Disable Http Session in Java Web Application
In this example we will enforce the prevention of using Http Session in a Web Application. If you are working in a Team and you want to enforce your team to not using the Http Session. Then you can Disable Http Session in your applications for example: when building stateless rest services. There is no default setting in Java or your Web Container to prevent using sessions. But we can write a custom wrapper around our HttpServletRequest
that will throw an UnsupportedOperationException
every time a developer is trying to access the HttpSession
.
HttpRequestWrapper Wrapper: Disable Http Session
package com.memorynotfound;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletRequestWrapper;
import javax.servlet.http.HttpSession;
public class HttpServletDisableSessionRequestWrapper extends HttpServletRequestWrapper {
/**
* Constructs a request object wrapping the given request.
*
* @param request HttpServletRequest
* @throws IllegalArgumentException if the request is null
*/
public HttpServletDisableSessionRequestWrapper(HttpServletRequest request) {
super(request);
}
/**
* Disable Http Session
*/
@Override
public HttpSession getSession() {
throw new UnsupportedOperationException("HttpSession is not allowed");
}
/**
* Disable Http Session
*/
@Override
public HttpSession getSession(boolean create) {
throw new UnsupportedOperationException("HttpSession is not allowed");
}
}
Wrap HttpServletRequest With our Disable Http Session Wrapper
package com.memorynotfound;
import javax.servlet.*;
import javax.servlet.annotation.WebFilter;
import javax.servlet.http.HttpServletRequest;
import java.io.IOException;
@WebFilter(urlPatterns = {"/*"}, dispatcherTypes = DispatcherType.REQUEST, description = "will enforce to not use sessions")
public class DisableSessionFilter implements Filter {
@Override
public void init(FilterConfig filterConfig) throws ServletException {
}
@Override
public void doFilter(ServletRequest req, ServletResponse resp, FilterChain chain) throws IOException, ServletException {
HttpServletDisableSessionRequestWrapper wrapper = new HttpServletDisableSessionRequestWrapper((HttpServletRequest)req);
chain.doFilter(wrapper, resp);
}
@Override
public void destroy() {
}
}
Example Servlet Trying to Access the session
package com.memorynotfound;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
@WebServlet("/example")
public class SessionServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// Trying to grab the session
HttpSession session = req.getSession();
}
}
Demo
URL: localhost:8080/disable-httpsession/example
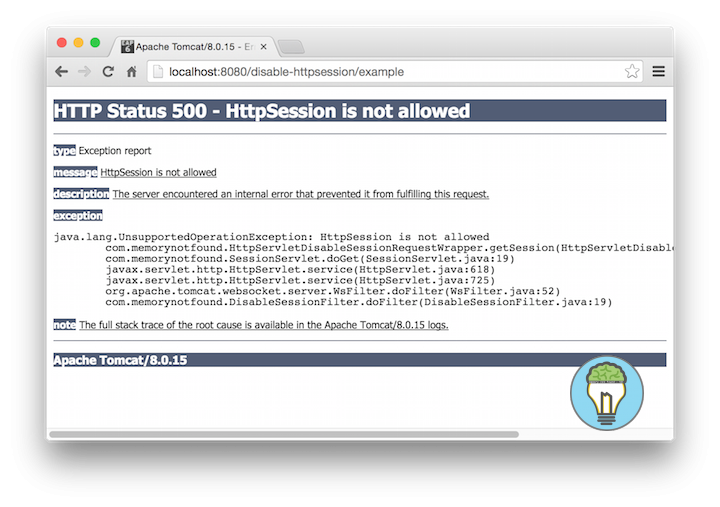