JSF Validate Long Range Input Field
This tutorial shows how to use f:validateLongRange
JSF Validate Long Range Input Field. This element takes two attributes minimum and maximum these are used to validate if the input field does not exceed these boundaries.
<h:inputText id="age" label="Age" value="#{userBean.age}">
<f:validateLongRange minimum="18" maximum="150"/>
</h:inputText>
JSF Managed Bean
package com.memorynotfound.jsf;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class UserBean {
private Integer age;
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
}
Validate Emtpy Input
If you are experiencing that JSF does not validate empty fields, then you should add the following snippet to your web.xml. Using this property tells JSF to validate empty fields.
<context-param>
<param-name>javax.faces.VALIDATE_EMPTY_FIELDS</param-name>
<param-value>true</param-value>
</context-param>
JSF Validate Long Range
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core">
<h:body>
<h2>JSF validate long range example</h2>
<h:form>
<h:panelGrid columns="2">
<h:outputLabel value="Age:">
<h:inputText id="age" label="Age" value="#{userBean.age}">
<f:validateLongRange minimum="18" maximum="150"/>
</h:inputText>
</h:outputLabel>
<h:message for="age" style="color:red" />
</h:panelGrid>
<h:commandButton value="submit" action="result"/>
</h:form>
</h:body>
</html>
Demo
URL: http://localhost:8080/jsf-validate-long-range/
JSF Validate Long Range
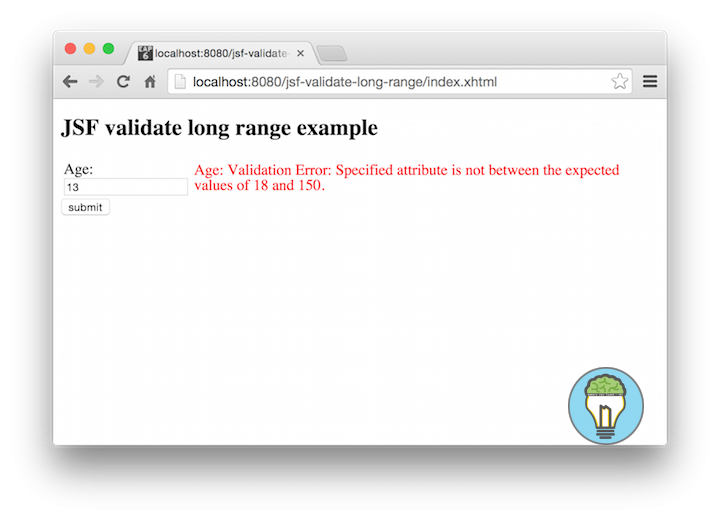