JSF Bookmarkable View Parameters Example
Prior to JSF 2.0 it was hard to accomplish bookmarkable URL’s. Now since JSF 2.0 they have made a good effort. We now have a new category of parameters. JSF Bookmarkable View Parameters. These are implemented by the UIViewParameter
class that extends UIInput
. Let’s take a look at an example how to achieve bookmarkable URL’s in JSF 2.x.
Managed Bean
We are using this managed bean to pass a view parameter from one page to another.
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class UserBean {
private int id;
private String name;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Include JSF Bookmarkable View Parameters
View parameters can be included in links by using the includeVidwParams=”true”.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:f="http://xmlns.jcp.org/jsf/core"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF 2.2 Bookmarkable View Parameters</h1>
<h:link value="click link with parameters" outcome="result" includeViewParams="true">
<f:param name="id" value="13"/>
<f:param name="name" value="John Doe"/>
</h:link>
</h:body>
</html>
Map and validate Bookmarkable View Parameters
JSF gets the request parameter’s values by name from the page URL and applies optional specified converters/validators. After conversion/validation succeeds and before the view is rendered, JSF binds the values of the request parameters to the managed bean properties by calling the appropriate setter methods.
Since the view parameters are of type UIViewParameter
and extend the UiInput
class, this means that it inherits all attributes such as required and requiredMessage. When you need to ensure the url must contain certain attributes and the application flow is correct you can inform the user with the appropriate message, by using the <h:messages>
.
View Parameters extend from
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:f="http://xmlns.jcp.org/jsf/core"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:head>
<f:metadata>
<f:viewParam name="id" value="#{userBean.id}" required="true" requiredMessage="id is required"/>
<f:viewParam name="name" value="#{userBean.name}"/>
</f:metadata>
</h:head>
<h:body>
<h:messages style="color: red"/>
<h1>JSF 2.2 Bookmarkable View Parameters</h1>
<h:panelGrid columns="2">
<h:outputLabel value="Requested id: "/>
<h:outputText value="#{userBean.id}"/>
<h:outputLabel value="Requested name: "/>
<h:outputText value="#{userBean.name}"/>
</h:panelGrid>
</h:body>
</html>
Servlet Descriptor
Don’t forget to register the FacesServlet
in the servlet descriptor.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<display-name>JavaServerFaces</display-name>
<!-- Change to "Production" when you are ready to deploy -->
<context-param>
<param-name>javax.faces.PROJECT_STAGE</param-name>
<param-value>Development</param-value>
</context-param>
<!-- Welcome page -->
<welcome-file-list>
<welcome-file>index.xhtml</welcome-file>
</welcome-file-list>
<!-- JSF mapping -->
<servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<!-- Map these files with JSF -->
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
</web-app>
Demo
URL: http://localhost:8080/jsf-view-parameters/
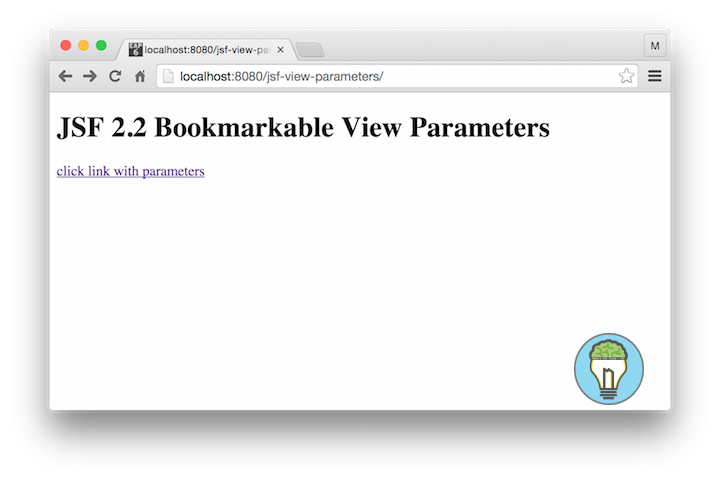
URL: http://localhost:8080/jsf-view-parameters/result.xhtml?id=13&name=John+Doe
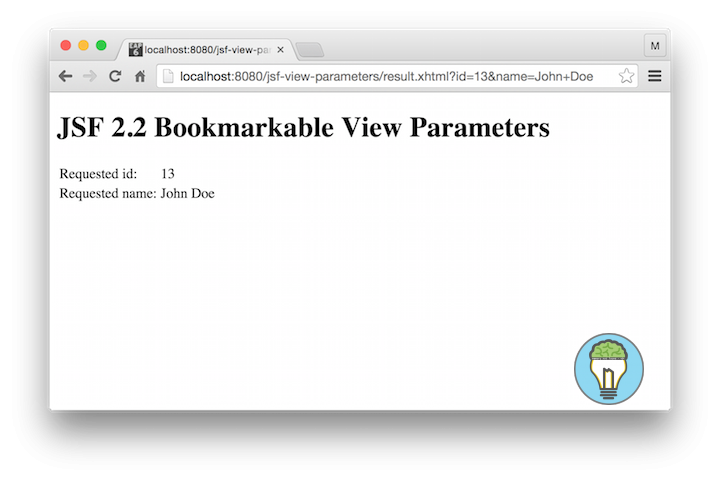
URL: http://localhost:8080/jsf-view-parameters/result.xhtml?name=John+Doe
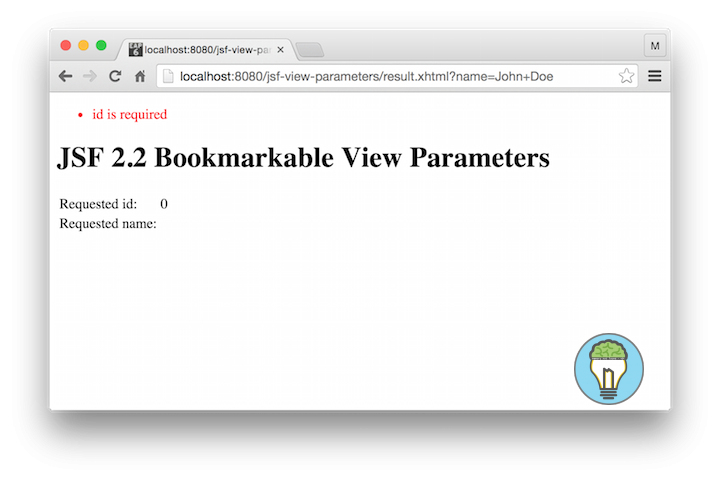