JSF CommandButton Example
This tutorial explains how to use the <h:commandButton>
element.
The <h:commandButton>
generates by default a HTML <input type="submit">
element which submits the parent form using an HTTP POST method. This form is required. If the form is not present and the javax.faces.PROJECT_STAGE is set to Development this will produce the following message: "The form component needs to have a UIForm in its ancestry. Suggestion: enclose the necessary components within <h:form>"
.
When the command button is invoked, any actions attached like action
, actionListener
and/or <f:ajax listener>
are executed. Let’s see how this works in a concrete example.
Managed Bean
This mananaged bean holds the value from the form submission.
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class HelloBean {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Demonstration of JSF CommandButton
This page demonstrates the different usages of the <h:commandButton>
. You can have three types of command buttons:
- submit is the default type, you can either leave out the type or explicitly declare as type submit.
- reset will reset all the values inside the parent form.
- button will make an html input button of type button. The button will not be able to submit form values.
Since JSF 2.2 you can enhance HTML elements with JSF using the http://xmlns.jcp.org/jsf namespace. This makes it possible to render an html page without deploying it to a web server.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:jsf="http://xmlns.jcp.org/jsf">
<h:body>
<h2>JSF command button example</h2>
<h:form id="bf">
<h:outputLabel value="Favorite beer:">
<h:inputText id="name" value="#{helloBean.name}"/>
</h:outputLabel>
<h4>jsf h:commandButton</h4>
<h:commandButton id="submit-btn" value="Process" action="result"/>
<h4>jsf h:commandButton type button</h4>
<h:commandButton id="button-btn" value="Process" type="button" action="result"/>
<h4>jsf h:commandButton type reset</h4>
<h:commandButton id="reset-btn" value="Process" type="reset" action="result"/>
<h4>html input type submit enhanced with jsf</h4>
<input jsf:id="jsf-submit-btn" type="submit" jsf:value="Process" jsf:action="result"/>
</h:form>
</h:body>
</html>
The submitted result will be displayed on a result page.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h2>JSF command button example</h2>
<p>hello, #{helloBean.name}!</p>
</h:body>
</html>
Demo
URL: http://localhost:8081/jsf-command-button/
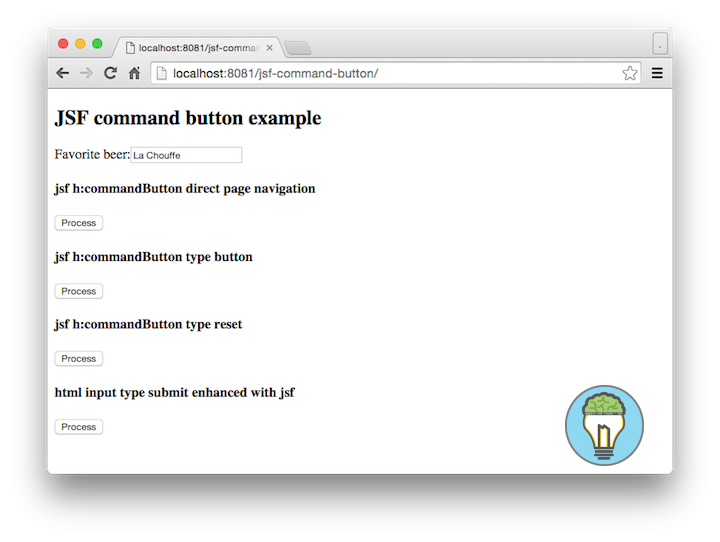
When result is submitted.
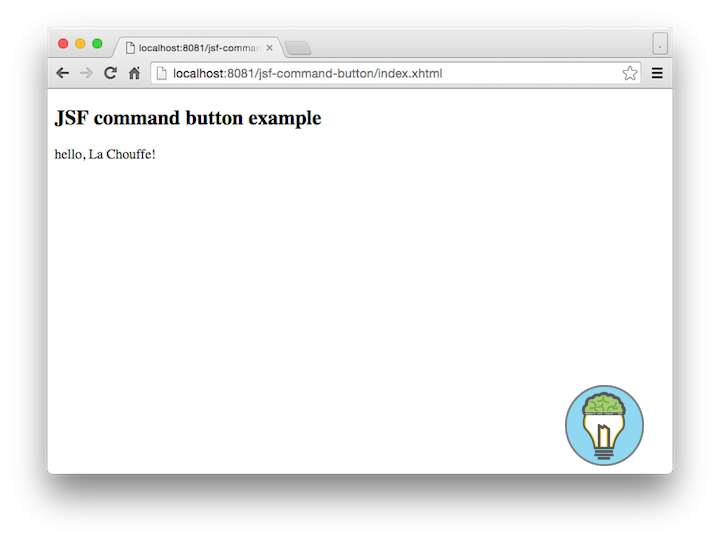
When button with type reset is pressed, the form is cleared back to default.
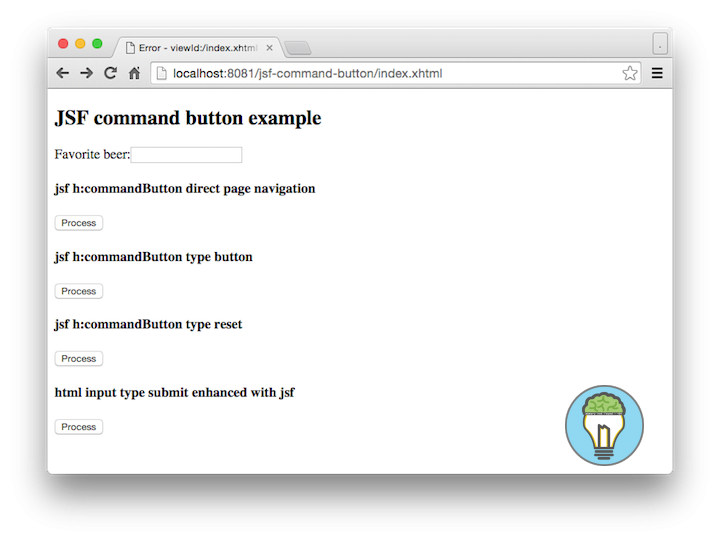