JSF Identify Browser Tab with ClientWindow example
In this example we’ll identify browser tabs with JSF 2 Client Window API. In the past it was almost impossible to identify from where a response was coming. As the HTTP specification is stateless and that almost every JSF application requires some kind of state, some errors could occur when the user opened many tabs in the browser. Since JSF 2 there is a new Client Window API which can help you to identify from which browser tab the request is coming.
Window Managed Bean
This managed bean will enable or disable the window rendering mode. By enabling the window rendering mode JSF automatically identifies the current window id by adding a unique id to the URL. The unique id will be the value of the jfwid attribute.
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import javax.faces.context.ExternalContext;
import javax.faces.context.FacesContext;
import javax.faces.lifecycle.ClientWindow;
@ManagedBean
@RequestScoped
public class WindowBean {
public void getWindowID(){
FacesContext facesContext = FacesContext.getCurrentInstance();
ExternalContext externalContext = facesContext.getExternalContext();
ClientWindow clientWindow = externalContext.getClientWindow();
if (clientWindow != null){
System.out.println("client window id: " + clientWindow.getId());
} else {
System.out.println("client window cannot be determined!");
}
}
public String enableClientWindow(){
FacesContext facesContext = FacesContext.getCurrentInstance();
ExternalContext externalContext = facesContext.getExternalContext();
ClientWindow clientWindow = externalContext.getClientWindow();
clientWindow.enableClientWindowRenderMode(facesContext);
return "index?faces-redirect=true";
}
public String disableClientWindow(){
FacesContext facesContext = FacesContext.getCurrentInstance();
ExternalContext externalContext = facesContext.getExternalContext();
ClientWindow clientWindow = externalContext.getClientWindow();
clientWindow.disableClientWindowRenderMode(facesContext);
return "index?faces-redirect=true";
}
}
Enabling client window mode
To enable the client window mode you must set the javax.faces.CLIENT_WINDOW_MODE to url. By default it results to none. These are the only two options available at this time.
<!-- enable cliend window mode -->
<context-param>
<param-name>javax.faces.CLIENT_WINDOW_MODE</param-name>
<param-value>url</param-value>
</context-param>
Enabling or disabling the window id via ClientWindow
There are two ways you can enable the client window rendering mode.
- Programatically, as we saw in the managed bean.
- There is also an attribute on the
<h:link>
element.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h2>JSF Identify Browser Tab example</h2>
<h4>Output links</h4>
<h:panelGrid columns="2">
<h:link value="Enable client window" outcome="index" disableClientWindow="false"/>
<h:link value="Disable client window" outcome="index" disableClientWindow="true"/>
</h:panelGrid>
<h4>Command buttons</h4>
<h:form>
<h:commandButton value="Get client window" actionListener="#{windowBean.getWindowID}"/>
<h:commandButton value="Enable client window" action="#{windowBean.enableClientWindow}"/>
<h:commandButton value="Disable client window" action="#{windowBean.disableClientWindow}"/>
</h:form>
</h:body>
</html>
Demo
URL: http://localhost:8081/jsf-identify-open-tabs/index.xhtml
Disabled
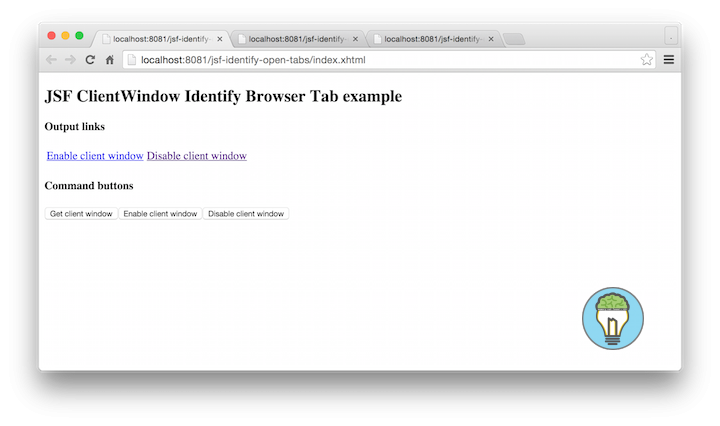
Enabled window 1
URL: http://localhost:8081/jsf-identify-open-tabs/index.xhtml?jfwid=07209F77AFB8D2DEEAB3A3286707E6CD:0
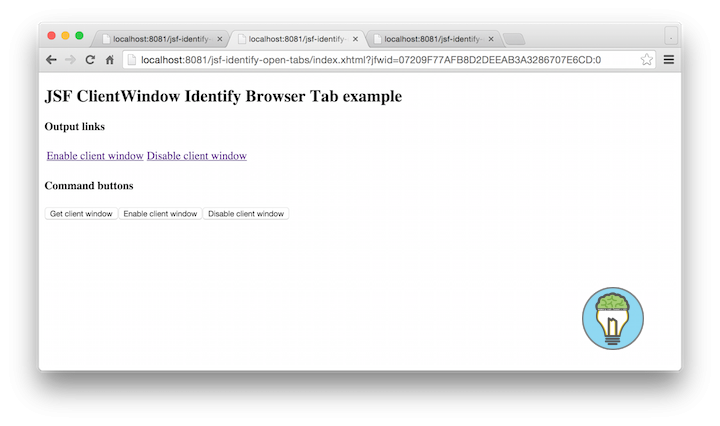
Enabled window 2
URL: http://localhost:8081/jsf-identify-open-tabs/index.xhtml?jfwid=07209F77AFB8D2DEEAB3A3286707E6CD:1
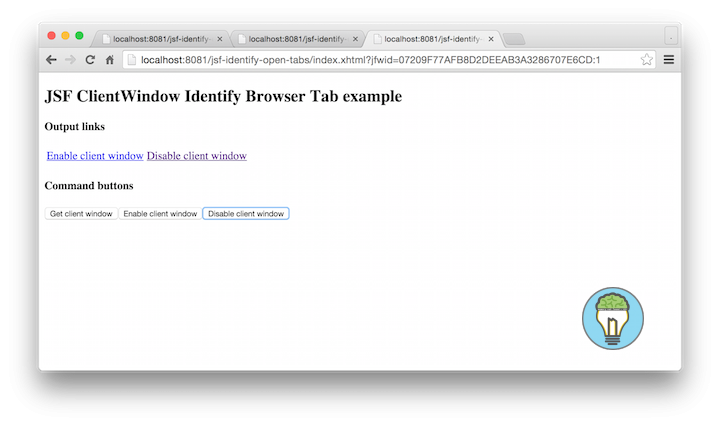
great example. exactly what i was looking for!