JSF PreRenderView – Execute Initialization Code At page Load
This tutorial show how to execute a initialization method before the page is loaded in JSF. View parameters are not yet available in the @PostConstruct
phase. JSF PreRenderView tackles this problem, you can address this problem by attaching the preRenderView event listener where you can do some custom initialization.
Managed Bean with JSF PreRenderView method
When working with view parameters, these are not yet available in the @PostConstruct
phase. You can resolve this by adding a custom initialization listener method.
package com.memorynotfound;
import javax.annotation.PostConstruct;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import javax.faces.context.FacesContext;
import javax.faces.event.ComponentSystemEvent;
@ManagedBean
@RequestScoped
public class MessageBean {
private String name;
@PostConstruct
public void init(){
System.out.println("@PostConstruct: view params are NOT available here");
System.out.println("Name: " + name);
}
public void before(ComponentSystemEvent event){
if (!FacesContext.getCurrentInstance().isPostback()){
System.out.println("PreRenderView: view parameters are available here");
System.out.println("Name: " + name);
name = name.toUpperCase();
}
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Include View Params to Link
Passing a parameter using the <h:link> element.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:f="http://xmlns.jcp.org/jsf/core"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF 2.2 Bookmarkable View Parameters Listener</h1>
<h:link value="Link with parameter" outcome="result" includeViewParams="true">
<f:param name="name" value="helloWorldMessage"/>
</h:link>
</h:body>
</html>
<f:event> JSF PreRenderView Method
We can register a preRenderView type on the <f:event> element which registers a initialization listener to do some initialization on load of the page.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:f="http://xmlns.jcp.org/jsf/core"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:head>
<f:metadata>
<f:viewParam name="name" value="#{messageBean.name}"/>
<f:event type="preRenderView" listener="#{messageBean.before}"/>
</f:metadata>
</h:head>
<h:body>
<h1>JSF 2.2 Bookmarkable View Parameters Listener</h1>
Requested message name: #{messageBean.name}
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-view-parameter-listener/
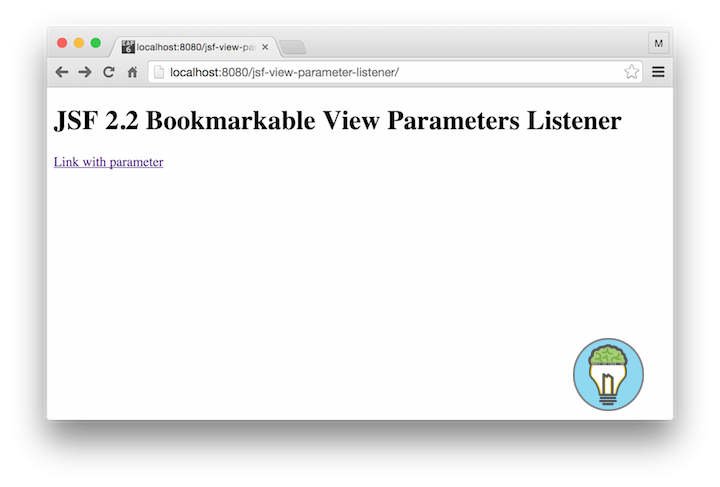
URL: http://localhost:8080/jsf-view-parameter-listener/result.xhtml?name=helloWorldMessage
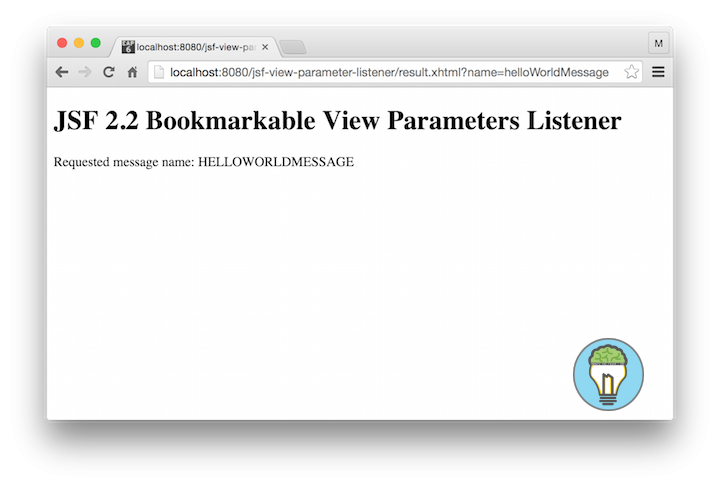
Getting error on import javax.faces.event.ComponentSystemEvent not found.