Encrypt Decrypt Password Protected PDF Documents iText
PDF documents are popular replacements for paper documents. They are standard, have the same look and feel on every platform, and typically aren’t something users can edit. As with paper documents, some PDFs are confidential. You can secure pdf documents with password protection. You can optionally specify the allowed permissions and encryption type. In this tutorial we show how to encrypt decrypt password protected PDF documents using iText.
Maven Dependencies
We use Apache Maven to manage our project dependencies. Add the following dependencies to your pom.xml
and Maven will manage these dependencies automatically. We use com.itextpdf.itext
and org.bouncycastle.bcprov-jdk15on
. The bouncycastle dependency is used for digital encryption support.
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.9</version>
</dependency>
<dependency>
<groupId>org.bouncycastle</groupId>
<artifactId>bcprov-jdk15on</artifactId>
<version>1.54</version>
</dependency>
Protect PDF document with password
After we created a PdfWriter
we can optionally set an encryption. In this example we use password encryption. The first and second arguments are the user and owner passwords, respectively.
- User Password: also referred as the “open password“. This password is typically shared with the users who may read the PDF document.
- Owner Password: also referred as the “permissions password“. You need this password in order to change the content or permissions of this document.
The third argument sets the permissions for the PDF document. Finally, the last argument is used to set the encryption type. We explain which permissions and encryptions you can use in the following section.
String USER_PASSWORD = "user";
String OWNER_PASSWORD = "owner";
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream("password-protected.pdf"));
writer.setEncryption(
USER_PASSWORD.getBytes(),
OWNER_PASSWORD.getBytes(),
PdfWriter.ALLOW_COPY | PdfWriter.ALLOW_PRINTING,
PdfWriter.ENCRYPTION_AES_256 | PdfWriter.DO_NOT_ENCRYPT_METADATA);
- When a user opens this document with the user password, he’s only able to perform the actions, specified in the permissions parameter. In this case, the user is able to print and copy the page.
- If the user opens the document with the owner password, he’s able to change the permissions.
- When you don’t specify a user password, all users will be able to open the document without being prompted for a password, but the permissions and restrictions – if any – will remain in place.
PDF Permissions
In the third argument of the setEncryption()
method, we specify the allowed permissions. When 0
is passed as a parameter, the user is only allowed to view the document. Otherwise, you can use any of the following permissions.
PdfWriter.ALLOW_PRINTING
PdfWriter.ALLOW_MODIFY_CONTENTS
PdfWriter.ALLOW_COPY
PdfWriter.ALLOW_MODIFY_ANNOTATIONS
PdfWriter.ALLOW_FILL_IN
PdfWriter.ALLOW_SCREENREADERS
PdfWriter.ALLOW_ASSEMBLY
PdfWriter.ALLOW_DEGRADED_PRINTING
You can optionally compose multiple permissions using the bitwise ORing operator.
PdfWriter.ALLOW_COPY | PdfWriter.ALLOW_PRINTING
PDF Encryption
The last parameter is for the encryption type. Here are the encryption type options you can use.
PdfWriter.STANDARD_ENCRYPTION_40
PdfWriter.STANDARD_ENCRYPTION_128
PdfWriter.ENCRYPTION_AES_128
PdfWriter.ENCRYPTION_AES_256
You can optionally add the PdfWriter.DO_NOT_ENCRYPT_METADATA
option, using the bitwise ORing operator. When this option is specified, iText will not encrypt the meta-data.
