JSF Passing Request Parameters
This example shows you how you can pass request parameters from a view to your backing bean or view. We are using the <f:param>
which will add a request parameter name-value pair to the request, which we can use lateron. Here is a short example how to achieve this.
Passing request parameters with <f:param>
Sometimes, you need to pass parameters from your view to a managed bean or to another view. You can use the <f:param>
tag for this. This tag puts a string name-value pair to the request.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core">
<h:body>
<h1>JSF 2.2 Passing JSF Request Parameters</h1>
<h:form>
<h:commandButton value="Send..." action="#{requestController.parametersAction()}">
<f:param id="param1" name="param1Name" value="param1Value"/>
<f:param id="param2" name="param2Name" value="param2Value"/>
</h:commandButton>
</h:form>
</h:body>
</html>
Request parameters in Managed Bean
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import javax.faces.context.FacesContext;
import java.util.Map;
@ManagedBean
@RequestScoped
public class RequestController {
private String param1;
private String param2;
public String parametersAction(){
FacesContext fc = FacesContext.getCurrentInstance();
Map<String, String> params = fc.getExternalContext().getRequestParameterMap();
param1 = params.get("param1Name");
param2 = params.get("param2Name");
return "result";
}
public String getParam1() {
return param1;
}
public String getParam2() {
return param2;
}
}
Displaying the result
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html">
<h:body>
<h1>JSF 2.2 Passing JSF Request Parameters</h1>
<h4>Request Map</h4>
<p>#{requestController.param1}</p>
<p>#{requestController.param2}</p>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-request-parameters/
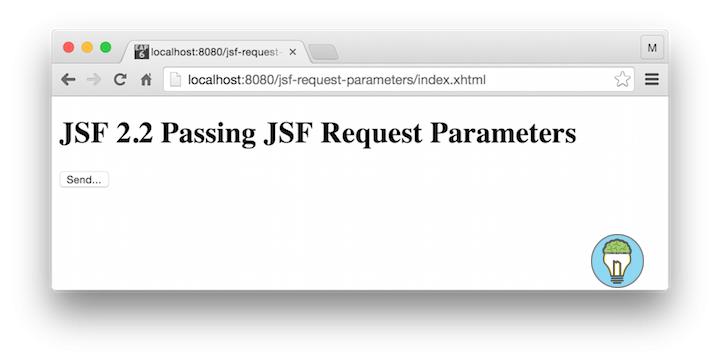
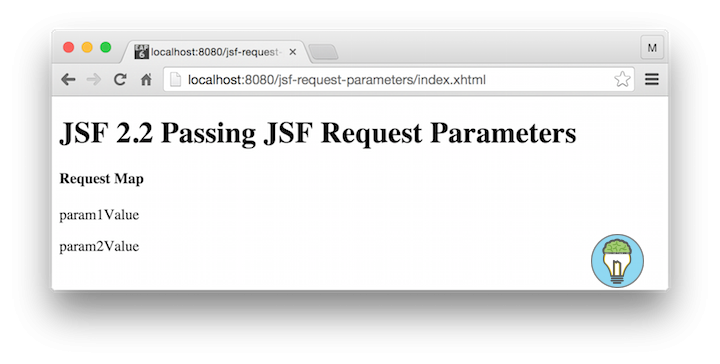