JAX-RS @FormParam Example
We can post an HTML form directly to our JAX-RS service, we can read the request bodies by mapping the fields using the @FormParam
annotation. Lets show how this works by a simple example.
We have the following form which we want to register a new course. We register the CourseResource
URI in the action attribute of the form.
<!DOCTYPE html>
<html>
<body>
<h1>JAX-RS @FormParam Example</h1>
<form action="api/courses" method="post">
<label for="name">Name: </label>
<input id="name" type="text" name="name" />
<label for="price">Price: </label>
<input id="price" type="text" name="price" />
<input type="submit" value="Submit" />
</form>
</body>
</html>
Mapping JAX-RS @FormParam
We can map the incoming request body parameters using the @FormParam
annotation. Make sure the name of the request parameter maps to the same name registered in the @FormParam
annotation. Form data is URL-encoded when it goes across the wire. When using @FormParam
, JAX-RS will automatically decode the form entry’s value before injecting it.
package com.memorynotfound.rs;
import javax.ws.rs.Consumes;
import javax.ws.rs.FormParam;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.core.MediaType;
@Path("/courses")
public class CourseResource {
@POST
@Consumes(MediaType.APPLICATION_FORM_URLENCODED)
public void createCustomer(@FormParam("name") String name,
@FormParam("price") Double price){
System.out.println("creating course");
System.out.println("name: " + name);
System.out.println("email: " + price);
}
}
Sending Form Parameters with JAX-RS Client API
You can use the Form
class to send form parameters using the JAX-RS client. This class automatically adds the application/x-www-form-urlencoded in the accept HTTP Request Headers. Here is an example how to use it.
package com.memorynotfound.rs;
import javax.ws.rs.client.Client;
import javax.ws.rs.client.ClientBuilder;
import javax.ws.rs.client.Entity;
import javax.ws.rs.core.Form;
import javax.ws.rs.core.Response;
public class RunClient {
public static void main(String... args){
Client client = ClientBuilder.newClient();
Form form = new Form()
.param("name", "JAX-RS @FormParam Example")
.param("price", "500");
Response response = client
.target("http://localhost:8081/jaxrs-form-param/api/courses")
.request()
.post(Entity.form(form));
if (response.getStatus() == 200){
System.out.println("Response: " + response.readEntity(String.class));
}
client.close();
}
}
Demo
URL: http://localhost:8081/jaxrs-form-param/
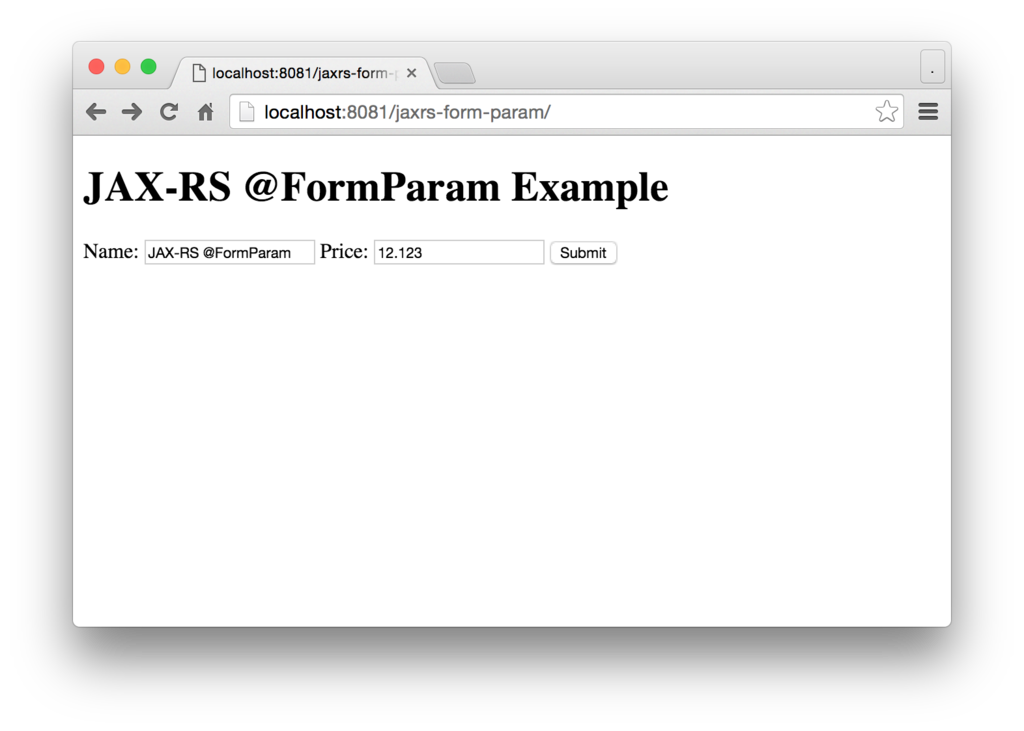
When submitting the form, the rest service produces the following output:
creating course
name: JAX-RS @FormParam
email: 12.123