JSF ConvertNumber – Standard Converter For Numbers
This tutorial shows how to use the <f:convertNumber>
tag. The convertNumber is a standard JSF converter, which converts String/Numbers into a specified format. This converter can be customized using a set of attributes, listed next:
- type represents the type of number, by default this type is set to number, but you can set it to currency or percent
- minIntegerDigits represents the minimum number of integer digits to display.
- maxIntegerDigits represents the maximum number of integer digits to display.
- minFractionDigits represents the minimum number of fractional digits to display.
- maxFractionDigits represents the maximum number of fractional digits to display.
- groupingUsed represents whether you want to use grouping or not. By default it is set to true.
- currencyCode represents a three-digit international currency code when the attribute type is currency.
- currencySymbol represents a currency symbol like $, ¥ or €. Is to be used when the type is currency.
- integerOnly if specified the fractional digits are ignored. By default it is set to false.
- pattern represents the decimal format pattern used to convert this number.
- locale represents the locale to be used for displaying this number.
Managed Bean
We are converting the price property of the CourseBean
Managed bean. Note that we start out with a double value of 12345.12345.
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class CourseBean {
private double price = 12345.12345;
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
JSF Standard Number Converter
The <f:convertNumber>
is a standard JSF Number Converter. In this page we convert the number of the CourseBean
into a couple of number-presentations including: display maximal fraction digits, add a currency symbol before the decimal, format a number as percentage and use a pattern to format the number.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core">
<h:body>
<h2>JSF convert number Example</h2>
<h:form>
<h:panelGrid columns="2">
<h:outputText value="Format as 00000.00000: "/>
<h:outputText value="#{courseBean.price}">
<f:convertNumber type="number"
maxIntegerDigits="5"
maxFractionDigits="5"
groupingUsed="false"/>
</h:outputText>
<h:outputText value="Format as 00000: "/>
<h:outputText value="#{courseBean.price}">
<f:convertNumber type="number"
maxIntegerDigits="5"
maxFractionDigits="0"/>
</h:outputText>
<h:outputText value="Format as currency: "/>
<h:outputText value="#{courseBean.price}">
<f:convertNumber type="currency"
currencySymbol="$"
maxIntegerDigits="5"
maxFractionDigits="2"/>
</h:outputText>
<h:outputText value="Format as percent: "/>
<h:outputText value="#{courseBean.price}">
<f:convertNumber type="percent"
maxIntegerDigits="5"
maxFractionDigits="5"/>
</h:outputText>
<h:outputText value="Format as pattern #####,00%: "/>
<h:outputText value="#{courseBean.price}">
<f:convertNumber pattern="#####,00%"/>
</h:outputText>
</h:panelGrid>
</h:form>
</h:body>
</html>
Demo
URL: http://localhost:8081/jsf-convertnumber/
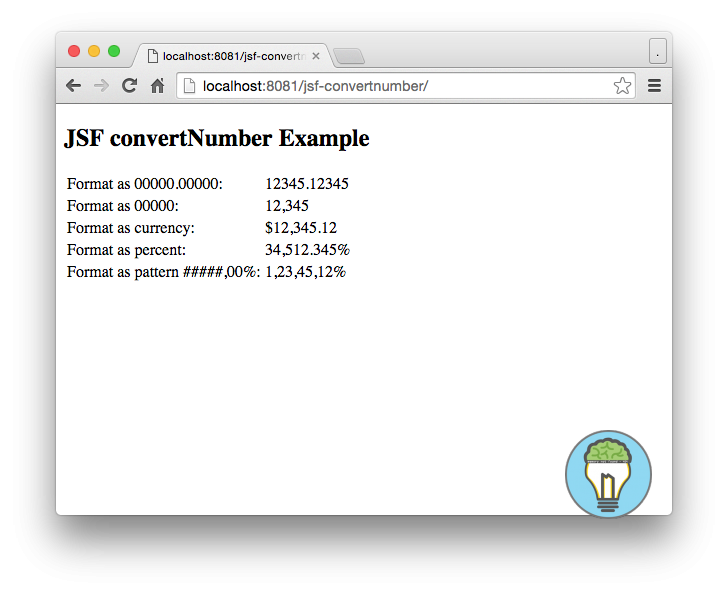