JSF Validate Double Range Input Field
This tutorial explains how to use f:validateDoubleRange
a JSF validate double range validator. This element takes two attributes minimum and maximum to configure the required length. This validator validates if an input field has is between the double minimum or maximum value.
<h:inputText id="price" label="Price" value="#{couseBean.price}">
<f:validateDoubleRange minimum="0.01" maximum="9.99"/>
</h:inputText>
JSF Managed Bean
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class CouseBean {
private double price;
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
Validate Emtpy Input
If you are experiencing that JSF does not validate empty fields, then you should add the following snippet to your web.xml. Using this property tells JSF to validate empty fields.
<context-param>
<param-name>javax.faces.VALIDATE_EMPTY_FIELDS</param-name>
<param-value>true</param-value>
</context-param>
JSF Validate Double Range
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core">
<h:body>
<h2>JSF validate double range example</h2>
<h:form>
<h:panelGrid columns="2">
<h:outputLabel value="Price:">
<h:inputText id="price" label="Price" value="#{couseBean.price}">
<f:validateDoubleRange minimum="0.01" maximum="9.99"/>
</h:inputText>
</h:outputLabel>
<h:message for="price" style="color:red" />
</h:panelGrid>
<h:commandButton value="submit" action="result"/>
</h:form>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-validate-double-range/
JSF Validate Double Range
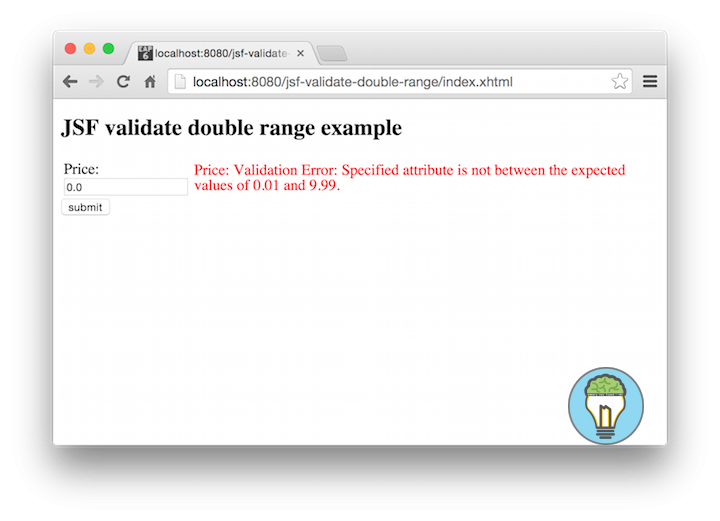