JAX-RS Rest Service Example with Jersey in Java
In this tutorial we will show you the basic configuration needed to get a RESTful web service up and running. Get the required dependencies, create and expose methods and finally configure the servlet to the right path. Common name for mapping your servlet is /api/*. That’s it!
Project Structure
src
|--main
| +--java
| +--com
| +--memorynotfound
| +--rs
| |--HealthCheck.java
| +--resources
| +--webapp
| +--WEB-INF
| |--web.xml
pom.xml
Jersey Dependencies
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.memorynotfound.webservice</groupId>
<artifactId>jax-rs-jersey-example</artifactId>
<version>1.0.0-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-servlet</artifactId>
<version>1.18.3</version>
</dependency>
</dependencies>
</project>
Note: If you want to use jersey version 2 you need to replace the jersey-servlet dependency with the following jersey-container-servlet dependency.
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet</artifactId>
<version>2.14</version>
</dependency>
JAX-RS Rest Service
package com.memorynotfound.rs;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
@Path("/healthcheck")
public class HealthCheck {
@GET
public String getHealth() {
return "alive and kicking";
}
}
Jersey Configuration web.xml
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<servlet>
<servlet-name>Jersey-Servlet</servlet-name>
<servlet-class>com.sun.jersey.spi.container.servlet.ServletContainer</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>Jersey-Servlet</servlet-name>
<url-pattern>/api/*</url-pattern>
</servlet-mapping>
</web-app>
Note: If you want to use jersey version 2 you need to replace the servlet definition with the following:
<servlet>
<servlet-name>Jersey-Servlet</servlet-name>
<servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>jersey.config.server.provider.packages</param-name>
<param-value>com.memorynotfound.rs</param-value>
</init-param>
</servlet>
Testing
You can test the JAX-RS REST Service using URL: http://localhost:8080/jersey/api/healthcheck
- The jersey part is the path where our application is deployed
- The api part is the path where our jersey servlet is mapped
- The healthcheck part is the path where our health check rest service is deployed
Executing the RESTfull web service using curl
$ curl http://localhost:8080/jersey/api/healthcheck
alive and kicking
Inspecting the headers
$ curl -I http://localhost:8080/jersey/api/healthcheck
HTTP/1.1 200 OK
Server: Apache-Coyote/1.1
Content-Type: text/html
Content-Length: 0
Date: Thu, 11 Dec 2014 16:49:41 GMT
Demo
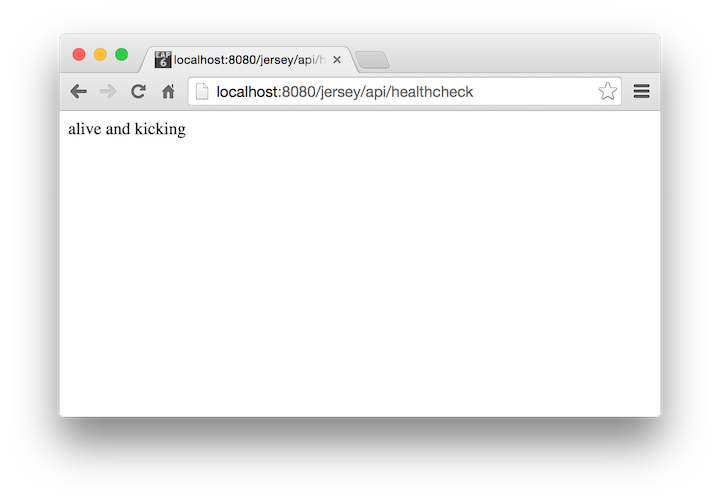
For more information on this topic you can read more on the following hyperlinks.