JSF Facelets Templating Example
This tutorial explains how to use JSF facelets templating. Templating is the ability to reuse portion of your layout in order to follow the DRY (Don’t Repeat Yourself) principle. This allows you to easily structure your web page into multiple reusable components. Templating also helps in maintaining a standard look and feel in an application with a large number of pages. JavaServer faces technology provides the tools to implement user interfaces that are easy to extend and reuse.
Main Layout
A template is just a normal XHTML page with custom JSF Facelets tags to define the template layout. These templates can be overridden to accumulate custom dynamic content with the same layout. We are using this template to act as the base of our design.
ui:insert
is used to define replaceable sections.ui:include
is used to provide a default content. If the child page does not override the template this will act as a default.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:ui="http://java.sun.com/jsf/facelets">
<h:head>
<title>
<ui:insert name="title">default title</ui:insert>
</title>
<h:outputStylesheet library="css" name="style.css"/>
</h:head>
<h:body>
<ui:insert name="header" >
<ui:include src="/templates/common/header.xhtml" />
</ui:insert>
<ui:insert name="content" >
<ui:include src="/templates/common/content.xhtml" />
</ui:insert>
<ui:insert name="footer" >
<ui:include src="/templates/common/footer.xhtml" />
</ui:insert>
<h:outputScript library="js" name="script.js"/>
</h:body>
</html>
Header, Content and Footer Template
Here we will create our templates: header, content and footer.
<ui:composition
xmlns="http://www.w3.org/1999/xhtml"
xmlns:ui="http://java.sun.com/jsf/facelets">
<header>
<h1>This is default header</h1>
</header>
</ui:composition>
<ui:composition
xmlns="http://www.w3.org/1999/xhtml"
xmlns:ui="http://java.sun.com/jsf/facelets">
<section class="content">
<h1>This is default content</h1>
</section>
</ui:composition>
<ui:composition
xmlns="http://www.w3.org/1999/xhtml"
xmlns:ui="http://java.sun.com/jsf/facelets">
<footer>
<h1>This is default footer</h1>
</footer>
</ui:composition>
Index Page
This will be our actual page. If we don’t override any parts of our template we receive the default configuration.
<ui:composition template="/templates/layout/main-layout.xhtml"
xmlns="http://www.w3.org/1999/xhtml"
xmlns:ui="http://java.sun.com/jsf/facelets">
</ui:composition>
Generated Output
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"><head id="j_idt2">
<title>default title</title>
<link type="text/css" rel="stylesheet" href="/jsf-templating/javax.faces.resource/style.css.xhtml?ln=css" />
</head><body>
<header>
<h1>This is default header</h1>
</header>
<section class="content">
<h1>This is default content</h1>
</section>
<footer>
<h1>This is default footer</h1>
</footer></body>
</html>
Example Output
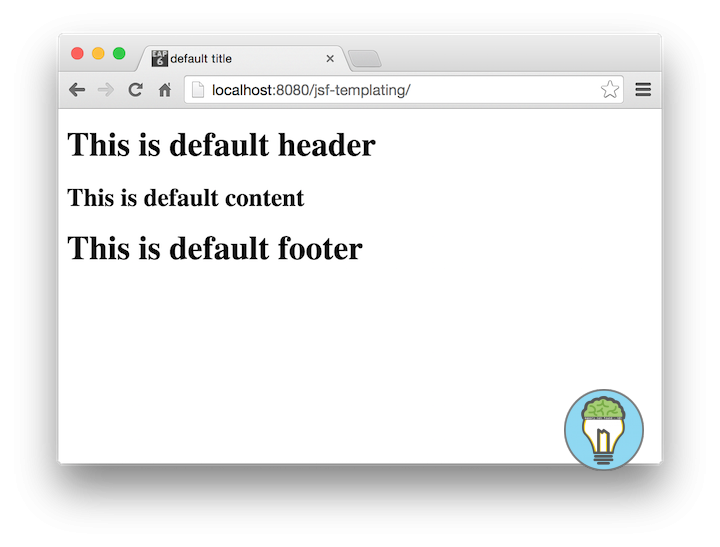
Overridden Page
<ui:composition template="/templates/layout/main-layout.xhtml"
xmlns="http://www.w3.org/1999/xhtml"
xmlns:ui="http://java.sun.com/jsf/facelets">
<ui:define name="title">Override.xhtml</ui:define>
<ui:define name="content">
<h2>This is page1 content</h2>
</ui:define>
<ui:define name="footer">
<h2>This is page1 Footer</h2>
</ui:define>
</ui:composition>
Generated Output
We can also override certain templates. Here is an example.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"><head id="j_idt2">
<title>Override.xhtml
</title>
<link type="text/css" rel="stylesheet" href="/jsf-templating/javax.faces.resource/style.css.xhtml?ln=css" />
</head>
<body>
<header>
<h1>This is default header</h1>
</header>
<h2>This is page1 content</h2>
<h2>This is page1 Footer</h2>
<script type="text/javascript" src="/jsf-templating/javax.faces.resource/script.js.xhtml?ln=js"></script></body>
</html>
Example Output
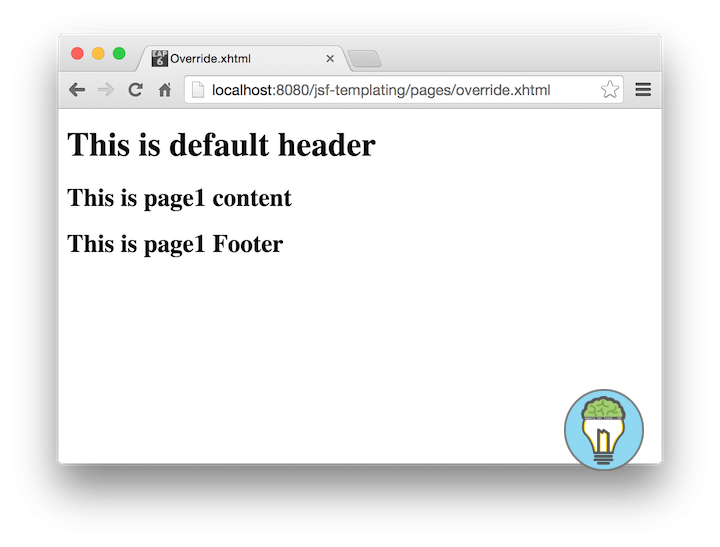