JSF Hidden Input Value Example
In this example we show the usage of the JSF hidden input value tag. The tag can be used to pass temporary data or information provided by the user that should be used again. JSF has the tag <h:inputHidden> tag to pass these hidden parameters.
JSF Tag Conversion
In JSF you can use the <h:inputHidden> tag to render a hidden input field on the HTML page.
<h:inputHidden value="#{orderBean.hidden}" id="name"/>
The previous code will be rendered as the following in HTML.
<input id="name" type="hidden" name="name" value="Some hidden value" />
The equivalent to the prior JSF tag is the new JSF tag.
<input type="hidden" jsf:id="description" jsf:value="#{orderBean.description}"/>
JSF Hidden Input Value Example
In this tutorial we will fill a JSF hidden input value field and display the hidden value by displaying it with javascript in an alert box.
Managed Bean
In this managed bean, we set the default value of the hidden field.
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class OrderBean {
private String name = "I'm an hidden name";
private String description = "I'm an hidden description";
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
}
Access JSF Hidden Input value
The jsf hidden input value will not be rendered on the page. We display the value using javascript.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:jsf="http://xmlns.jcp.org/jsf">
<h:body>
<h1>JSF hidden value example</h1>
<h:form id="of">
<h:inputHidden value="#{orderBean.name}" id="name"/>
<input type="hidden" jsf:id="description" jsf:value="#{orderBean.description}"/>
<h:commandLink value="Click me" onclick="displayHiddenValue()" />
</h:form>
<script type="text/javascript">
function displayHiddenValue(){
alert(document.getElementById("of:name").value + "\n" +
document.getElementById("of:description").value);
}
</script>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-hidden-input-value
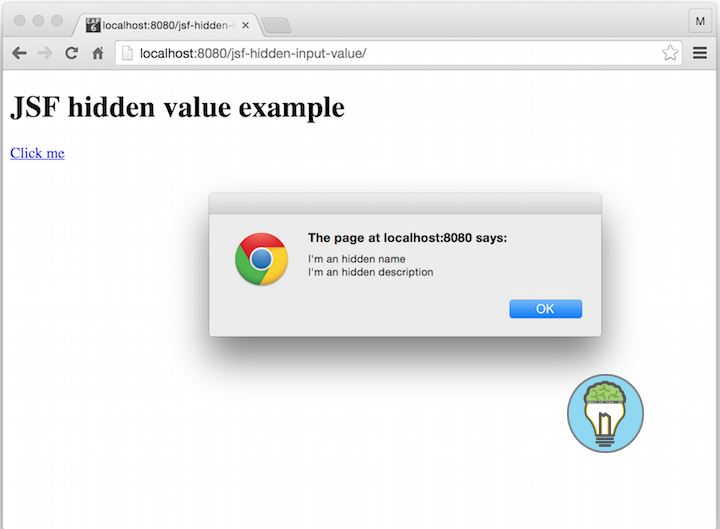
awesome tutorial