JSF Standard Date and Time Converters
In this tutorial we will show you how you can convert, display, format and localize date and time using the standard Date and Time Converters provided by JSF. We will display date and time in different formats and for different locales.
JSf provides a dedicated converter called converterDateTime
which can be configured using the following attributes.
Attribute Name | Description |
---|---|
type | Specifies whether to display the date, time or both. |
dateStyle | Specifies the formatting style for the date portion of the string. Supported values are medium which is default, short, long and full. This attribute is only valid if the type attribute is set. |
timeStyle | Specifies the formatting style for the time portion of the string. Supported values are medium which is default, short, long and full. This attribute is only valid if the type attribute is set. |
timeZone | Specifies the time zone for the date. By default GMT will be used. |
locale | Specifies the locale to use for displaying the date. |
pattern | Specifies which date format pattern used. |
DateTime Managed bean
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import java.util.Date;
@ManagedBean
@RequestScoped
public class DateTimeBean {
private Date currentDate = new Date();
public Date getCurrentDate() {
return currentDate;
}
public void setCurrentDate(Date currentDate) {
this.currentDate = currentDate;
}
}
Date and Time Converters
Here we provide a couple examples how to convert date and time (using a java.util.Date
instance) using the f:converterDateTime
.
<?xml version='1.0' encoding='UTF-8' ?>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:f="http://xmlns.jcp.org/jsf/core">
<h:head>
<title>Standard Date and Time Converter</title>
</h:head>
<h:body>
<h2>JSF 2: Standard Date and Time Converter Example</h2>
<p>Formatting current date and time</p>
<h:panelGrid columns="1">
<h:outputText value="#{dateTimeBean.currentDate}">
<f:convertDateTime type="date" dateStyle="medium"/>
</h:outputText>
<h:outputText value="#{dateTimeBean.currentDate}">
<f:convertDateTime type="date" dateStyle="full"/>
</h:outputText>
<h:outputText value="#{dateTimeBean.currentDate}">
<f:convertDateTime type="time" dateStyle="full"/>
</h:outputText>
<h:outputText value="#{dateTimeBean.currentDate}">
<f:convertDateTime type="date" pattern="dd/mm/yyyy"/>
</h:outputText>
<h:outputText value="#{dateTimeBean.currentDate}">
<f:convertDateTime dateStyle="full" pattern="yyyy-mm-dd"/>
</h:outputText>
<h:outputText value="#{dateTimeBean.currentDate}">
<f:convertDateTime dateStyle="full" pattern="yyyy.MM.dd 'at' HH:mm:ss z"/>
</h:outputText>
<h:outputText value="#{dateTimeBean.currentDate}">
<f:convertDateTime dateStyle="full" pattern="h:mm a"/>
</h:outputText>
<h:outputText value="#{dateTimeBean.currentDate}">
<f:convertDateTime dateStyle="long" timeZone="EST" type="both"/>
</h:outputText>
<h:outputText value="#{dateTimeBean.currentDate}">
<f:convertDateTime locale="fr" timeStyle="long" type="both" dateStyle="full"/>
</h:outputText>
<h:outputText value="#{dateTimeBean.currentDate}">
<f:convertDateTime locale="us" timeStyle="short" type="both" dateStyle="full"/>
</h:outputText>
</h:panelGrid>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-date-time-converter/
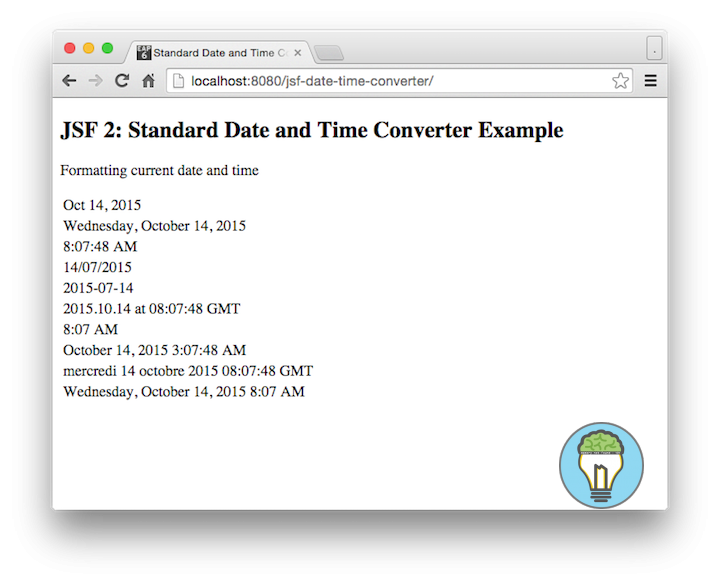