JSF Managed Property Example
This example shows how to use jsf managed property. When annotating a field with @ManagedProperty
the values are set immediately after the bean’s construction and are available during @PostConstruct
. Be informed that you can only use @ManagedProperty
with beans managed by JSF @ManagedBean
, not with beans managed by CDI @Named
.
JSF Managed Property
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core">
<h:body>
<h1>JSF 2.2 Managed Property</h1>
<h:form>
<h:commandButton value="Send..." action="#{requestController.parametersAction()}">
<f:param id="paramMP1" name="paramMP1Name" value="paramMP1Value"/>
<f:param id="paramMP2" name="paramMP2Name" value="paramMP2Value"/>
</h:commandButton>
</h:form>
</h:body>
</html>
JSF Managed Property BackingBean
Warning: Keep in mind that
@ManagedProperty
is usable only with beans managed by JSF (@ManagedBean
), not with beans managed by CDI (@Named
).package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.ManagedProperty;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class RequestController {
@ManagedProperty(value = "#{param.paramMP1Name}")
private String param1MP;
@ManagedProperty(value = "#{param.paramMP2Name}")
private String param2MP;
public String parametersAction(){
return "result";
}
public String getParam1MP() {
return param1MP;
}
public void setParam1MP(String param1MP) {
this.param1MP = param1MP;
}
public String getParam2MP() {
return param2MP;
}
public void setParam2MP(String param2MP) {
this.param2MP = param2MP;
}
}
Display Properties
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html">
<h:body>
<h1>JSF 2.2 Managed Property</h1>
<h4>Managed Property</h4>
<p>#{requestController.param1MP}</p>
<p>#{requestController.param2MP}</p>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-managed-property
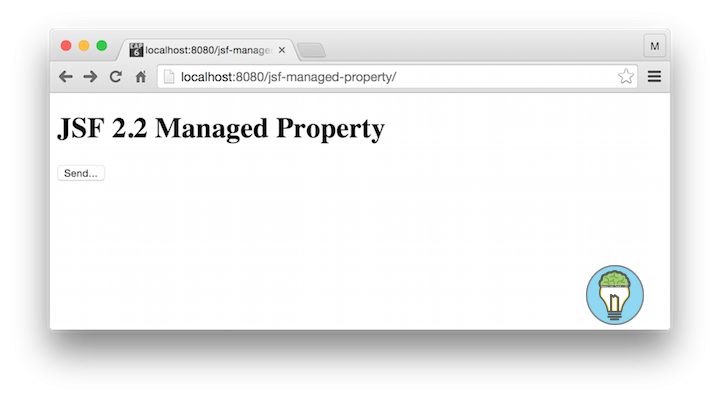
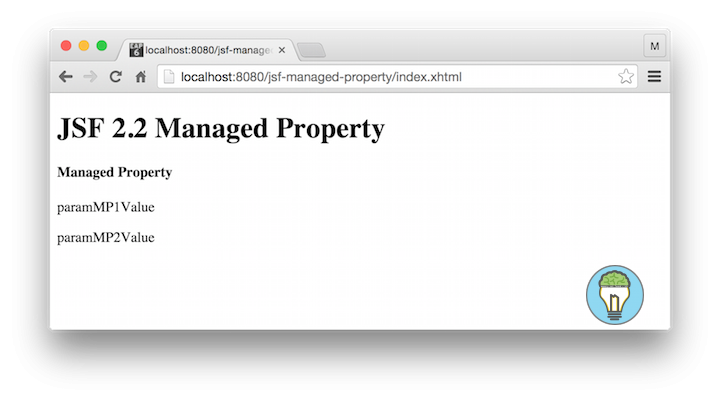
References
Download
Download it ! – jsf-managed-property-example