Encrypt PDF document iText
The following example creates an encrypted password protected PDF document.
package com.memorynotfound.pdf.itext;
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
public class EncryptPdfDocument {
static String user = "user";
static String owner = "owner";
static String destination = "password-protected.pdf";
public static void main(String... args) throws FileNotFoundException, DocumentException {
Document document = new Document(PageSize.A4);
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream(destination));
// secure pdf with password protection
writer.setEncryption(
user.getBytes(),
owner.getBytes(),
PdfWriter.ALLOW_COPY | PdfWriter.ALLOW_PRINTING,
PdfWriter.ENCRYPTION_AES_256 | PdfWriter.DO_NOT_ENCRYPT_METADATA);
document.open();
document.add(new Paragraph("Secure Pdf with Password using iText"));
document.close();
}
}
Decrypt Pdf Document iText
The following example decrypts an encrypted password protected PDF document.
package com.memorynotfound.pdf.itext;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.pdf.PdfReader;
import com.itextpdf.text.pdf.PdfStamper;
import java.io.FileOutputStream;
import java.io.IOException;
public class DecryptPdfDocument {
static String owner = "owner";
static String original = "password-protected.pdf";
static String destination = "decrypted-password-protected.pdf";
public static void main(String... args) throws IOException, DocumentException {
PdfReader reader = new PdfReader(original, owner.getBytes());
PdfStamper stamper = new PdfStamper(reader, new FileOutputStream(destination));
stamper.close();
}
}
Output
When we look at the properties of the document, we see that the document is encrypted using password protection.
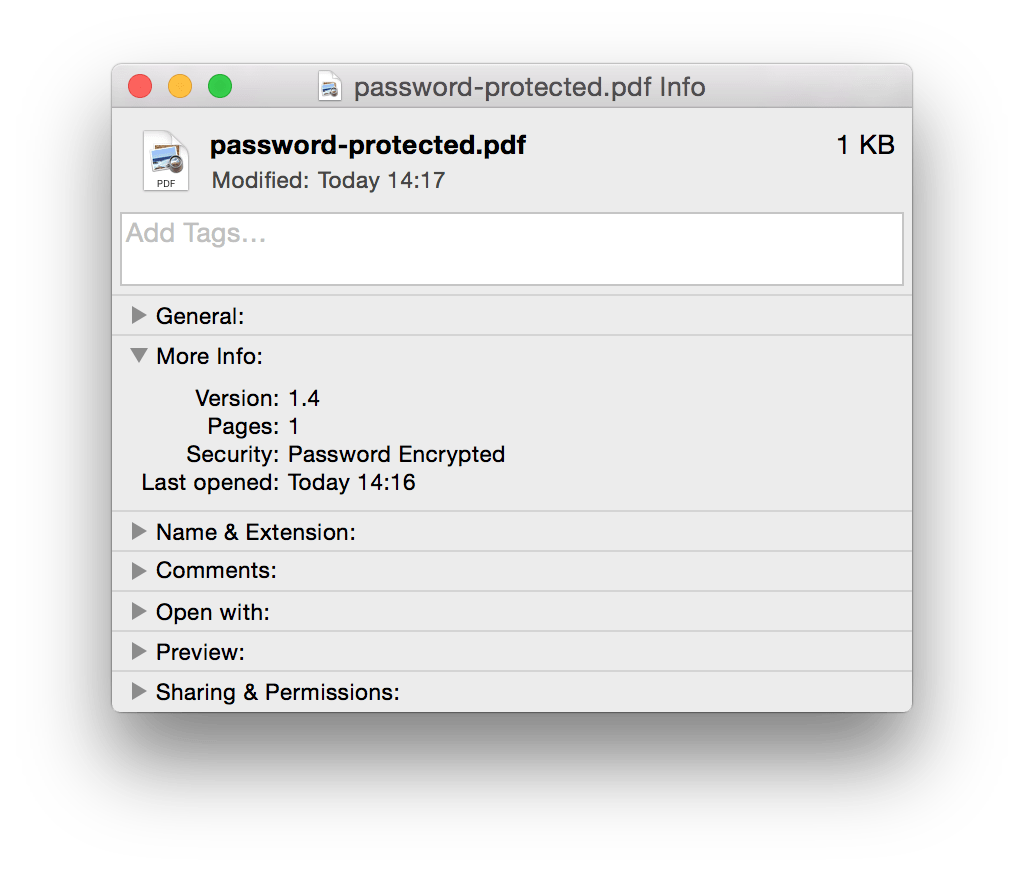
We can open the document when we enter either the user or owner password: