JSF How to Find Component Programatically
In this tutorial we show you how to find component programatically in JSF. Some times you may need to manipulate an attribute, component, validator, etc.. In order to manipulate your component we need a reference to that component.
Find Component Programatically
You can get the component tree by getViewRoot()
of the FacesContext
and find a particular component by ID by findComponent()
method.
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import javax.faces.component.UIComponent;
import javax.faces.component.UIViewRoot;
import javax.faces.context.FacesContext;
@ManagedBean
@RequestScoped
public class UserBean {
private String name;
public String processAction(){
UIViewRoot view = FacesContext.getCurrentInstance().getViewRoot();
UIComponent component = view.findComponent("uf:name");
component.getAttributes().put("value", "John");
return null;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Give your components an identity
Don’t forget to name your components. Give your form and component a unique id.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF Find Component Programatically</h1>
<h:form id="uf">
<h:inputText id="name" value="#{userBean.name}"/>
<h:commandButton action="#{userBean.processAction}" value="Submit"/>
</h:form>
</h:body>
</html>
Demo
URL: http://localhost:8081/jsf-find-component/
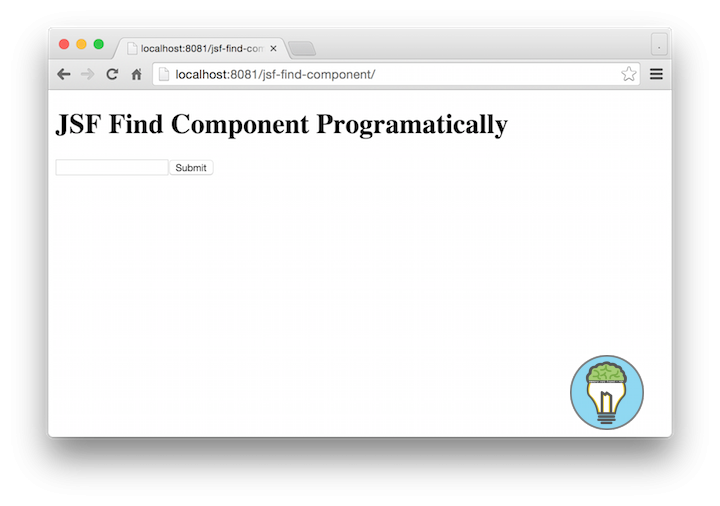
Component is found and given a value of “john”.
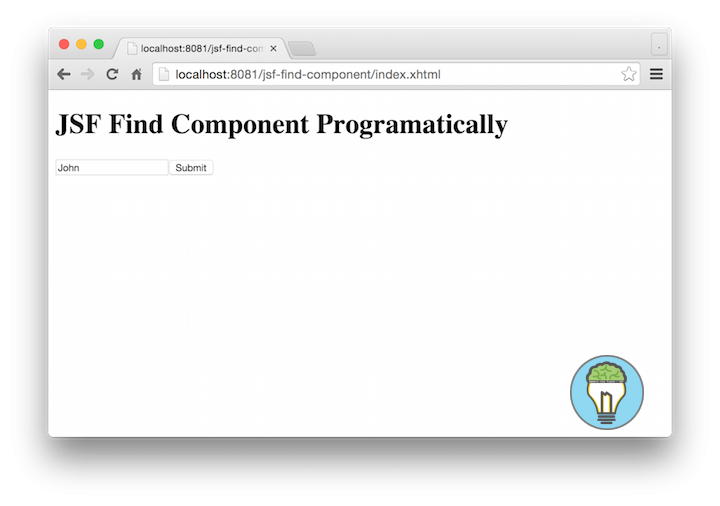