JSF add custom ajax event example
In this tutorial we will bind a JSF custom ajax event to a managed bean method. This example will fire an event on keyup event. When the user is typing the data is sent to the managed bean which is sent back via AJAX.
Processing Managed Bean
The processing method will transform the name into uppercase.
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import javax.faces.event.AjaxBehaviorEvent;
@ManagedBean
@RequestScoped
public class ProcessingBean {
private String name;
public void processing(){
name = name.toUpperCase();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
JSF add custom ajax event
We bind a keyup event to the <f:ajax>
element via the event attribute. This will fire the event listener every time the user inputs a character.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:f="http://xmlns.jcp.org/jsf/core">
<h:head>
<h:outputScript library="javax.faces" name="jsf.js"/>
</h:head>
<h:body>
<h2>JSF add custom ajax event example</h2>
<h:form>
<h:inputText value="#{processingBean.name}">
<f:ajax event="keyup" render="@this" listener="#{processingBean.processing}" />
</h:inputText>
</h:form>
</h:body>
</html>
Demo
URL: http://localhost:8081/jsf-ajax-event/
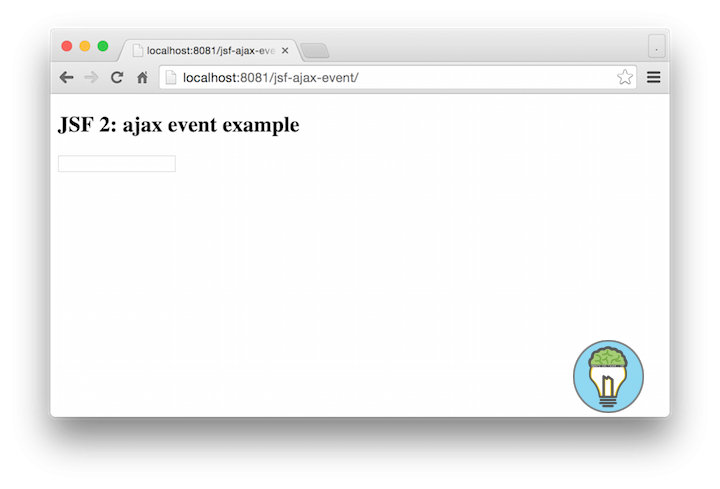
The string is automatically converted into upper case.
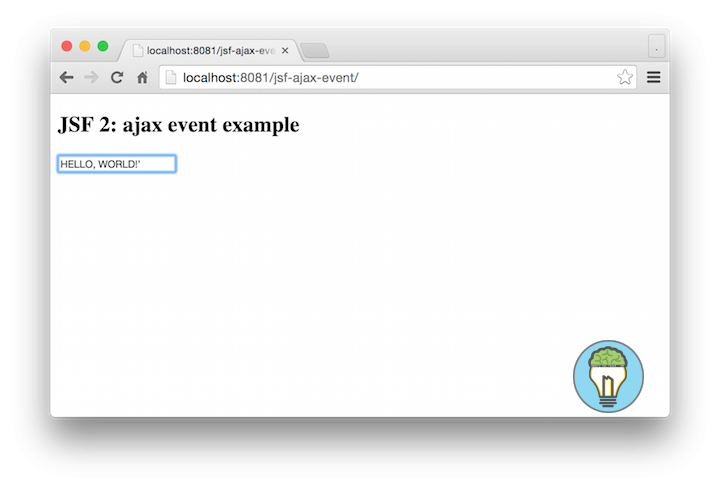