JSF 2 Project Configuration and Dependencies
JavaServer Faces (JSF) is a Java specification for building component-based user interfaces for web applications. In this tutorial, we will show you how to develop and configure a JavaServer Faces example project. Some key differences of JSF 2 over JSF 1 is the configuration with annotations. This means less XML configuration witch is a huge improvement.
Project Environment
- Java JDK 1.7
- JSF 2.2.13
- Servlet Spec. 3.1.0
- Apache Tomcat 8.0.15
- Maven 3.2.3
JSF 2.x basic example
Maven Configuration
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.memorynotfound</groupId>
<artifactId>basic-example</artifactId>
<version>1.0.0-SNAPSHOT</version>
<name>UI - JSF basic example</name>
<url>https://memorynotfound.com</url>
<packaging>war</packaging>
<dependencies>
<!-- JSF for java ee applications -->
<dependency>
<groupId>javax.faces</groupId>
<artifactId>jsf-api</artifactId>
<version>2.1</version>
</dependency>
<!-- JSP Standard Tag Libraries -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- servlet provided by tomcat -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
<!-- JSF for tomcat -->
<dependency>
<groupId>com.sun.faces</groupId>
<artifactId>jsf-api</artifactId>
<version>2.2.13</version>
</dependency>
<dependency>
<groupId>com.sun.faces</groupId>
<artifactId>jsf-impl</artifactId>
<version>2.2.13</version>
</dependency>
Managed Bean
A managed bean is a regular java bean with a fancy name. When we register the bean with JSF with the @Managedbean annotation or the XML configuration in the faces-config.xml the bean becomes managed by JSF. In JSF, managed beans are used as model for UI components.
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class HelloBean {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Servlet Configuration
<web-app xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
version="3.0">
<!-- JSF project stage set to production when going live -->
<context-param>
<param-name>javax.faces.PROJECT_STAGE</param-name>
<param-value>Development</param-value>
</context-param>
<!-- Welcome page -->
<welcome-file-list>
<welcome-file>index.xhtml</welcome-file>
</welcome-file-list>
<!-- JSF mapping -->
<servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<!-- Mappings for JSF FacesServlet -->
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
</web-app>
You need to register a javax.faces.webapp.FacesServlet with the appropriate mapping. For simplicity I only register one servlet mapping to *.xhtml. Witch corresponds to http://localhost:8080/jsf-basic-example/<page>.html.
JSF Views
Index.xhtml<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core">
<h:body>
<h2>JSF 2.x hello world example</h2>
<h:form>
<h:outputLabel value="Favorite beer:">
<h:inputText value="#{helloBean.name}"/>
</h:outputLabel>
<h:commandButton value="submit" action="result"/>
</h:form>
</h:body>
</html>
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core">
<h:body>
<h2>JSF 2.x hello world example</h2>
<p>hello, #{helloBean.name}!</p>
</h:body>
</html>
Demo
URL : http://localhost:8080/jsf-example/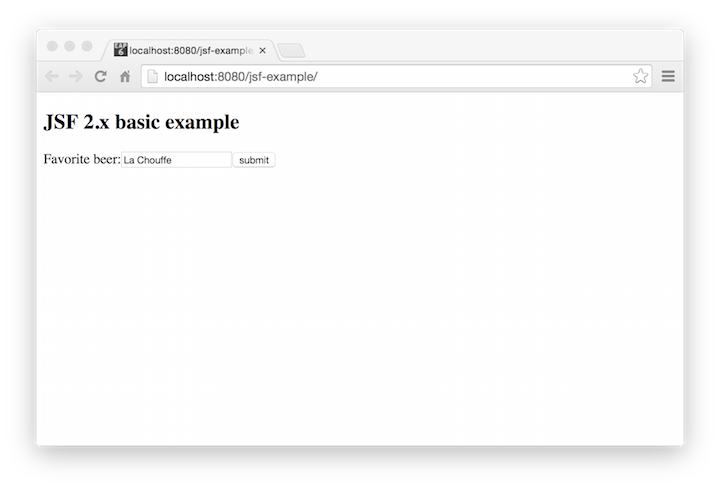
Sample application that asks the user witch is his favorite beer.
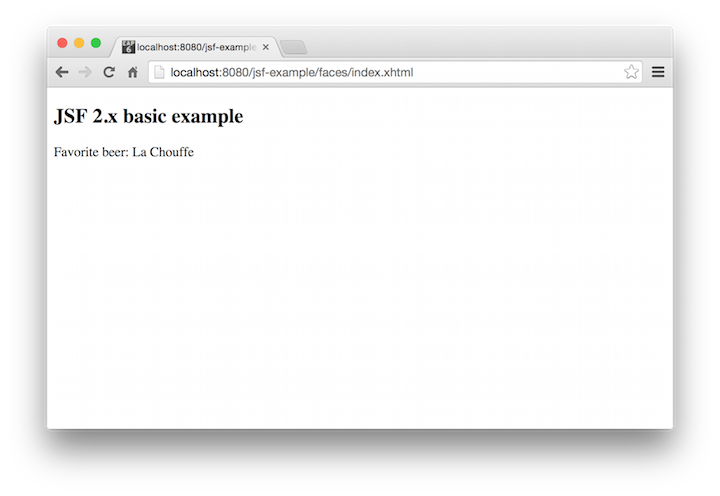
When the user submits the form, the result page is called witch prints out the submitted input value.