Deploy JAXWS application on Tomcat Example
In this tutorials we will show you how to deploy a JAXWS application on tomcat. You need to package your JAXWS Web Services as a war project. You can achieve this in maven by setting the packaging
to war
. Follow the following steps to deploy JAXWS application on tomcat.
Project Environment
- Java JDK 1.7
- JAX-WS 2.2.8
- Servlet Spec. 3.1.0
- Apache Tomcat 8.0.15
- Maven 3.2.3
Project Structure
src
|--main
| +--java
| +--com
| +--memorynotfound
| +--ws
| |--GreetingService.java
| |--GreetingServiceImpl.java
| +--resources
| +--webapp
| +--WEB-INF
| |--sun-jaxw.xml
| |--web.xml
pom.xml
Maven Dependencies
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.memorynotfound.webservice</groupId>
<artifactId>jax-ws-tomcat-example</artifactId>
<version>1.0.0-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<!-- jax-ws maven dependency -->
<dependency>
<groupId>com.sun.xml.ws</groupId>
<artifactId>jaxws-rt</artifactId>
<version>2.2.8</version>
</dependency>
<!-- servlet provided by tomcat -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
Greeting Service Interface
package com.memorynotfound.ws;
import javax.jws.WebMethod;
import javax.jws.WebService;
import javax.jws.soap.SOAPBinding;
import javax.jws.soap.SOAPBinding.Style;
@WebService
@SOAPBinding(style = Style.RPC)
public interface GreetingService {
@WebMethod
String printMessage();
}
Greeting Service Interface Implementation
package com.memorynotfound.ws;
import javax.jws.WebService;
@WebService
public class GreetingServiceImpl implements GreetingService {
public String printMessage() {
return "Hello, World!";
}
}
Add neccesary libraries to Tomcat
By default, Tomcat does not comes with any JAX-WS dependencies, So, you have to include it manually.
- Go here http://jax-ws.java.net/.
- Download JAX-WS RI distribution.
- Unzip it and copy following JAX-WS dependencies to Tomcat library folder “{$TOMCAT}/lib“.
- jaxb-impl.jar
- jaxws-api.jar
- jaxws-rt.jar
- gmbal-api-only.jar
- management-api.jar
- stax-ex.jar
- streambuffer.jar
- policy.jar
Servlet Descriptor web.xml
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<listener>
<listener-class>com.sun.xml.ws.transport.http.servlet.WSServletContextListener</listener-class>
</listener>
<session-config>
<session-timeout>120</session-timeout>
</session-config>
</web-app>
Deploy JAXWS application on Tomcat
You need to create a file called sun-jaxws.xml and put it in the ../WEB-INF/
folder.
<?xml version="1.0" encoding="UTF-8"?>
<endpoints xmlns="http://java.sun.com/xml/ns/jax-ws/ri/runtime" version="2.0">
<endpoint name="GreetingService" url-pattern="/greeting"
implementation="com.memorynotfound.ws.GreetingServiceImpl"/>
</endpoints>
Demo
URL: http://localhost:8080/jaxws-tomcat/greeting
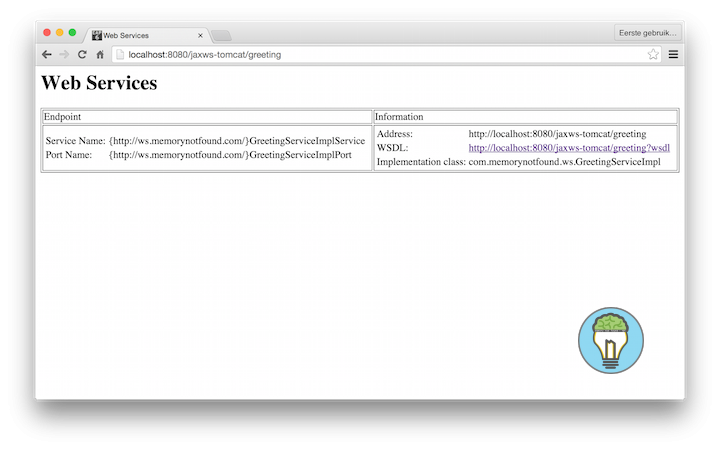
URL: http://localhost:8080/jaxws-tomcat/greeting?wsdl
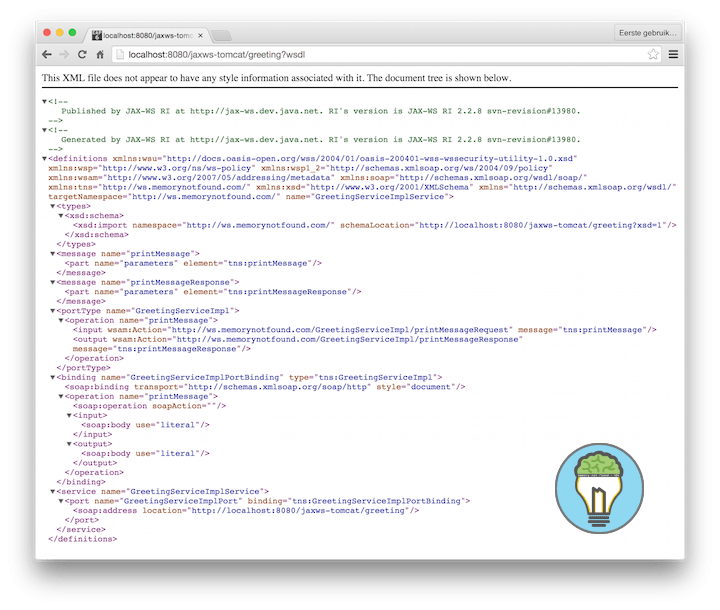