JSF Backing Bean Annotation @ManagedBean example
This tutorial we configure JSF Backing Beans with Annotation Configuration. The @ManagedBean
marks a bean to be managed by JSF. By default if no name attribute is specified it’ll use the fully qualified class name with the first letter in lower case. The bean will be created with lazy initialization which means that the bean will be created only when it’s requested. You can change this by specifying a eager attribute which will eagerly create the managed bean.
Dependencies
Here is a list of dependencies we used to successfuly start the application in tomcat 8.0.24.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.memorynotfound.jsf.managedbeans</groupId>
<artifactId>jsf-managed-bean-xml</artifactId>
<version>1.0.0-SNAPSHOT</version>
<name>JSF - ${project.artifactId}</name>
<url>https://memorynotfound.com</url>
<packaging>war</packaging>
<dependencies>
<!-- java ee api -->
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>7.0</version>
<scope>provided</scope>
</dependency>
<!-- JSF api and impl -->
<dependency>
<groupId>com.sun.faces</groupId>
<artifactId>jsf-api</artifactId>
<version>2.2.12</version>
</dependency>
<dependency>
<groupId>com.sun.faces</groupId>
<artifactId>jsf-impl</artifactId>
<version>2.2.12</version>
</dependency>
<!-- JSP Standard Tag Libraries -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- servlet provided by tomcat -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
JSF Backing Bean Annotation Configuration
The @ManagedBean
demarks the class to be managed by JSF. If no scope is present then the bean will be created with request scope. If we specify a scope greater than request scope we must make the class implement the java.io.Serializable
because JSF can passivate the scope to disk.
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class UserBean {
private String firstName;
private String lastName;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
Servlet Descriptor
We must register the javax.faces.webapp.FacesServlet
to map the requests to JSF.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<display-name>JavaServerFaces</display-name>
<!-- Change to "Production" when you are ready to deploy -->
<context-param>
<param-name>javax.faces.PROJECT_STAGE</param-name>
<param-value>Development</param-value>
</context-param>
<!-- Welcome page -->
<welcome-file-list>
<welcome-file>index.xhtml</welcome-file>
</welcome-file-list>
<!-- JSF mapping -->
<servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<!-- Map these files with JSF -->
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
</web-app>
Calling the Managed Bean
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF Backing Bean Annotation Configuration</h1>
<h:form>
<h:panelGrid columns="2">
<h:outputLabel value="First name" for="firstName"/>
<h:inputText id="firstName" value="#{userBean.firstName}"/>
<h:outputLabel value="Last name" for="lastName"/>
<h:inputText id="lastName" value="#{userBean.lastName}"/>
<h:commandButton action="result" value="send"/>
</h:panelGrid>
</h:form>
</h:body>
</html>
Displaying the result
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF Backing Bean Annotation Configuration</h1>
First name: #{userBean.firstName}
Last name: #{userBean.lastName}
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-managed-bean-annotation/
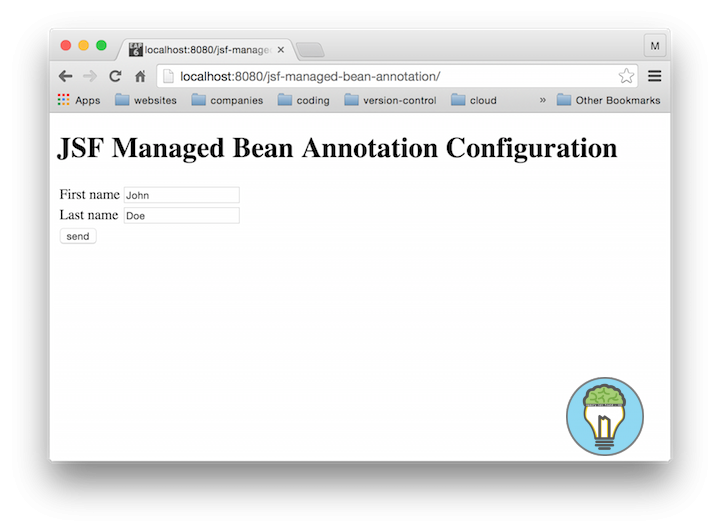
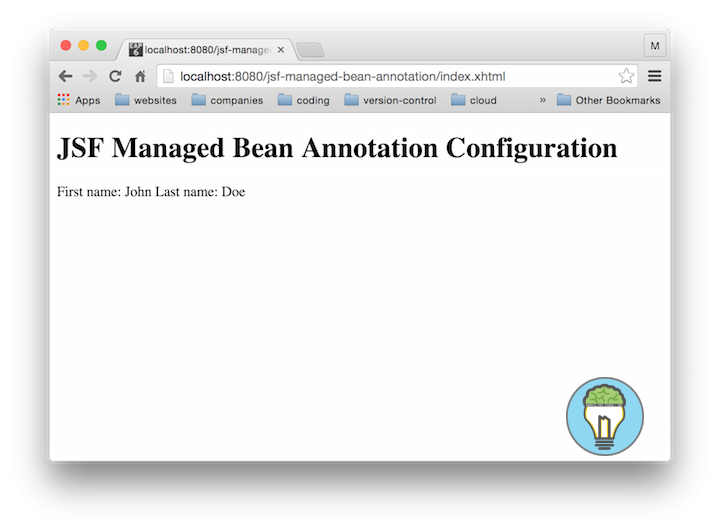