JSF 2: Custom Output FacesComponent Example
In this tutorial we will show you how to build a custom output component, also known as a FacesComponent. We’ll only look how to create a custom component using annotations. If you’re looking for prior JSF 2 you can read the creating custom components in jsf.
Creating the custom component AKA FacesComponent
We can create a custom component and automatically create the tagfile. We do this by annotating the class with @FacesComponent
and setting the creatTag attribute to true. You’ll also need to give the tag a name, namespace and value.
We are creating a simple custom output component so we can extend from the UIComponentBase
class, so we can override the encodeAll()
method. This allows us to get the response writer and write necessary attributes to our component.
package com.memorynotfound.jsf;
import javax.faces.component.FacesComponent;
import javax.faces.component.UIComponentBase;
import javax.faces.context.FacesContext;
import javax.faces.context.ResponseWriter;
import java.io.IOException;
@FacesComponent(createTag = true,
tagName = "custom-output",
namespace = "https://memorynotfound.com/example",
value = CustomOutput.FAMILY)
public class CustomOutput extends UIComponentBase {
protected static final String FAMILY = "com.memorynotfound.jsf.CustomOutput";
@Override
public void encodeAll(FacesContext context) throws IOException {
ResponseWriter writer = context.getResponseWriter();
writer.startElement("div", this);
writer.writeAttribute("id", getClientId(context), null);
writer.writeAttribute("style", "color : red", null);
String message = (String) this.getAttributes().get("greeting");
if (message == null){
writer.writeText("HelloWorld! today is: " + new java.util.Date(), null);
} else {
writer.writeText(message, null);
}
writer.endElement("div");
}
@Override
public String getFamily() {
return CustomOutput.FAMILY;
}
}
Displaying the custom output component
We can simply use our component by adding the namespace and using the custom component in our page.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:custom="https://memorynotfound.com/example">
<h:body>
<h2>JSF 2: Custom Output Component</h2>
<custom:custom-output greeting="Hello, World!"/>
</h:body>
</html>
Demo
URL: http://localhost:8081/jsf-custom-output-component/
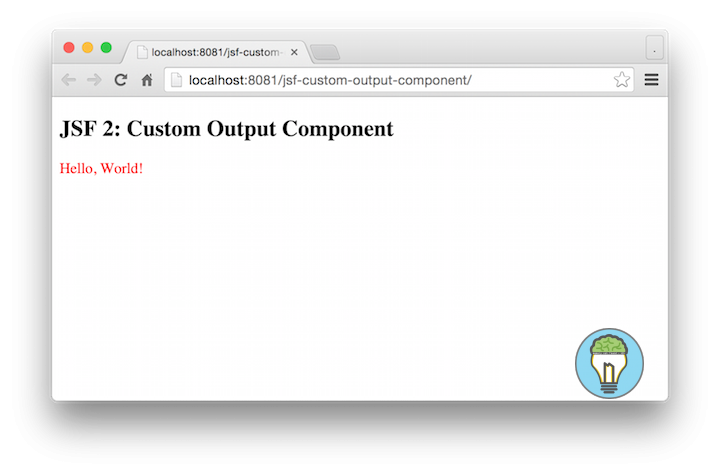