JSF Setting properties via setPropertyActionListener
In this example we will show you how to set properties directly into the managed bean. With the
Managed Bean
Here is a managed bean that’ll evaluate the setter methods when they are called.
package com.memorynotfound;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class UserBean {
private String firstName;
private String lastName;
public String parametersAction(){
System.out.println("FirstName From parametersAction: " + firstName);
System.out.println("LastName From parametersAction: " + lastName);
return null;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
System.out.println("FirstName From setFirstName: " + firstName);
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
System.out.println("Last Name From setLastName: " + lastName);
this.lastName = lastName;
}
}
Setting properties into the managed bean
When the page is first requested there are no values present for the first and last name properties. When the command button is pressed the setPropertyActionListener will automatically set the values of the target property with the corresponding value.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:f="http://xmlns.jcp.org/jsf/core"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:body>
<h1>JSF Setting properties via setPropertyActionListener</h1>
<h:panelGrid columns="2">
<h:outputText value="First name:"/>
<h:outputText value="#{userBean.firstName}"/>
<h:outputText value="Last name:"/>
<h:outputText value="#{userBean.lastName}"/>
</h:panelGrid>
<h:form>
<h:commandButton value="Send" actionListener="#{userBean.parametersAction}">
<f:setPropertyActionListener value="John" target="#{userBean.firstName}"/>
<f:setPropertyActionListener value="Doe" target="#{userBean.lastName}"/>
</h:commandButton>
</h:form>
</h:body>
</html>
Demo
URL: http://localhost:8081/jsf-action-listener/
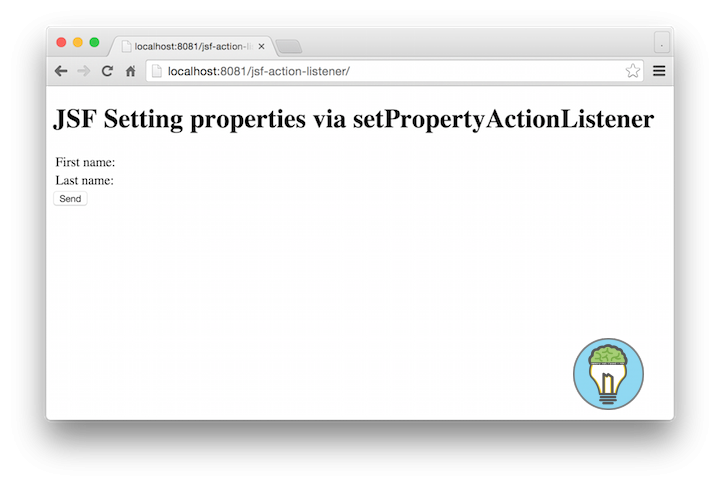
When the command button is pressed.
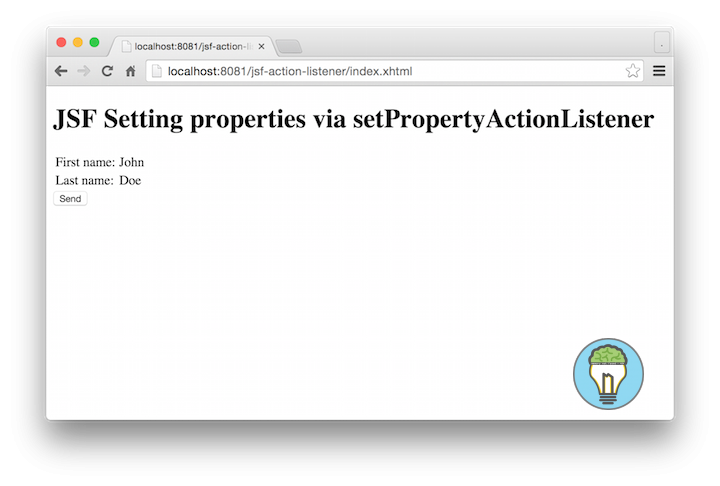