Java 7z Seven Zip Example – compress and decompress a file
In this tutorial we demonstrate how to compress files to 7z format recursively and decompress 7z files. 7-Zip also known as 7z is a compressed archive file format that supports several different data compression, encryption and pre-processing algorithms.
Project Structure
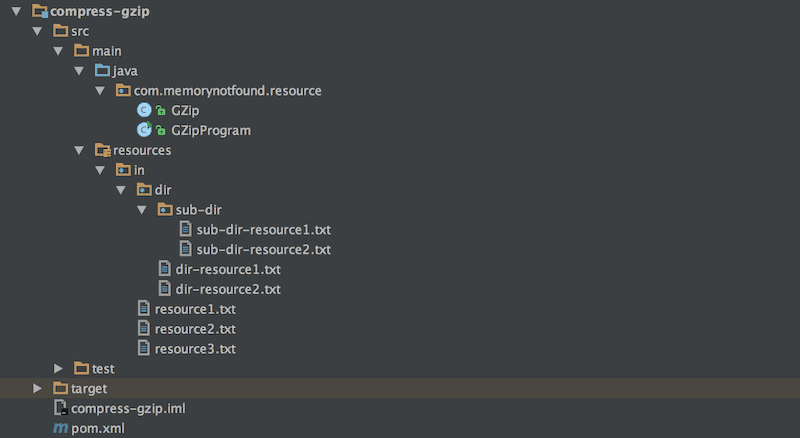
Maven Dependencies
We use Apache Maven to manage our project dependencies. Make sure the following dependencies reside on the class-path.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.memorynotfound.io.compression</groupId>
<artifactId>7z</artifactId>
<version>1.0.0-SNAPSHOT</version>
<name>IO Compression - ${project.artifactId}</name>
<url>https://memorynotfound.com</url>
<packaging>jar</packaging>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-compress</artifactId>
<version>1.14</version>
</dependency>
<dependency>
<groupId>org.tukaani</groupId>
<artifactId>xz</artifactId>
<version>1.6</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.7.0</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
</project>
Compress and Decompress 7z files Recursively
- Compressing files in 7z format: We use the
SevenZOutputFile
to compress files and/or directories into 7z format. We can add entries in the archive using theSevenZArchiveEntry
. - Decompressing 7z archive: We can read the 7z archive using the
SevenZFile
class. Next, we loop over theSevenZArchiveEntry
using theSevenZArchiveEntry.getNextEntry()
class and write the content to anFileOutputStream
.
package com.memorynotfound.resource;
import org.apache.commons.compress.archivers.sevenz.SevenZArchiveEntry;
import org.apache.commons.compress.archivers.sevenz.SevenZFile;
import org.apache.commons.compress.archivers.sevenz.SevenZOutputFile;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class SevenZ {
private SevenZ() {
}
public static void compress(String name, File... files) throws IOException {
try (SevenZOutputFile out = new SevenZOutputFile(new File(name))){
for (File file : files){
addToArchiveCompression(out, file, ".");
}
}
}
public static void decompress(String in, File destination) throws IOException {
SevenZFile sevenZFile = new SevenZFile(new File(in));
SevenZArchiveEntry entry;
while ((entry = sevenZFile.getNextEntry()) != null){
if (entry.isDirectory()){
continue;
}
File curfile = new File(destination, entry.getName());
File parent = curfile.getParentFile();
if (!parent.exists()) {
parent.mkdirs();
}
FileOutputStream out = new FileOutputStream(curfile);
byte[] content = new byte[(int) entry.getSize()];
sevenZFile.read(content, 0, content.length);
out.write(content);
out.close();
}
}
private static void addToArchiveCompression(SevenZOutputFile out, File file, String dir) throws IOException {
String name = dir + File.separator + file.getName();
if (file.isFile()){
SevenZArchiveEntry entry = out.createArchiveEntry(file, name);
out.putArchiveEntry(entry);
FileInputStream in = new FileInputStream(file);
byte[] b = new byte[1024];
int count = 0;
while ((count = in.read(b)) > 0) {
out.write(b, 0, count);
}
out.closeArchiveEntry();
} else if (file.isDirectory()) {
File[] children = file.listFiles();
if (children != null){
for (File child : children){
addToArchiveCompression(out, child, name);
}
}
} else {
System.out.println(file.getName() + " is not supported");
}
}
}
Java Zz Example
This program demonstrates the 7z archive compression decompression example.
package com.memorynotfound.resource;
import java.io.File;
import java.io.IOException;
public class SevenZProgram {
private static final String OUTPUT_DIRECTORY = "/tmp";
private static final String TAR_GZIP_SUFFIX = ".7z";
private static final String MULTIPLE_RESOURCES = "/example-multiple-resources";
private static final String RECURSIVE_DIRECTORY = "/example-recursive-directory";
private static final String MULTIPLE_RESOURCES_PATH = OUTPUT_DIRECTORY + MULTIPLE_RESOURCES + TAR_GZIP_SUFFIX;
private static final String RECURSIVE_DIRECTORY_PATH = OUTPUT_DIRECTORY + RECURSIVE_DIRECTORY + TAR_GZIP_SUFFIX;
public static void main(String... args) throws IOException {
// class for resource classloading
Class clazz = SevenZProgram.class;
// get multiple resources files to compress
File resource1 = new File(clazz.getResource("/in/resource1.txt").getFile());
File resource2 = new File(clazz.getResource("/in/resource2.txt").getFile());
File resource3 = new File(clazz.getResource("/in/resource3.txt").getFile());
// compress multiple resources
SevenZ.compress(MULTIPLE_RESOURCES_PATH, resource1, resource2, resource3);
// decompress multiple resources
SevenZ.decompress(MULTIPLE_RESOURCES_PATH, new File(OUTPUT_DIRECTORY + MULTIPLE_RESOURCES));
// get directory file to compress
File directory = new File(clazz.getResource("/in/dir").getFile());
// compress recursive directory
SevenZ.compress(RECURSIVE_DIRECTORY_PATH, directory);
// decompress recursive directory
SevenZ.decompress(RECURSIVE_DIRECTORY_PATH, new File(OUTPUT_DIRECTORY + RECURSIVE_DIRECTORY));
}
}
Generated Files
Here is an example of the generated 7z archive with multiple files.

Here is an example of the generated 7z archive with recursive directory.
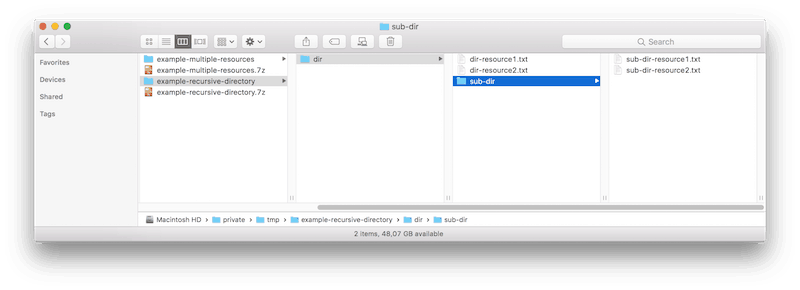
References
- Apache Commons Compress Official Website
- Apache Commons Compress API JavaDoc
- SevenZOutputFile JavaDoc
- SevenZArchiveEntry JavaDoc
- SevenZFile JavaDoc
Hi,
I want to use AES-256 encryption algorithm and using a password protected 7zip file.Zip file can be decrypted via 7zip application). I want to use the same library that you have used. Could you please help me for the solution?
Thanks,
Regards.