JSF FacesBehavior Confirm Delete Behavior
In this tutorial we will show a under used feature of JSF 2: FacesBehavior. With FacesBehavior you can add client side programming at the backend. In this example we’ll create a simple confirm delete dialog.
Confirm Delete FacesBehavior
First we annotate our class with @FacesBehavior
and extend the ClientBehaviorBase
class. This gives us a getScript()
method which we need to override. This method adds an onclick method to the element.
package com.memorynotfound.jsf;
import javax.faces.component.behavior.ClientBehaviorBase;
import javax.faces.component.behavior.ClientBehaviorContext;
import javax.faces.component.behavior.FacesBehavior;
@FacesBehavior(value = "confirm")
public class ConfirmDeleteBehavior extends ClientBehaviorBase {
@Override
public String getScript(ClientBehaviorContext behaviorContext) {
return "return confirm('Are you sure ? ')";
}
}
Creating the tag
We must create a tag so that we can link to this tag in our xhtml file.
<?xml version="1.0"?>
<!DOCTYPE facelet-taglib PUBLIC
"-//Sun Microsystems, Inc.//DTD Facelet Taglib 1.0//EN"
"http://java.sun.com/dtd/facelet-taglib_1_0.dtd">
<facelet-taglib version="2.0"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-facelettaglibrary_2_0.xsd">
<namespace>https://memorynotfound.com/example</namespace>
<tag>
<tag-name>confirmDelete</tag-name>
<behavior>
<behavior-id>confirm</behavior-id>
</behavior>
</tag>
</facelet-taglib>
Registering the tag in the web.xml
We need to register our new tag in the web.xml file. We do this by adding a context-param with the name javax.faces.FACELETS_LIBRARIES and value the location of our tag.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<!-- register custom tag -->
<context-param>
<param-name>javax.faces.FACELETS_LIBRARIES</param-name>
<param-value>/WEB-INF/delete.taglib.xml</param-value>
</context-param>
<!-- Change to "Production" when you are ready to deploy -->
<context-param>
<param-name>javax.faces.PROJECT_STAGE</param-name>
<param-value>Development</param-value>
</context-param>
<!-- Welcome page -->
<welcome-file-list>
<welcome-file>index.xhtml</welcome-file>
</welcome-file-list>
<!-- JSF mapping -->
<servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<!-- Mappings for JSF FacesServlet -->
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
</web-app>
Setting the tag on a component
Finally we can use our new tag on a component. Make sure to include the namespace of our tag.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:b="https://memorynotfound.com/example">
<h:body>
<h2>JSF FacesBehavior Confirm Delete Behavior </h2>
<h:form>
<h:commandButton value="Delete" action="done">
<b:confirmDelete/>
</h:commandButton>
</h:form>
</h:body>
</html>
Demo
URL: http://localhost:8081/jsf-confirm-dialog-behavior/
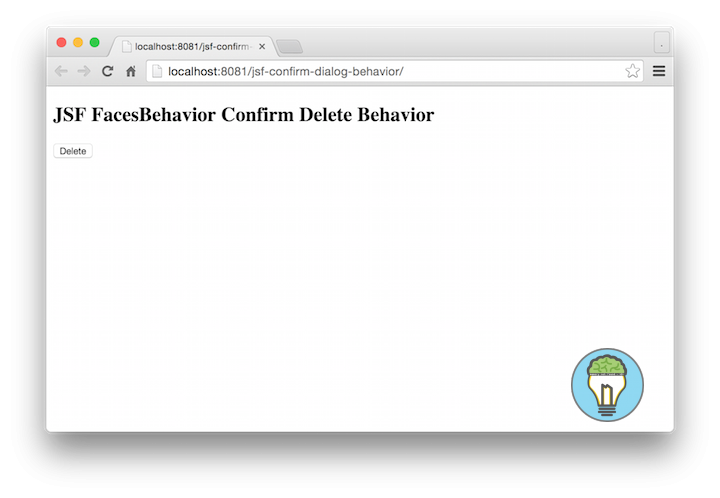
The delete confirm dialog.
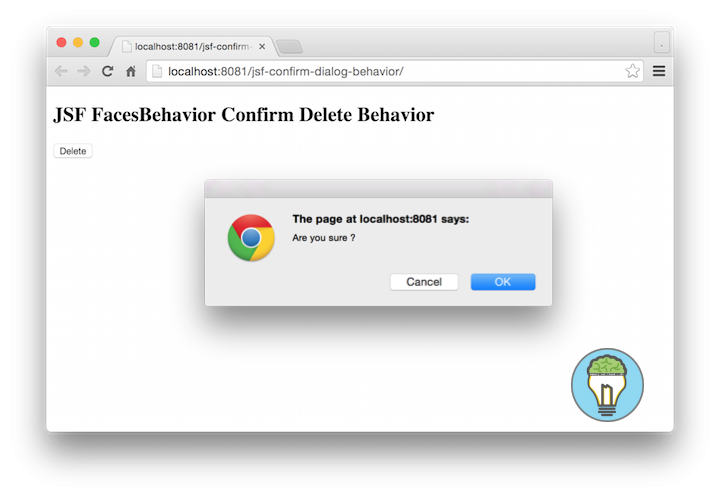