Servlet 3 initialisation parameter annotation @WebInitParam
We will show how you can use the servlet 3 initialisation parameter annotation @WebInitParam
to inject initialisation parameters in our servlet. We can configure our servlet entirely with annotations which means no more web.xml which is awesome.
Project structure
+--src
| +--main
| +--java
| +--com
| +--memorynotfound
| |--ExampleServlet.java
| |--resources
| |--webapp
pom.xml
Maven Dependency
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
Servlet 3 initialisation parameter annotation
The @WebInitParam
annotation is used to specify init parameters for a servlet or filter. The servlet 3 initialisation parameter takes a required name and value. You can add a description but this is rather informative. In the initialisation method init()
we can get our init parameter using the servletConfig.getInitParameter()
method.
package com.memorynotfound;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebInitParam;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet(
value = "/example",
initParams = {
@WebInitParam(name = "email", value = "[email protected]", description = "Email from webmaster"),
@WebInitParam(name = "phone", value = "xxxx/xx.xx.xx", description = "Phone webmaster")
},
description = "Servlet 3 initialisation parameter annotation example: @WebInitParam")
public class ExampleServlet extends HttpServlet{
private String email, phone;
@Override
public void init(ServletConfig config) throws ServletException {
email = config.getInitParameter("email");
phone = config.getInitParameter("phone");
}
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
resp.setContentType("text/html");
PrintWriter out = resp.getWriter();
out.write("<h2>Servlet 3 initialisation parameter annotation example: @WebInitParam</h2>");
out.write("<p><strong>E-mail: </strong>" + email + "</p>");
out.write("<p><strong>Phone: </strong>" + phone + "</p>");
}
}
Demo
URL: localhost:8080/init-param/example
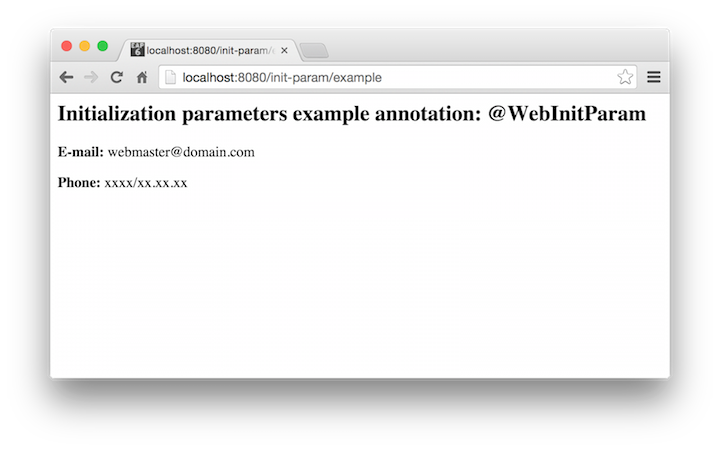