JSF 2 HTML5 placeholder and passThroughAttribute example
In this tutorial we will show you the new JSF 2 HTML5 placeholder and passThroughAttribute. JSF 2.2 introduces pass through attributes to support new and modified attributes defined in HTML5. Components don’t process pass through attributes. These attributes are directly passed to the rendered output element.
Managed Bean
This managed bean defines a couple of email addresses to work with on the page. Note that it also has a getAttributes()
method. We are using these attributes to pass through an element.
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import java.util.HashMap;
import java.util.Map;
@ManagedBean
@RequestScoped
public class UserBean {
private String email1, email2, email3, email4;
public Map<String, Object> getAttributes(){
Map<String, Object> attributes = new HashMap<String, Object>();
attributes.put("type", "email");
attributes.put("placeholder", "Enter email");
return attributes;
}
public String getEmail1() {
return email1;
}
public void setEmail1(String email1) {
this.email1 = email1;
}
public String getEmail2() {
return email2;
}
public void setEmail2(String email2) {
this.email2 = email2;
}
public String getEmail3() {
return email3;
}
public void setEmail3(String email3) {
this.email3 = email3;
}
public String getEmail4() {
return email4;
}
public void setEmail4(String email4) {
this.email4 = email4;
}
}
HTML5 placeholder and passThroughAttribute
- by passthrough attribute inside element
- by passthrough as inner element
- by passtrhrough with attributes from managed bean
- by enhancing html with jsf
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:p="http://xmlns.jcp.org/jsf/passthrough"
xmlns:jsf="http://xmlns.jcp.org/jsf"
xmlns:f="http://xmlns.jcp.org/jsf/core">
<head jsf:id="head">
<link jsf:rel="stylesheet" jsf:href="style.css" jsf:name="style.css" />
</head>
<h:body>
<h2>JSF 2: HTML5 placeholder</h2>
<h:form id="form">
<p>by passthrough attribute inside element</p>
<h:inputText id="email1" value="#{userBean.email1}" p:type="email" p:placeholder="Enter email"/>
<p>by passthrough as inner element</p>
<h:inputText id="email2" value="#{userBean.email2}">
<f:passThroughAttribute name="type" value="email"/>
<f:passThroughAttribute name="placeholder" value="Enter email"/>
</h:inputText>
<p>by passtrhrough with attributes from managed bean</p>
<h:inputText id="email3" value="#{userBean.email3}">
<f:passThroughAttributes value="#{userBean.attributes}"/>
</h:inputText>
<p>by enhancing html with jsf</p>
<input type="email" jsf:id="email4" placeholder="Enter email" jsf:value="#{userBean.email4}">
<f:validateLength minimum="5"/>
</input>
<p>action buttons</p>
<h:panelGroup>
<h:commandButton value="Save by jsf"/>
<input jsf:id="save" type="submit" value="Save by html"/>
</h:panelGroup>
</h:form>
</h:body>
</html>
Generated Output
All the previous elements are rendered the same.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="head">
<link type="text/css" rel="stylesheet" href="/jsf-html5-input-placeholder/javax.faces.resource/style.css.xhtml" />
</head>
<body>
<h2>JSF 2: HTML5 placeholder</h2>
<form id="form" name="form" ...>
...
<p>by passthrough attribute inside element</p>
<input id="form:email1" name="form:email1" value="" placeholder="Enter email" type="email" />
<p>by passthrough as inner element</p>
<input id="form:email2" name="form:email2" value="" placeholder="Enter email" type="email" />
<p>by passtrhrough with attributes from managed bean</p>
<input id="form:email3" name="form:email3" value="" placeholder="Enter email" type="email" />
<p>by enhancing html with jsf</p>
<input id="form:email4" name="form:email4" value="" placeholder="Enter email" type="email" />
<p>action buttons</p>
<input id="form:save-command-button" type="submit" name="form:save-command-button" value="Save by jsf" />
<input id="form:save" name="form:save" type="submit" value="Save by html" />
...
</form>
</body>
</html>
Demo
URL: http://localhost:8081/jsf-html5-input-placeholder/index.xhtml
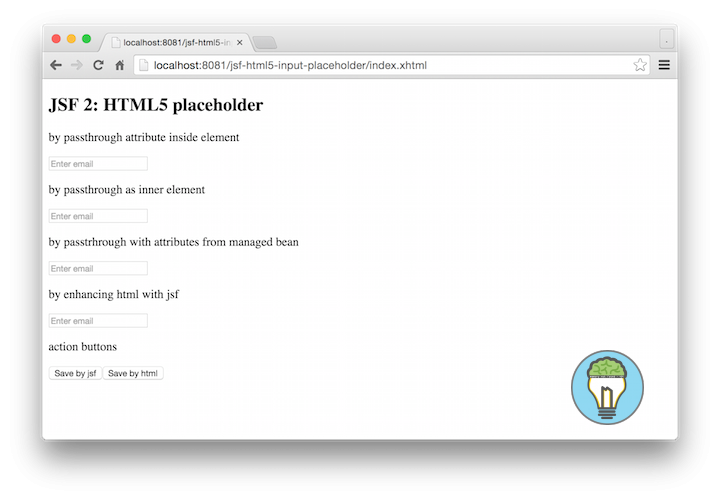