Servlet 3 Exception Handling example
In this tutorial we will tackle servlet 3 exception handling. When a servlet generates an error we can handle those exceptions on various ways, lets say a user tries a URL that does not map to a servlet the user typically gets a 404 page. With the error listing in our web.xml also known as deployment descriptor we can handle those exceptions.
Project structure
+--src
| +--main
| +--java
| +--com
| +--memorynotfound
| |--ErrorServlet.java
| |--ExampleServlet.java
| |--resources
| +--webapp
| +--WEB-INF
| |--web.xml
pom.xml
Maven Dependency
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
Defining our Servlet that will produce an error
This servlet is used to throw an error tot test our configuration.
package com.memorynotfound;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet("/error")
public class ExampleServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
throw new UnsupportedOperationException("HTTP GET request is not allowed!");
}
}
Servlet 3 Exception Handling
We will map this servlet in our servlet descriptor to handle all the exception. You can get information about the exception that occurred from the request attributes. Here is a list of possible attributes that are in the request when an exception occurred.
javax.servlet.error.exception
will hold information about the exception thrown.javax.servlet.error.status_code
will hold the statuscode returned by the container.javax.servlet.error.servlet_name
will hold the servlet name in case the exception is thrown from within a servlet.javax.servlet.error.request_uri
will hold the request URI from where the error request originated.
package com.memorynotfound;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet("/error-handler")
public class ErrorServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// Analyze the servlet exception
Exception exception = (Exception)req.getAttribute("javax.servlet.error.exception");
Integer statusCode = (Integer)req.getAttribute("javax.servlet.error.status_code");
String servletName = (String)req.getAttribute("javax.servlet.error.servlet_name");
String requestUri = (String)req.getAttribute("javax.servlet.error.request_uri");
// Set response content type
resp.setContentType("text/html");
// print the output
PrintWriter out = resp.getWriter();
out.write("<html><head><title>Servlet 3 Exception Handling example</title></head><body>");
if (statusCode != 500){
out.write("<h3>Servlet 3 Exception Handling</h3>");
out.write("<strong>Status Code</strong>:" + statusCode + "<br>");
out.write("<strong>Requested URI</strong>:" + requestUri);
} else {
out.write("<h3>Servlet 3 Exception Handling</h3>");
out.write("<ul><li>Servlet Name:" + servletName + "</li>");
out.write("<li>Exception Name:" + exception.getClass().getName() + "</li>");
out.write("<li>Requested URI:" + requestUri + "</li>");
out.write("<li>Exception Message:" + exception.getMessage() + "</li>");
out.write("</ul>");
}
out.write("</body></html>");
}
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
Servlet 3 web.xml Configuration
We can define our servlet 3 exception handling servlet in the servlet descriptor.
- Global: If none of the other error-code or exception-type definitions maps then this global location element will map to the exception handler.
- Error Code: If a specific error occurres that maps to an error code then the error page will be forwarded to the registred servlet 3 exception handling error servlet.
- Exception Type: This will maps the thrown exception with a exception handler.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<!-- define global error handler -->
<error-page>
<location>/error-handler</location>
</error-page>
<!-- define specific error code handler -->
<error-page>
<error-code>404</error-code>
<location>/error-handler</location>
</error-page>
<!-- define specific error exception handler -->
<error-page>
<exception-type>java.lang.UnsupportedOperationException</exception-type>
<location>/error-handler</location>
</error-page>
</web-app>
Demo
404 – Page Not Found
URL: http://localhost:8080/exception-handling/page-does-not-exist
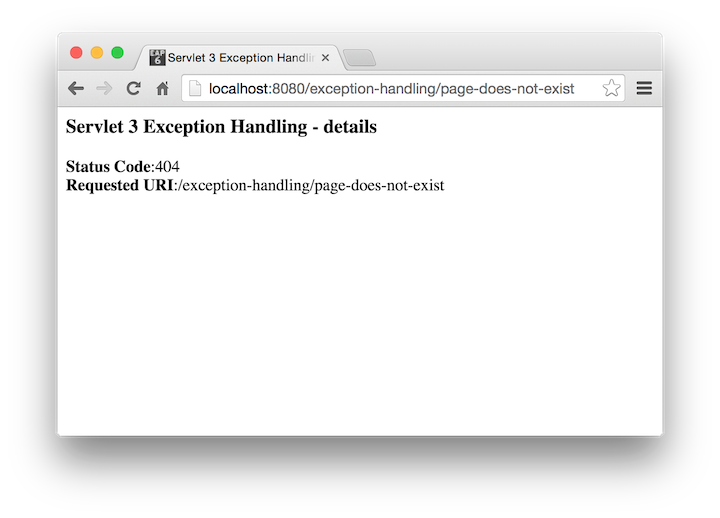
500 – Servlet That Generates an Error
URL: http://localhost:8080/exception-handling/error
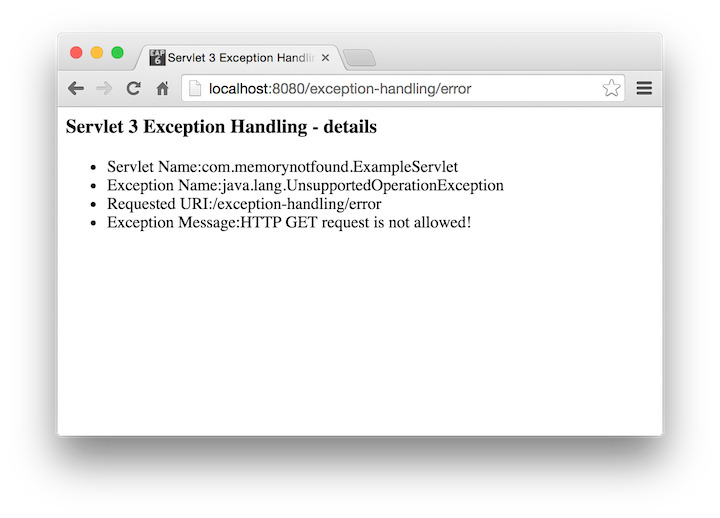