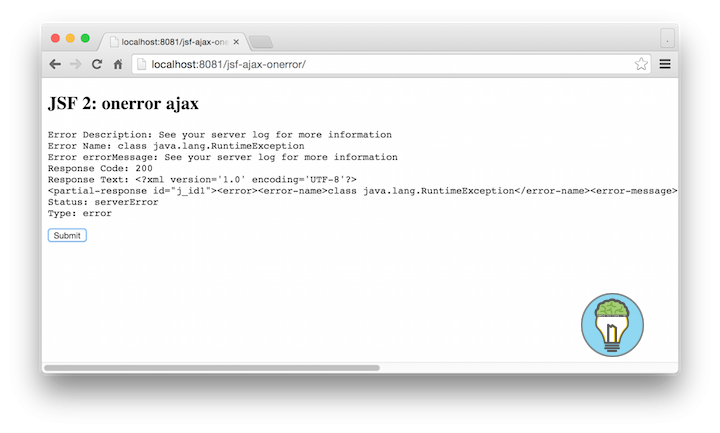