JSF 2.2 Basic Ajax Feature Example explained
Project Environment
- Java JDK 1.7
- JSF 2.2.8-02
- Servlet Spec. 3.1.0
- Apache Tomcat 8.0.15
- Maven 3.2.3
JSF 2.2 AJAX View
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core">
<h:head>
<h:outputScript library="javax.faces" name="jsf.js" target="body" />
</h:head>
<h:body>
<h1>JSF 2.x basic ajax example</h1>
<h:form>
<h:outputLabel value="Show a message using ajax: ">
<h:inputText id="message" value="#{messageBean.message}"/>
</h:outputLabel>
<h:commandButton value="Show Message">
<f:ajax execute="message" render="output" />
</h:commandButton>
</h:form>
<p><h:outputText id="output" value="#{messageBean.message}" /></p>
</h:body>
</html>
In this example we use ajax to submit the form to the server and re-render only a port of the screen. In this case only output will be re-rendered.
<h:commandButton value="Show Message">
<f:ajax execute="message" render="output" />
</h:commandButton>
The execute attribute of the <f:ajax/> element indicates that only the elements specified here are submitted to the server. In our case only “message” will be submitted to the server for processing.
<h:outputText id="output" value="#{messageBean.message}" />
The render attribute of the <f:ajax/> element will refresh the component with an id of “output” if the ajax request is finished.Managed Bean
We have a simple managed bean which holds a message to be displayed on the page.
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class MessageBean {
private String message;
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
}
Demo
URL : http://localhost:8080/jsf-basic/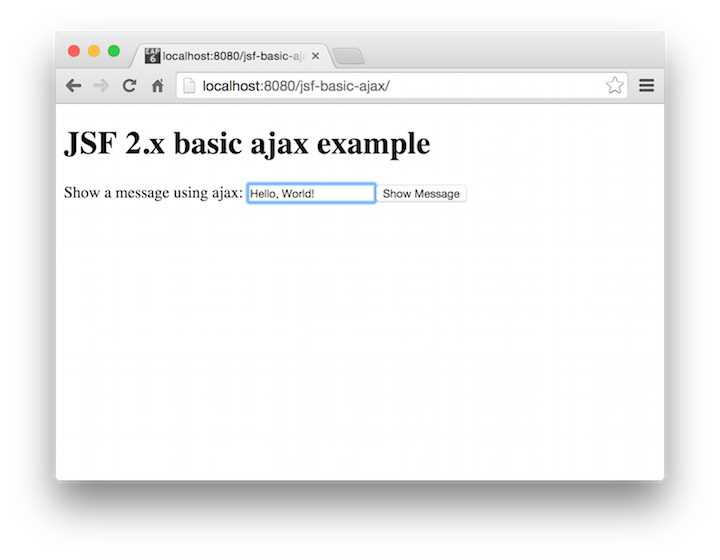
We submit a value to the server using the command button which is configured with the <f:ajax/> tag. This will send a partial form submission to the server for processing.
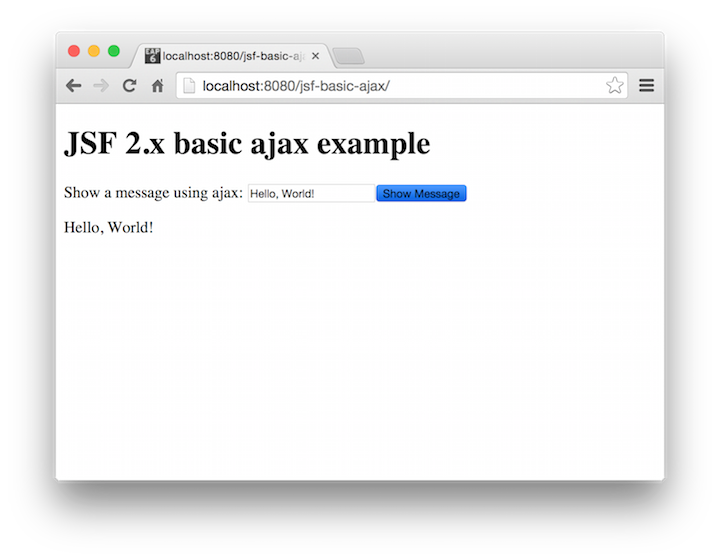
When the user presses the “Show Message” button the form is submitted to the server and the “output” is refreshed with the submitted value.
Hi this line will give an exception:
Exception :
javax.faces.FacesException: contains an unknown id ‘output’
– cannot locate it in the context of the component j_idt8
Solution:
in JSF 2.0 and above tag required the “render” output within the same form level, so you should put inside the scope of